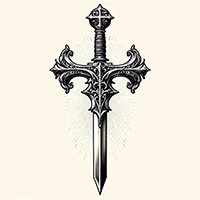
WE SHALL NEVER SURRENDER
What is PyModule_CheckExact? đIn the realm of Python, modules are like toolkits. A module is a file containing Python definitions and statements, and itâs one of the primary ways we organize and reuse code. But how do we check if a particular object is exactly a module, and not anything more general or derived? Enter PyModule_CheckExact.
PyModule_CheckExact is a function in Pythonâs C API used to verify whether a given object is a module created by Pythonâs default module implementation (PyModule_Type).
What is PyModule_FromDefAndSpec2? đTo understand PyModule_FromDefAndSpec2, letâs start with the basics. In Python, a module is a file that contains Python definitions and statements. A module can define functions, classes, and variables, and it can also include runnable code.
The PyModule_FromDefAndSpec2 function is part of Pythonâs C API â the part of Python that allows you to write modules in C (so they can run faster or interface with C libraries).
What is PyModule_GetDef? đPyModule_GetDef is a function used in the world of Pythonâs C API. While it may sound intimidating, letâs break it down step-by-step. In essence, it is a way to get the module definition (PyModuleDef) of a given module object (PyObject).
Imagine PyModule_GetDef as a backstage pass at a rock concert. You get access to see the âblueprintâ of the moduleâwhere all the magic was designed. Itâs like peeking behind the curtain to understand how a Python module is structured and behaves.
What is PyModule_GetFilenameObject? đFirst things first: PyModule_GetFilenameObject is a function in Pythonâs C-API. The primary purpose of this function is to retrieve the filename associated with a Python module. Think of it as a backstage pass that lets you peek at where a specific module resides on your filesystem.
The Basics: What it Does and Why it Matters đImagine you have a library book. Knowing the title (the module name) is nice, but sometimes you need to know where itâs located on the shelf (the filename or path).
What is PyModule_GetNameObject? đIn simple terms, PyModule_GetNameObject is a C API function used in Python to fetch the name of a Python module as a Python string object. Itâs part of the Python C API, a powerful tool that lets you interact with Python objects and functions at a lower, more granular level than pure Python code.
How is PyModule_GetNameObject Used? đImagine youâre at a library and you want to know the title of a book without opening the cover.
What is PyModule_NewObject? đImagine Python as a bustling city. Each module is like a building where a specific task or set of tasks is performed. PyModule_NewObject is the architect that designs and constructs these buildings. In simpler terms, it is a function in the Python C API used to create a new module object.
How is PyModule_NewObject Used? đWhile as a beginner, you might rarely need to use this function directly; understanding how it works can illuminate some of Pythonâs inner workings and deepen your appreciation for its magic.
What is PyModule_SetDocString? đImagine if every book you picked up had no blurbs, summaries, or intros. Youâd be left guessing what the content is about until you read through the entire thing. Tedious, right? PyModule_SetDocString is like that friendly blurb on the back of the book cover. It gives users a sneak peek into the moduleâs purpose and functionality.
In more technical terms, PyModule_SetDocString is a C API function in Python that sets the documentation string (docstring) for a module.
What is PyModule_Type? đImagine youâre at a library. Each section in the library categorizes various books on specific topics. Similarly, in Python, PyModule_Type is like a category in the languageâs vast library. Itâs a predefined structure in C that defines Python modulesâ type objects, playing a crucial role in Pythonâs C-API.
To put it another way, when Python scripts import a module (i.e., the files containing reusable code), the PyModule_Type object type ensures the module behaves like it should â a reusable, hierarchical bundle of code.
What is PyModuleDef_Init? đAt its core, PyModuleDef_Init is a C function that initializes a Python module. When you create a new module in C, especially if youâre writing Python extensions, PyModuleDef_Init is your starting point. Think of it as setting up the foundation for a buildingâjust as every building needs a reliable base, every module needs a solid initiation process.
How is PyModuleDef_Init Used? đUsing PyModuleDef_Init involves a few key steps.
What is PyModuleDef_Slot.slot? đIn laymanâs terms, PyModuleDef_Slot.slot is an attribute within the PyModuleDef_Slot structure, which itself is part of Pythonâs C API. This API allows developers to write C code that interacts with Python code, opening up a world of performance improvements and custom functionalities.
The PyModuleDef_Slot Structure đHereâs a simplified look at the structure for context:
typedef struct { int slot; // This is where PyModuleDef_Slot.slot comes in void *value; // This allows for additional data/configuration } PyModuleDef_Slot; slot: this field specifies the type of module initialization or function the slot is intended for.
