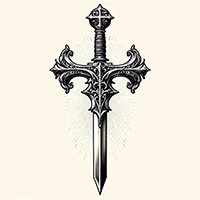
WE SHALL NEVER SURRENDER
What on Earth is PyAnySet_CheckExact()? đImagine youâre a club owner (a Python developer) and youâve got a VIP section (your code) that only certain people (data types) can enter. PyAnySet_CheckExact() is like your super-strict bouncer, making sure only the exact type of sets get into the club.
So, What Does It Do? đPyAnySet_CheckExact() checks if an object is exactly a set. Not a list that kinda looks like a set. Not a dictionary trying to sneak in with a fake ID.
What is PyAnySet_Check()? đImagine you have a magical spell that can tell you if an object is a set or not. Thatâs exactly what PyAnySet_Check() does! Itâs a built-in function in Pythonâs C API that checks whether a given object is a set (or something closely related).
How to Use PyAnySet_Check() đNow, unless youâre planning on dabbling in some Python-C hybrid code, you wonât be using PyAnySet_Check() directly in your everyday Python scripts.
What the Heck is PyAIter_Check()? đThink of PyAIter_Check() as the bouncer at a club. Its job? To make sure only the cool kids (asynchronous iterators) get in. Itâs a C API function in Python that checks if an object is an asynchronous iterator.
How to Use It? đOh, you donât actually use it in your day-to-day Python scripts. Itâs more like the secret sauce in the Python internals that keeps everything running smoothly.
What is Py_ssize_t PyGC_Collect(void)? đThe function Py_ssize_t PyGC_Collect(void) is part of Pythonâs C API, which means itâs used in the underlying C code that implements Python, rather than in everyday Python scripts. Its primary role is to trigger a garbage collection process, which identifies and cleans up memory that is no longer in use.
How is it Used? đIn Python, most of the time, you donât have to worry about memory management because the interpreter handles it for you.
What is PyCodec_StrictErrors? đPyCodec_StrictErrors is a predefined error handler in Python that deals with encoding and decoding issues strictly. When an error arises in the text conversion process, this handler raises a UnicodeDecodeError or UnicodeEncodeError, effectively stopping the process and signaling that something has gone wrong.
Why Use PyCodec_StrictErrors? đThink of encoding and decoding like translating languagesâerrors can lead to misunderstandings or even data corruption. Hereâs why PyCodec_StrictErrors could be your go-to:
What is PyCoro_CheckExact? đImagine yourself at a party, and in one corner of the room, thereâs a separate group chatting animatedly about coroutines. Now, PyCoro_CheckExact is like a super specific bouncer who can identify, with perfect accuracy, if someone belongs to this âcoroutine clan.â
In Python, coroutines are special functions that allow for asynchronous programming. They let you pause and resume execution, which is helpful when youâre dealing with tasks that might take some time, like network requests or file I/O operations.
What is PyNumberMethods.nb_true_divide? đYou can think of PyNumberMethods.nb_true_divide as a function that handles division for numeric types in Python. Imagine you have two numbers and you want to divide one by the other, much like splitting a pizza into equal slices. This function figures out how to make those slices perfectly.
Hereâs a quick explanation in technical terms: PyNumberMethods.nb_true_divide is a field in the PyNumberMethods structure, which is part of Pythonâs C-API.
What Is PyContext_Exit? đImagine youâre hosting a party at your house. When guests arrive, you greet them and ensure they have everything they need. Upon leaving, you bid them farewell and take the opportunity to tidy up the space they occupied. This sequence of actionsâensuring a smooth entry and a clean exitâis akin to what context management in Python aims to accomplish.
PyContext_Exit is an internal function in Python that ensures the cleanliness and orderliness of resources when exiting a context.
What is PyConfig.pathconfig_warnings? đImagine youâre cleaning your house. You have a checklist of things to tidy up, but sometimes you face unexpected issuesâlike finding out youâve misplaced the vacuum cleaner. In such cases, warnings can alert you about the missing items. PyConfig.pathconfig_warnings works similarly: it issues warnings related to the path configuration of your Python environment.
Technically, PyConfig.pathconfig_warnings is a field in the CPython configuration system (PyConfig), which is part of Pythonâs C-API.
What is PyImport_ImportModuleLevelObject? đIn simple terms, PyImport_ImportModuleLevelObject is a C API function in Python that is used to import modules. Think of it as a backstage crew member at a theater production. While you might not see it in action directly, itâs doing a lot of work behind the scenes to ensure everything runs smoothly on stage.
