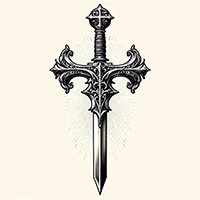
WE SHALL NEVER SURRENDER
What is PyNumberMethods.nb_inplace_or? đImagine youâre building a custom pizza. Normally, youâd make changes to your order by scratching out ingredients and writing new ones. But what if you had a magical pen that lets you update your order instantly without scratching anything out? Thatâs what nb_inplace_or does for objects in Python â it modifies them in place.
PyNumberMethods is a struct that contains various function pointers used to implement the numerical operations on Python objects.
What Exactly is PyBytes_FromString? đThink of PyBytes_FromString like a skilled artisan. This function takes raw materialsâin this case, a C-style string (essentially an array of characters ending in a null character \0)âand crafts it into a beautiful, usable Python bytes object.
Why Would You Need It? đImagine youâre a gourmet chef, and C is your kitchen. Youâve got all your ingredients in the form of C strings, but your customers (Python programs) want a delectable bytes object.
What is PyGen_Type? đPicture Python as a large, elaborate theater. Within this theater, PyGen_Type holds a very special roleâit is the underlying type for Python generators. Think of it as a specific type of actor that specializes in performing generator functions. Just like how every theater actor has a specific role to play, every generator in Python is an instance of PyGen_Type.
Generators, in the simplest terms, are a way to iterate over data without having to store it all in memory at once.
What is PyInterpreterState_Delete? đPyInterpreterState_Delete is a function at the core of Pythonâs CPython implementation, part of its C API. Its main job is to safely and efficiently clean up an interpreter state when itâs no longer needed. If Python interpreters were waiters at a restaurant, then PyInterpreterState_Delete would be the one making sure the table is cleaned and reset for the next guests.
How is it Used? đTypically, you wonât see PyInterpreterState_Delete in your everyday Python script.
Breaking Down PyLong_AsUnsignedLongLong đSo, what is PyLong_AsUnsignedLongLong? To put it simply, itâs a function within the Python C API that converts a Python object (typically an integer) to an unsigned long long type in C. Think of it as a specialized translator for taking something from Pythonâs party and introducing it to Câs party, making sure it fits in well.
The Key Players: Python and C đFirst, letâs recall the two languages at our party - Python and C.
What Is PyDateTime_CheckExact? đSimply put, PyDateTime_CheckExact is a function used in Pythonâs C API, particularly if you are diving into extending or embedding Python with C or C++. It checks whether a given object is an exact instance of a datetime object. So, in simpler terms: itâs like a bouncer at an exclusive club that verifies whether someone is a bona fide member (a datetime object) and not just some duck trying to walk the walk.
What is PyModuleDef.m_base? đImagine Pythonâs C API as an extensive toolkit â within this toolkit, PyModuleDef is a blueprint for defining new modules. Modules, in Python, are akin to file folders containing scripts that you can import and use in your programs.
Hereâs where PyModuleDef.m_base plays a critical role. Think of it as the foundational bedrock of this blueprint. Itâs the base structure that ensures everything is in place for Python to understand and interact with your module correctly.
The Function: What is PyConfig_SetBytesString? đAt its core, PyConfig_SetBytesString is a function in the Python C API. If Python were a theater, this function would be one of the crew members working behind the curtains, ensuring the show runs smoothly. Itâs designed to set configuration options in Pythonâs runtime environment.
Think of PyConfig_SetBytesString as a specialized messenger tasked with delivering specific configuration settings to Python, allowing for precise adjustments in the interpreterâs behavior.
What is PyImport_ExecCodeModuleObject? đImagine youâre a wizard and you have a scroll filled with magical spells (code). Now, you need a way to recite these spells within your enchanted book (module) so they come to life. The function PyImport_ExecCodeModuleObject is like a magical incantation in Python that allows you to insert, compile, and execute code dynamically within a module.
In simpler terms, PyImport_ExecCodeModuleObject is a function that executes Python code within the namespace of a given module object.
What is PyCode_Addr2Location? đTo put it simply, PyCode_Addr2Location is a function from the Python C API. It translates an address (or bytecode offset) within a code object to a line number. Imagine it as a stage director who can tell you exactly which line of the script a particular scene (or bytecode address) corresponds to.
How is PyCode_Addr2Location Used? đThis function might not be something you use directly in everyday scripting.
