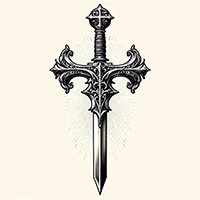
WE SHALL NEVER SURRENDER
What is PyCodec_IgnoreErrors? đFirst, letâs demystify this enigmatic name. PyCodec_IgnoreErrors is an error handling strategy in Python thatâs used when youâre working with text encoding and decoding. In essence, it tells Python to ignore any characters it canât encode or decode, acting like a doorman who simply refuses entry to any unrecognized guests.
Imagine youâve got an exclusive party (your string) and only characters with proper invitations (valid encoded characters) are allowed in.
Whatâs the Deal with PyCodec_KnownEncoding? đFirst, letâs start with the basics. Python, like any other programming language, must efficiently handle text. Text comes in various encodingsâways to map characters to numbers, essentially like different languages. PyCodec_KnownEncoding is a utility in Pythonâs codebase that helps manage these text encodings.
Why Do We Need PyCodec_KnownEncoding? đImagine trying to read a book written in a language you donât understand. Youâd need a dictionary or translation guide.
What is PyCodec_LookupError? đTo demystify PyCodec_LookupError, we need to break it down. In Python, the term âCodecâ stands for âCoder-Decoder,â which is essentially a mechanism to encode or decode data. A common use case for codecs is handling text encoding, like converting Unicode (which Python uses internally) to other encodings such as UTF-8 or ASCII.
A LookupError is a built-in Python exception raised when a specified key or index is not found in a sequence or mapping.
What is PyCodec_NameReplaceErrors? đAt its core, Pythonâs PyCodec_NameReplaceErrors is a function that deals with encoding and decoding errors. Picture it as a lifeguard patrolling the beach, ready to save your program from drowning in the sea of mysterious bytes and encoding mishaps.
When youâre working with text data that includes characters not covered by your chosen encoding, errors can ensue. PyCodec_NameReplaceErrors steps in to handle these errors gracefully, ensuring your program keeps running smoothly.
What Is PyCodec_RegisterError? đImagine that youâre in a foreign country, and youâve got a trusty tour guide who can help you navigate tricky situations. In Python, when youâre dealing with encodings and your program encounters an error, PyCodec_RegisterError acts like that tour guide who steps in to help handle the situation gracefully.
Simply put, PyCodec_RegisterError is a function that allows you to register a custom error handler for codec operations. A codec is a method for encoding or decoding a digital data stream or signal.
What is PyCodec_ReplaceErrors? đPyCodec_ReplaceErrors is a function in Python that deals with handling errors during encoding and decoding processes. When youâre converting data from one format to another (like text to bytes or vice versa), you might encounter characters that canât be directly translated. PyCodec_ReplaceErrors steps in to replace these problem characters with a placeholder character, typically the Unicode replacement character ďż˝ (U+FFFD).
Imagine youâre reading a book, and you come across an illegible word due to a smudge.
What is PyCodec_XMLCharRefReplaceErrors? đImagine youâre writing a novel and you discover some characters that donât belong thereâthey disrupt the flow of your wonderful story. In Python, manipulating strings (like Unicode characters) might occasionally result in unexpected or troublesome characters. Thatâs where PyCodec_XMLCharRefReplaceErrors steps inâlike an astute editor fixing those unwanted characters without altering the entire story.
Specifically, PyCodec_XMLCharRefReplaceErrors is an error handler provided by Pythonâs codec module. When encoding and decoding strings, it pinpoints invalid characters and replaces them with their corresponding XML character reference.
What is It? đPyCompilerFlags.cf_feature_version is a flag in Python that is part of the PyCompilerFlags structure. This particular flag is used to set the version of the Python syntax and features that the compiler should recognize. Think of it as telling the python compiler, âHey, pretend youâre a specific version of Python while youâre doing your job.â
Why Does It Matter? đImagine youâre a time traveler who can visit different eras, but you need to wear the proper attire for each period to blend in.
What Is PyCompilerFlags.cf_flags? đIn simple terms, PyCompilerFlags.cf_flags is a part of Pythonâs internal machinery that handles certain aspects of code compilation. When Python code is compiled, various flags can be set to tweak or modify how the code should be interpreted and executed. Think of these flags as little switches you can flip to enable or disable specific features.
Imagine youâre preparing a delicious stew. You have a recipe, but there are optional ingredients you can addâherbs for flavor, spices for heat, maybe even a splash of wine for a richer taste.
What is PyComplex_FromCComplex? đIn plain English, PyComplex_FromCComplex is a Python C API function that allows you to create a Python complex number object from a C complex number. Imagine youâre a linguist who speaks both English (Python) and Mandarin (C) â this function is akin to translating a concept from Mandarin to English seamlessly.