What Is PyModuleDef_Slot? đBefore diving into what PyModuleDef_Slot.value is, we need to understand what PyModuleDef_Slot itself is. In Pythonâs C extension API (also known as CPython), a PyModuleDef_Slot is a structure that helps you define additional behaviors for Python modules implemented in C. This lets you go beyond the basic functionalities and add custom features to your Python modules.
Think of a PyModuleDef as a blueprint for building a house (your Python module).
What is PyModuleDef.m_clear? đIn Python, a module is like a blueprint for various functionalities that you can import and use in your programs. Every module has its internal state and objects. Over time, especially with prolonged usage, the moduleâs internals can accumulate unused or obsolete data. This is where PyModuleDef.m_clear steps inâit helps clear out these unnecessary remnants, keeping the moduleâs state manageable and reducing memory footprint.
How is PyModuleDef.m_clear Used?
What is PyModuleDef.m_doc? đIn simple terms, PyModuleDef.m_doc is a field that holds the documentation string (also known as a docstring) for a Python module. This docstring serves as an official description of what the module does, providing essential information to anyone who uses your module. Picture it as the cover summary of a book, offering you a snapshot of what to expect inside.
Why is it Important? đ Clarity and Usability: Just like a well-written book summary can entice you to read a novel, a well-defined m_doc can make your module much easier to understand and use for others.
What is PyModuleDef.m_free? đPyModuleDef.m_free is a method in the Python C API, part of the PyModuleDef structure, which is responsible for defining a module in C. In simpler terms, when you create a Python module using C, youâre dealing with PyModuleDef. The m_free function pointer is there to clean up any resources that your module no longer needs when itâs being unloaded.
Anatomy of PyModuleDef đTo understand m_free, letâs take a quick look at the PyModuleDef structure:
What is PyModuleDef.m_methods? đImagine your Python module as a fancy restaurant. Each function in the module is like a dish on the menu. The PyModuleDef.m_methods is the menu itself, listing all the dishes (functions) that patrons (users) can order (call) from the restaurant (module).
Technically, PyModuleDef.m_methods is an array of PyMethodDef structures. Each PyMethodDef holds information about a single function: its name, its C implementation, its calling convention, and a docstring.
What is PyModuleDef.m_name? đWhen you are extending or embedding Python with C, PyModuleDef.m_name is the name attribute of the PyModuleDef structure. In simpler terms, itâs the way you tell Python, âHey, this is the name of the module Iâm defining in C.â Just like how your name identifies you among a sea of people, m_name is pivotal for Python to recognize and interact with your custom module.
Why is PyModuleDef.m_name Important?
What is PyModuleDef.m_size? đAt its core, PyModuleDef.m_size is a part of the PyModuleDef structure, which defines a new module in C. When you create a module in C and make it available to Python, PyModuleDef is your blueprint. Specifically, m_size sets the size of the moduleâs state in memory. In simple terms, it just tells Python how much space it should carve out for your moduleâs persistent state.
Think of m_size as the label on a jar that indicates how many cookies (or bytes) can be stored inside.
What is PyModuleDef.m_traverse? đIn the context of Python C extensions, PyModuleDef.m_traverse is part of the PyModuleDef structure, which defines a Python module implemented in C. This structure facilitates garbage collection for your C extension. If youâve ever wondered how Python handles memory management seamlessly, this is one of the mechanisms behind it.
Simply put, m_traverse is a function pointer that helps Pythonâs garbage collector identify all objects referenced by the module.
What is PyNumberMethods.nb_absolute? đIn the Python ecosystem, PyNumberMethods is a struct that defines numerous functions related to numerical operations, such as addition, subtraction, and comparison. Think of it as a toolkit that Python uses to perform arithmetic and other number-related tasks.
The nb_absolute function within this struct is specifically used to compute the absolute value of an object. The absolute value of a number is like its distance from zero on the number line, so it is always positive or zero.
What is PyNumberMethods.nb_add? đIn simple terms, PyNumberMethods.nb_add is a function pointer used to define how two objects should be added together within Pythonâs C extensions. Think of it as the behind-the-scenes magic that powers the + operator when youâre adding numbers, strings, or even custom objects.
Imagine you have two puzzle pieces. The + operator is like the interlocking mechanism that connects these pieces. PyNumberMethods.nb_add defines that mechanism at a lower level in Python.
What is PyNumberMethods.nb_floor_divide? đBefore we dive deeper, letâs break down the term:
PyNumberMethods: This is a structure in the Python C API that holds function pointers for numerical operations like addition, subtraction, multiplication, and division. nb_floor_divide: This specific function pointer within PyNumberMethods is responsible for the floor division operation, which you might know better as //. In simple terms, PyNumberMethods.nb_floor_divide is the mechanism behind the scenes that makes the // operator work in Python.