What is PyDelta_FromDSU? đImagine youâve just baked a cake. Youâd like to know how much time you spent mixing, baking, and frosting it. Now, think of PyDelta_FromDSU as the recipe card that transforms your raw measurements (hours, minutes, and seconds) into a single, delicious time object you can use in your Python code.
In technical terms, PyDelta_FromDSU is a function in Pythonâs C API that creates a timedelta object. This timedelta object represents the difference between two dates or times.
What is PyErr_SetFromErrnoWithFilename? đIn simple terms, PyErr_SetFromErrnoWithFilename is a function used in Pythonâs C API that sets an exception based on the most recent error number (errno) and attaches an optional filename to the exception message. Think of it as the Python equivalent of having a magic wand that can raise a specific error along with pointers to a file for added context.
Unboxing the Functionality đThe primary purpose of PyErr_SetFromErrnoWithFilename is error reporting.
What is PyEval_EvalCode? đIn the Python universe, PyEval_EvalCode is akin to a sophisticated translator at the United Nations. Just as a translator converts spoken words from one language to another, PyEval_EvalCode takes source code objects (essentially precompiled Python code) and breathes life into them, allowing your program to actually âdoâ something with that code.
In more technical terms, PyEval_EvalCode evaluates Python code objects in a given execution environment. Itâs the function that sits at the very core of Pythonâs evaluation loop, turning bytecode into executed actions.
What is PyEval_EvalFrame? đIn the realm of Python, PyEval_EvalFrame is like the conductor of an orchestra, ensuring that each piece of code follows the correct sequence and harmonizes perfectly. Itâs a core function in the CPython interpreter that executes the bytecode for Python functions. This might sound complex, so letâs unpack it layer by layer.
How Is It Used? đFor most Python users, PyEval_EvalFrame operates behind the scenesâthey donât interact with it directly.
What is PyFrame_GetLasti? đTo understand PyFrame_GetLasti, we first need to take a quick detour into Pythonâs internal workings. When Python code runs, it executes inside a âframe,â which you can think of as a snapshot containing all the information Python needs to execute your code. Every frame keeps track of variables, the control flow, and the state of the current execution.
PyFrame_GetLasti, in essence, is a function that retrieves the index of the last executed bytecode instruction in a specific frame.
What Exactly is PyFunction_AddWatcher? đEssentially, PyFunction_AddWatcher is a function that lets you set up a âwatcherâ â a callback function that gets notified whenever any other function is called or returns. This can be incredibly useful for debugging, profiling, or simply keeping track of what your program is doing.
How to Use PyFunction_AddWatcher đTo get started, youâll need to understand the basic usage pattern. Hereâs a step-by-step guide:
Import the Required Module: This functionality is part of Pythonâs internal API, so itâs not something you use directly in Python scripts.
What is PyLong_AsSsize_t? đImagine for a moment youâve got a giant robot (your Python program) and a small human interpreter (your C code) working together. They need to communicate effectively. PyLong_AsSsize_t is like the translator that helps the robot translate its long words into shorter, more manageable words that the little human can understand.
In more technical terms, PyLong_AsSsize_t is a function in Pythonâs C API. It is used to convert a PyObject (specifically, a Python integer object) into a value of type Py_ssize_t.
What is PyCodec_Decode? đImagine youâre deciphering a secret message. Instead of cryptic symbols, you have strings of bytes that need to be transformed into readable text. PyCodec_Decode is your decoding tool for this job.
In technical terms, PyCodec_Decode is a function used within Pythonâs C API to decode byte sequences (like bytes or bytearrays) into strings using a specified character encoding. In simpler terms, itâs the magic wand that converts encoded data into human-readable formats.
What is PyInstanceMethod_New? đIn a nutshell, PyInstanceMethod_New is a PyObject representing a method bound to an instance. Imagine you have a magical key that only works with a specific lock. PyInstanceMethod_New creates that key for you. This function is part of Pythonâs C-API, which means itâs typically used in extending or embedding Python with C or C++.
Why Should You Care? đUnderstanding PyInstanceMethod_New can be quite handy if you ever dive into creating C extensions or embedding Python in other applications.
What is PyGen_NewWithQualName? đThe function PyGen_NewWithQualName is part of Pythonâs C API, which is a collection of functions used to interact with Python objects at a low level. Concisely, this function is responsible for creating a new generator object.
How is PyGen_NewWithQualName Used? đLetâs start with a brief overview: Generator objects in Python are essentially âpausedâ functions that yield values one at a time. You may have used generators in Python with the yield statement, but PyGen_NewWithQualName is a bit more technical, being a part of the underlying machinery.