When and Why to Use It? đThis function is particularly useful when youâre working with C extensions or embedding C code in Python.
What is PyConfig_InitIsolatedConfig? đImagine your traditional Python environment as a bustling downtown with all sorts of interconnected streets, buildings, and pathways. Now, sometimes you need a quieter, more isolated spotâperhaps a private officeâto get some serious coding done without the downtown distractions.
PyConfig_InitIsolatedConfig is that private office. Itâs a way to initialize Python in an isolated configuration, meaning it allows you to run Python without interference from the outside environment or various system configurations that might mess things up.
What is PyConfig_Read? đIn essence, PyConfig_Read is a function provided by Pythonâs C API that reads the configuration from the PyConfig structure and initializes the Python runtime environment. If that sounds like a mouthful, letâs break it down.
Imagine your Python environment is a spaceship. Before launching, you need to make sure every system onboard is working correctlyâengines, life support, navigation. PyConfig_Read is like your pre-flight checklist. It examines the setup parameters, ensuring nothing critical is missing or misconfigured before the spaceship lifts off.
What is PyConfig_SetArgv? đImagine youâre directing a play. Your script (the Python code) dictates what the actors (the program) need to say and do, but someone still needs to handle the logistics, like getting the right props (command line arguments) on stage at the right time. Thatâs where PyConfig_SetArgv comes in. This function allows you to specify what the command line arguments should be, directly from your Python code.
How to Use PyConfig_SetArgv đBefore diving into the details, letâs set the scene with a quick example.
What is PyConfig_SetBytesArgv? đImagine your Python script as a cook in a kitchen, ready to whip up a delicious dish. But, before the cook can start, they need to know what ingredients (arguments) they have at their disposal. The PyConfig_SetBytesArgv function helps you provide these ingredients to your Python script, ensuring it runs with the right inputs.
In more technical terms, PyConfig_SetBytesArgv sets the command-line arguments (argv) of your Python program.
What is PyConfig_SetWideStringList? đLetâs start with a quick overview. PyConfig_SetWideStringList is a function in Pythonâs C API that allows developers to set a list of wide strings (wchar_t** in C) in a PyConfig structure. This might sound a bit complex initially, especially if youâre new to the C API or Unicode handling in Python, but stick with me.
Hereâs a simple analogy: Imagine you are programming a high-tech smart home, and PyConfig is like the central control unit where all settings and configurations are stored.
What is PyConfig.base_executable? đThink of PyConfig.base_executable as the foundation stone of your Python environment. Simply put, itâs a configuration setting in Pythonâs internal API that specifies the path to the Python interpreter originally used to start the current Python process. This is particularly relevant when youâre working with embedded Python applications or customizing Python for specialized use-cases.
Imagine you have a factory making widgets (your Python programs). The blueprint and machinery (the Python interpreter) used for creating these widgets are what PyConfig.
What is PyConfig.bytes_warning? đThink of Pythonâs configuration settings as a toolbox that allows you to fine-tune the behavior of your Python interpreter. Within this toolkit is a rather interesting parameter: PyConfig.bytes_warning. In simple terms, this parameter is used to control how the Python interpreter handles situations where byte strings and Unicode strings are implicitly mixed.
Why Does It Matter? đIn Python, strings are like mutable and immutable citizens of two different cities.
What is PyConfig.check_hash_pycs_mode? đLetâs break it down. Python, as you may know, is an interpreted language. This means that your Python code (those .py files) are eventually converted into bytecode (those .pyc files) that the Python interpreter can execute more efficiently. However, like any files, these .pyc files can be vulnerable to corruption, accidental modifications, or even malicious tampering. This is where PyConfig.check_hash_pycs_mode comes into play.
The PyConfig.check_hash_pycs_mode is a configuration option that determines how Python handles these compiled bytecode files.
What is PyConfig? đPyConfig is like the master plan for configuring various aspects of the Python interpreter. You change how Python behaves by tweaking the settings in PyConfig. Think of it as customizing a car to your specifications â you can decide on the color, interior design, and performance mods. One of the specs in this master plan is the exec_prefix.
The Exec Prefix in Detail đWhat Does PyConfig.exec_prefix Do? đIn simpler terms, PyConfig.
What Is PyConfig.filesystem_errors? đImagine the Python interpreter as a luxurious high-speed car. Now, this car needs a set of solid brakes to handle unexpected obstacles (errors) while racing down the information superhighway. PyConfig.filesystem_errors is akin to a brake system specifically designed to handle filesystem encoding errors.