What is PyNumberMethods.nb_inplace_floor_divide? đIn the world of Python, objects and data types are the stars of the show. Behind the scenes, Python uses special operations to perform certain actions on objects. These operations are part of a larger structure known as PyNumberMethods.
nb_inplace_floor_divide is a field within PyNumberMethods specifically designed for in-place floor division. Floor division is like regular division, but it discards the fractional part and rounds down to the nearest whole number.
What is PyNumberMethods.nb_inplace_matrix_multiply? đIn simple terms, PyNumberMethods.nb_inplace_matrix_multiply is a pointer in Pythonâs C API. Yeah, I know, that sounds more technical than simple. Letâs break it down.
PyNumberMethods: This is a structure in Python that holds pointers to functions for numerical operations such as addition, subtraction, multiplication, etc. nb_inplace_matrix_multiply: This specific member of the PyNumberMethods structure points to the function responsible for performing in-place matrix multiplication. In other words, nb_inplace_matrix_multiply allows you to define how two matrices should be multiplied together and stored back into the left-hand operand.
What is PyNumberMethods.nb_inplace_multiply? đImagine you have a magical wand that not only doubles or triples objects instantly but does so in the blink of an eye. This is somewhat analogous to what nb_inplace_multiply does in Python. Itâs a function pointer within the PyNumberMethods structure that performs in-place multiplication on numbers.
In more concrete terms, nb_inplace_multiply modifies the original object directly rather than creating a new object, effectively performing the operation x *= y.
What is PyNumberMethods.nb_inplace_rshift? đIn simple terms, PyNumberMethods.nb_inplace_rshift is a method used to perform the in-place right-shift operation on a Python object. If youâre already familiar with bitwise operations, you can think of this as the in-place version of the right-shift operator (>>), but for Pythonâs internal data types.
Why Should You Care About In-Place Operations? đImagine you have a magic box (or a variable in programming terms) and you want to transform the content of this box without producing a clone of it elsewhere.
What is PyNumberMethods.nb_inplace_subtract? đPyNumberMethods.nb_inplace_subtract is part of Pythonâs C API and is a function pointer for handling the in-place subtraction operation -= for custom Python objects. Imagine youâre playing with Lego bricks (your Python objects), and you want to perform a specific operation like snapping two bricks apart (-=), but you still want to work with the existing brick (object) rather than creating a new one. Thatâs what PyNumberMethods.nb_inplace_subtract allows you to defineâhow to modify the Lego brick in place when performing subtraction.
What is PyNumberMethods.nb_inplace_true_divide? đAt its core, PyNumberMethods.nb_inplace_true_divide is a function pointer used in Pythonâs C API. If that sentence made your eyes glaze over, letâs back up a bit. In simpler terms, this is part of the way Python handles basic arithmetic operations like division, but with a twist: it works specifically for the operation a /= b, known as in-place true division.
True Division vs. In-Place True Division đFirst, remember that division in Python can be done in a few ways:
What is PyNumberMethods.nb_inplace_xor? đSimply put, PyNumberMethods.nb_inplace_xor is a special method in Python that handles the in-place exclusive or (XOR) operation for objects. If that sounds like gibberish, think of it this way: itâs like a backstage crew at a theater making sure the right spotlights are on when actors perform. It ensures that the ^= operation, a compound operator for XOR, works smoothly on Python objects.
Cracking the Code of XOR đBefore we dig deeper into nb_inplace_xor, letâs what XOR is.
What is PyNumberMethods.nb_multiply? đIn Python, the PyNumberMethods structure is part of Pythonâs C-API, which allows us to define how different types of objects behave during numerical operations. This structure encompasses various function pointers that map to numeric operations like addition, subtraction, and, you guessed it, multiplication.
The nb_multiply field specifically refers to the function that handles the multiplication (*) operation for custom objects. It is essentially the behind-the-scenes operator that dictates what should happen when two objects are multiplied using the * operator.
What is PyNumberMethods.nb_positive? đThink of PyNumberMethods.nb_positive as a backstage handler in a theatre production. While you see the stars and action on stage (your Python code), nb_positive pulls the strings in the background to make sure everything runs smoothly, especially when you use the unary positive operator (+).
In Python, this operator is invoked when you write something like +object. The PyNumberMethods structure provides a way to define what happens behind the scenes for numerical operations, and nb_positive is specifically responsible for the unary positive operation.