What is PyCodec_Encode? đImagine you have a super-secret recipe written in your native language, and you wish to share it with a friend who only speaks Binary Code (the language of computers). Hereâs where encoding comes in handy. PyCodec_Encode is your translator, converting strings in Python into a specific encoding that computers or other applications can understand.
In a more technical sense, PyCodec_Encode is a function within Python that applies a specified encoding to a given string or bytes-like object.
What is PyMarshal_ReadObjectFromFile? đImagine you have a magic box (file) where you can store anything you like (Python objects) by shrinking them down to a specialized format. Now, this magic box can be opened anytime to retrieve the objects back. PyMarshal_ReadObjectFromFile is like the magical spell used to pull an object out of this box, restoring it to its original form.
In technical terms, PyMarshal_ReadObjectFromFile is a C-level function in Python, primarily used to read a Marshalled object from a file.
What is PyByteArray_GET_SIZE? đIn Python, bytearrays are mutable sequences of bytes. They are essentially like lists, but each element is a byte. The PyByteArray_GET_SIZE function is a C API utility provided by Python that returns the size of a bytearray object. The size, in this context, refers to the number of bytes in the bytearray.
How is PyByteArray_GET_SIZE Used? đTo properly use PyByteArray_GET_SIZE, you need to be working with Pythonâs C API, usually when youâre writing a Python extension in C.
Whatâs the Deal with PyBytes_CheckExact? đImagine Pythonâs data structures as characters at a party. You have strings, lists, integers, andâa lesser-known guestâbytes. Now, these bytes are a rather unique bunch. They donât mingle freely like strings; they are more like the hardcore data types used for binary data, which your computer can crunch effortlessly.
PyBytes_CheckExact is our bouncer at this party. Its job is to strictly check if a particular guest (Python object) is specifically a byte object and not some imposter that just looks like one.
What is PyBytes_FromStringAndSize? đIn Python, itâs common to manipulate byte sequences, especially when working with file I/O, network communication, or binary data processing. The function PyBytes_FromStringAndSize is a utility to create a new bytes object from a C string and a size. Essentially, itâs like a factory method that crafts byte objects with an exact number of bytes you specify.
How is it Used? đTo use PyBytes_FromStringAndSize, you need to write some C/C++ code that interacts with Python.
What is PyBytes_Type? đImagine you have a box. Not just any box, but a very special one that ONLY holds pieces of string. But waitâthese pieces of string arenât your average yarn. They are encoded in bytes, the fundamental units of data in computing. Voila, thatâs essentially what PyBytes_Type is in the Python world!
More formally, PyBytes_Type is an internal type object in Python that represents byte strings (bytes objects). Itâs the blueprint Python follows whenever you create a bytes object.
What is PyCell_Type? đImagine a bookstore where certain books can only reference other specific books. A PyCell_Type is somewhat like a specialized bookcase in this store tailored to hold these references securely. In more technical terms, PyCell_Type is a special object in Python that wraps a reference to another object, maintaining that reference throughout the runtime.
A Simple Definition đPyCell_Type is a built-in type in Python, used internally to hold references to variables.
What is PyCode_AddWatcher? đImagine youâre watching a play. The actors on stage give you the visual story, but behind the scenes, thereâs a flurry of activity â directors, stagehands, and technicians ensure everything runs smoothly. PyCode_AddWatcher is akin to a backstage director, helping to monitor and manipulate Python bytecode execution without stepping into the spotlight.
Why Use PyCode_AddWatcher? đIn Python, bytecode is the set of instructions that the Python interpreter executes.
What is PyCode_GetCellvars? đIn the world of Python, functions can be both local and nested. When you have a nested function, variables from the outer function can be used inside the inner function. This is where PyCode_GetCellvars comes into play. Essentially, it retrieves the cell variables from a code object. These cell variables are the variables that are used in inner functions but are defined in outer functions.
Imagine you have a workbench (the outer function) with a toolbox (the closure) that you want to pass down to your apprentice (the inner function).
What is PyCodec_BackslashReplaceErrors? đIn plain English, PyCodec_BackslashReplaceErrors is a built-in error handler in Python used for dealing with encoding and decoding issues. Essentially, itâs like a bouncer at a club who politely handles characters that donât quite fit in, providing a fallback strategy to avoid crashes in your code.
How Does It Work? đHereâs the technical bit: PyCodec_BackslashReplaceErrors replaces any unencodable character with a backslashed escape sequence. Think of it as a translator trying to make sense of foreign words by jotting down its phonetic pronunciation instead of the actual word.