In simpler terms, PyConfig.filesystem_errors dictates how Python handles errors that occur when file names are encoded or decoded. This becomes particularly important when dealing with filenames containing special characters or non-ASCII text, which can cause headaches due to different encoding standards.
What is PyConfig.import_time? đPyConfig.import_time is a configuration option in Python that allows developers to measure the time taken to import each module. Think of it like a stopwatch that tracks how long it takes for you to open the different chapters of a book. By enabling this, you can assess and optimize the efficiency of your imports, which can be a big help when trying to make your Python applications faster and more efficient.
What is PyConfig.install_signal_handlers? đIn simple terms, PyConfig.install_signal_handlers is a configuration flag in the Python runtime that determines whether signal handlers should be installed. In Python, signal handlers are used to intercept and handle signals sent to a process, like SIGINT (the signal sent when you press Ctrl+C in the terminal) or SIGTERM (a termination signal).
Think of PyConfig.install_signal_handlers as a light switch. When itâs âonâ (set to 1), Python will install the default signal handlers.
What is PyConfig.isolated? đThink of PyConfig.isolated as the plastic wrap you put on food in your kitchen to avoid contamination. It provides your Python script with an isolated environment, meaning your script runs without being affected by the external worldâenvironment variables, user site directories, or even the current working directory. Itâs like setting up a sandbox for your script to keep it pristine and predictable.
How to Use PyConfig.isolated? đTo use PyConfig.
What is PyConfig.legacy_windows_stdio? đPython has a special configuration option called PyConfig.legacy_windows_stdio. This configuration is part of the PyConfig structure, which controls various aspects of the Python runtime environment when your script is running. Specifically, legacy_windows_stdio comes into play to handle quirks in how input and output (I/O) work on Windows.
Think of Windows I/O like a quirky old printer. Sometimes, it formats things in ways that modern programs donât expect. The legacy_windows_stdio flag basically tells Python, âHey, keep things old-school for compatibilityâs sake.
What is PyConfig.module_search_paths_set? đImagine youâre an explorer, and Python is your map. Python needs to know where to search for modules youâre trying to import, kind of like how youâd need directions to find hidden treasures. PyConfig.module_search_paths_set is a flag that helps Python figure out exactly where to look.
In technical terms, PyConfig.module_search_paths_set is a part of the Python C-API configuration (PyConfig). When this flag is set, it indicates that the list of paths where Python should search for modules is explicitly defined by the user.
What is PyConfig.orig_argv? đIn Python, PyConfig.orig_argv is a feature used to track the original command-line arguments passed to a Python program before any processing or modification by the interpreter. Think of it as a time capsule, preserving a snapshot of the initial command line arguments that kickstarted your Python script.
How is PyConfig.orig_argv Used? đTo understand how this feature is used, letâs consider a simple example:
Suppose you have a Python script named example.
What is PyConfig.perf_profiling? đPyConfig.perf_profiling is a configuration setting in Python that toggles performance profiling. Itâs part of the broader PyConfig structure, which contains numerous settings for configuring Python runtime behavior before initializing the Python interpreter.
How is PyConfig.perf_profiling Used? đSetting up PyConfig.perf_profiling is akin to setting a timer before you start baking; you want to measure how long the whole process will take. Hereâs how you can employ it:
Import Libraries: First, you need the PyConfig structure from Pythonâs internal modules.
What is PyConfig.platlibdir? đPicture Python as a well-organized library, with books scattered in different sections based on genres. Now, imagine PyConfig.platlibdir as the label on one of these sections, telling you where to find books related to âplatform-dependentâ libraries. These are the bits of Python that might change depending on whether youâre running your code on Windows, macOS, or Linux.
Why Should You Care? đWhen you write a Python program, it often relies on several little helpers called modules or libraries.
What is PyConfig.quiet? đImagine youâre sitting in a tranquil library, savoring the silence. Despite the bustling activities around, the environment remains noiseless because everyone operates in âquiet modeâ. Similarly, PyConfig.quiet is a setting in Pythonâs configuration that, when enabled, reduces the verbosity of Pythonâs output, much like that noise-reducing library experience.
How is PyConfig.quiet Used? đSetting up PyConfig.quiet đUsing PyConfig.quiet is straightforward. It involves working with the PyConfig configuration structure found in Pythonâs C-API.
What is PyConfig.run_command? đThink of PyConfig.run_command as a remote control that lets you execute snippets of Python code on the fly. Itâs a function provided by Pythonâs C API that enables you to run commands just as if you were typing them into a Python shell. This can be incredibly useful for embedding Python scripting capabilities within larger applications.
How to Use PyConfig.run_command đUsing PyConfig.run_command is akin to sending a text message to Python telling it what you want.