How is it Used? đConsider youâre writing a Python program and want to import a module, typically youâd use the usual import module_name syntax.
What is PyMem_RawFree? đImagine your computerâs memory is like a warehouse. When you store items (data) in the warehouse, you need to allocate space for them. Eventually, when these items are no longer needed, you should free up that space so it can be used for other things.
In Python, PyMem_RawFree is a function that helps manage this process of freeing up memory. It is part of the Python C API, which allows for Pythonâs integration with C code.
What is PyModuleDef.m_slots? đImagine building a custom toy robot. PyModuleDef.m_slots are like the different slots or compartments where you can install various featuresâlike wheels, sensors, or a cute little hat. In Python, a module often needs specific functionalities to work correctly, and these functionalities can be âslotted inâ using PyModuleDef.m_slots.
How is PyModuleDef.m_slots Used? đPyModuleDef.m_slots is part of the Python C API, used to define and extend modules. Itâs an array of PyModuleDef_Slot structures, which specify additional initialization options for a module.
What is PyNumberMethods.nb_index? đTo understand PyNumberMethods.nb_index, we must first explore the concept of magic methods (also known as dunder methods) in Python. These are special methods prefixed and suffixed with double underscores, such as __init__ or __str__. Theyâre designed to customize the behavior of objects within Python.
PyNumberMethods.nb_index corresponds to the __index__() method in Python. This method is used to define how an object should be converted to an integer, typically for operations that require integer-like behavior.
What is PyInterpreterState_Head? đLetâs imagine managing Pythonâs internal state as running an orchestra. Every instrument (component of Python) needs a conductor to keep things in harmony. In this analogy, PyInterpreterState_Head is like the conductorâs baton that initiates and coordinates the orchestraâs (or Pythonâs) performance.
In technical terms, PyInterpreterState_Head is a function in Pythonâs C API that returns the head of the linked list of all PyInterpreterState objects. A PyInterpreterState object contains information about one instance of the Python interpreter.
What the Duck is PyMapping_Length? đLetâs start with the basics: PyMapping_Length is a C-API function in Python that you can use to determine the length of an object that supports the Mapping Protocolâessentially dictionaries (but potentially other mapping types too). Think of it as a backstage pass to see how many pairings of keys and values are in your dictionary.
In the context of Python, this function is akin to the len() function.
What is PyGetSetDef.set? đTo put it simply, PyGetSetDef.set is a C-level function that defines setter methods in Pythonâs C API. If youâre scratching your head at âC APIâ, donât fret! This just means itâs used in the background when Python integrates with C or is dealing with low-level operations.
Why Should You Care? đWhile you usually deal with Pythonâs high-level, readable code, understanding setters and the C API helps you comprehend whatâs under the hood.
What is PyCallIter_Type? đImagine you have a magical friend who can read a book, and every time you ask them to read the next sentence, they follow your instruction precisely and give you just that one sentence. In the Python realm, PyCallIter_Type is somewhat like that friend. Itâs an internal object type designed to turn a callable (like a function) into an iterator.
An iterator, if you recall, is an object that contains a countable number of values that can be traversed, and it follows the iterator protocol: it has __iter__() and __next__() methods.
What is PyNumberMethods.nb_int? đImagine Python as a factory, and PyNumberMethods.nb_int as a specialized machine responsible for converting certain raw materials (objects) into the final product (integers). In technical terms, PyNumberMethods.nb_int is part of a structure in Pythonâs C API that lets you define how an object should be converted into an integer.
How is PyNumberMethods.nb_int Used? đSo, how do you use this powerful machine? Usually, you wonât interact with PyNumberMethods.nb_int directly unless youâre diving into extending Python with C or writing a Python C extension.
What Is PyDate_CheckExact? đImagine you have a freshly baked apple pie. Now, you need to determine if this pie is strictly an apple pie, not an apple and rhubarb pie, not an apple tartâjust a pure apple pie. In the Python C API world, PyDate_CheckExact is the function that ensures that your object is precisely a date object, not a derived class or a subclass.
In technical terms, PyDate_CheckExact checks if a given object is a date object without any subclassing shenanigans.
What is PyFloat_Pack8? đAt a high level, PyFloat_Pack8 is a function grounded in Pythonâs internal low-level C-API. Its primary job is to convert a floating-point number into an 8-byte sequence (hence, the â8â in PyFloat_Pack8). This packed format helps with low-level data manipulation, binary file operations, and networking where precision and format consistency are paramount.
Why should you care? đPicture this: Youâre an adventure-seeker rummaging through a treasure chest of ancient artifacts (similarly, Pythonâs vast function library), and you stumble upon this, PyFloat_Pack8.
What is Hashing? đThink of hashing as Pythonâs method of giving every object its own unique fingerprint. Just like how each individual has a unique set of fingerprints, each object in Python gets a unique hash value, thanks to the magic of hashing functions. These fingerprint-like values are used primarily to quickly compare dictionary keys during lookups.
The Role of PyHash_FuncDef.name đLetâs unravel PyHash_FuncDef.name bit by bit:
PyHash: This prefix indicates that weâre dealing with the hashing module in Python.
What is PyList_AsTuple? đPyList_AsTuple is a function in the Python/C API that converts a Python list into a tuple. If youâre not familiar with these terms yet, hereâs a quick refresher:
List: A mutable (changeable) ordered collection of elements. Think of it as a flexible, dynamic array. Tuple: An immutable (unchangeable) ordered collection of elements. Once you set its contents, you canât change them. Why Convert a List to a Tuple?
What is PyFunction_New? đIn Python, everything is an object, and functions are no exception. PyFunction_New is a foundational part of the Python C API that creates new function objects from code objects and a global dictionary. Think of it as the factory that assembles a car (the function) from its components (code objects and global dictionaries).
Why Should You Care About PyFunction_New? đYou might wonder why knowing about PyFunction_New is beneficial.
What is PyMem_RawCalloc? đJust as a carpenter needs a workbench to hammer out his masterpieces, Python needs memory management functions to allocate, use, and free memory effectively. Think of PyMem_RawCalloc as one of those sturdy workbenches designed to help Python handle memory allocation, particularly in the C environment where Pythonâs core is implemented.
In essence, PyMem_RawCalloc is a function provided by Pythonâs C API for low-level memory allocation. The keyword here is ârawâ, which indicates that it deals directly with raw memory allocation, bypassing Pythonâs built-in memory management.
What is PyModule_FromDefAndSpec? đImagine if you were to bake a cake. You need a recipe (definition) and ingredients (specification). These two together help you create a delicious cake. Similarly, in Pythonâs C-API, PyModule_FromDefAndSpec plays the culinary role of combining a module definition (PyModuleDef) with a module specification (PyModule_Spec) to bake the final module object.
How is PyModule_FromDefAndSpec Used? đWhen youâre extending Python with C (yes, you can supercharge Python with C), you sometimes need to create modules dynamically.
What is PyFunction_Check? đImagine youâre Sherlock Holmes, but instead of solving crimes, youâre solving issues in your Python code. Now, think of PyFunction_Check as your magnifying glass. Itâs a tool that helps you inspect and determine whether a given object in Python is a function or not.
In more technical terms, PyFunction_Check is a function in Pythonâs C API that checks if an object is a function.
Why Would You Use PyFunction_Check?
What is PyDict_Next? đBefore we explore PyDict_Next, letâs talk about dictionaries in Python. Think of a dictionary as a magical book where each word (key) is linked to its definition (value). Youâve probably used these magical books countless times by nowâfor example, to store user data or even keep track of inventory.
So Why PyDict_Next? đIn high-level Python, you iterate through dictionaries with a simple for loop. But under the hood, things get intricate.
What is PyFrozenSet_New? đThink of PyFrozenSet_New as a magic spell that conjures an immutable set. In simpler terms, it creates a set whose elements cannot be changed after creation. This can be immensely useful in scenarios where you need a reliable, unchangeable group of items.
Why Do We Use PyFrozenSet_New? đImagine youâre a museum curator. You have a list of rare artifacts that should never, ever be altered or swapped. The PyFrozenSet_New function is like the glass display cases for these artifactsâit ensures your list of precious items stays intact and untouched.
What is PyImport_ImportModule? đIn simple terms, PyImport_ImportModule is a function in Pythonâs C API that allows you to import modules. If youâve used Python before, youâre probably familiar with the import statement, like this one:
import math PyImport_ImportModule is like the backstage pass to this import mechanism. It does the heavy lifting behind the scenes, especially when youâre writing Python code that interacts directly with C or C++ through the Python/C API.
What is PyErr_FormatV? đSimply put, PyErr_FormatV is a function used in Pythonâs C API to set an exception with a formatted error message. Itâs the C equivalent of the Python raise statement that you might already be familiar with. If something goes wrong in your C code, youâll use PyErr_FormatV to communicate the error back to Python in a human-readable way.
Why is It Important? đImagine youâre trying to assemble a piece of IKEA furniture, and instead of a helpful picture, you receive a note saying, âSomething went wrong.