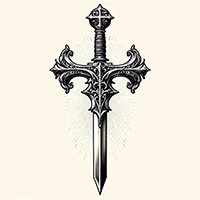
WE SHALL NEVER SURRENDER
What is PyComplex_AsCComplex? đIn a nutshell, PyComplex_AsCComplex is a C API function in Python that converts a Python complex object into a C-compatible complex struct. This function is particularly useful when youâre extending Python with C or C++ and need to handle complex numbers.
Think of Python and C as two friends speaking different languages. PyComplex_AsCComplex is like a skilled interpreter that helps them understand complex numbers fluently. It takes a Python complex object (like 3+4j) and translates it into a form that C can work with seamlessly.
What is PyComplex_CheckExact? đThink of Python as a bustling kitchen, with various ingredients (data types) and recipes (functions and methods) coming together to create delicious dishes (programs). In this kitchen, PyComplex_CheckExact is like the chefâs assistant, specifically trained to identify one particular ingredient: complex numbers.
In simpler terms, PyComplex_CheckExact is a function in the Python C API that checks whether a given Python object is a complex number (complex type) and ensures that it is the exact complex type rather than a subclass thereof.
What is PyConfig_InitPythonConfig? đThink of PyConfig_InitPythonConfig as the backstage crew at a theater performance. Theyâre seldom in the spotlight, but without them, the show wouldnât go on. In more technical terms, PyConfig_InitPythonConfig is a function in Pythonâs C API that initializes a PyConfig structure with default settings.
How is PyConfig_InitPythonConfig Used? đTo help you understand, letâs dive into an example. Imagine youâre setting up a new smartphone. Before you can start using it, you need to configure some initial settings, like language, Wi-Fi, and so on.
What is PyConfig.configure_c_stdio? đLetâs start with some basics. PyConfig is a structure in Python used for configuring the Python runtime environment before it starts. The configure_c_stdio attribute is one of the many settings you can tweak within this configuration.
Imagine configuring C stdio as setting up your own plumbing system in a house. Normally, you might not think about where the water pipes are or how they connectâthis is managed by some predefined system.
What is PyConfig.parse_argv? đImagine PyConfig.parse_argv as a helpful concierge at a hotel. This concierge takes your luggage (command-line arguments) and distributes it properly so you can effortlessly find what you need in your room. Essentially, PyConfig.parse_argv is responsible for processing command-line arguments in a way that Python can understand and use.
The Purpose of PyConfig.parse_argv đWhen running a Python script, you can pass additional information via command-line arguments. These arguments can alter the behavior of your script.
What is PyConfig.parser_debug? đThink of PyConfig.parser_debug as a pair of x-ray glasses for your Python code. When you put them on, you get a clear view of the skeletonâthe detailed process by which Python reads, analyzes, and converts your code into executable instructions.
The Technical Definition đPyConfig.parser_debug is a configuration option in Pythonâs C-API that allows developers to enable or disable parser debugging. Itâs part of the PyConfig structure used to initialize Python runtime with specific settings.
What is PyConfig.write_bytecode? đIn the simplest terms, PyConfig.write_bytecode is a configuration that controls whether Python should write .pyc files. These .pyc files are compiled Python files that the interpreter generates to streamline the execution of your code. Think of it as the fuel for a jet engineâwithout it, the engine canât start as efficiently.
In technical jargon, PyConfig.write_bytecode belongs to the PyConfig structure in Python, a flexible way to manage various settings that control the behavior of the Python interpreter.
The Magic Behind PyDate_FromTimestamp đImagine you have a massive, magical book that keeps a record of every single second since January 1, 1970 (yes, thatâs known as the Unix epoch). Now, what if you wanted to quickly pinpoint the exact date and time for a particular second in this colossal tome? This is essentially what PyDate_FromTimestamp does for you.
PyDate_FromTimestamp is a function in Pythonâs C API within the datetime module.
What is PyDateTime_DATE_GET_HOUR? đPyDateTime_DATE_GET_HOUR is a C API macro provided by Python that retrieves the hour component from a PyDateTime_DateTime object. Yes, it operates in the C programming layer of Python, which is why it might sound a bit intimidating at first. However, donât let the technical jargon scare youâthink of it as a specialized tool, like a screwdriver, that helps you focus on the exact part of the datetime object you are working with.
What is PyErr_SetFromWindowsErr? đImagine youâre embarking on a road trip. Every time your car encounters a problem (like a flat tire or an overheated engine), one of those little warning lights pops up on your dashboard, signaling precisely what went wrong. PyErr_SetFromWindowsErr is somewhat akin to those warning lights, but for Python programs running on Windows.
In simple terms, this function is used to set Python exceptions based on Windows system errors.
What is PyErr_SyntaxLocationEx? đThink of PyErr_SyntaxLocationEx as the GPS for your syntax errors. It does more than just tell you that youâve made a mistake; it pinpoints exactly where in your code the mistake occurred. When you use this function, Python is essentially saying, âHey, buddy, youâve got a problem on line 42, column 6.â
How is it Used? đLetâs step back for a second. PyErr_SyntaxLocationEx is part of the Python C API, which is a set of functions for developers who are diving deeper than the traditional Python script writing.
What is PyEval_EvalCodeEx? đLetâs start with the basics. PyEval_EvalCodeEx is a function in Pythonâs C API that evaluates a code object. Think of it as the conductor orchestrating an orchestra of code. It takes the composition (your Python code) and determines how it should be executed by the Python interpreter.
The Role in Pythonâs Ecosystem đImagine Python as a vast library filled with books (code). While you, as a user, might be flipping through pages (executing code) in a readable format, PyEval_EvalCodeEx is like the librarian who knows the behind-the-scenes mechanism, ensuring that each book is correctly indexed, placed, and available for reading.
What is PyEval_GetFuncName? đIn simple terms, PyEval_GetFuncName is a function in Pythonâs C API that retrieves the name of a function object. Itâs like asking, âHey Python, could you tell me the name of this function?â Python then dutifully responds with the functionâs name. Quite the courteous assistant, isnât it?
Why would you use PyEval_GetFuncName? đUnderstanding why you might need this function can help clarify its significance. Imagine a scenario where youâre debugging a complex Python application.
What Does PyEval_RestoreThread Do? đImagine Pythonâs GIL as a bouncer at a club who lets only one thread onto the dance floor (a.k.a., the Python interpreter) at a time. PyEval_RestoreThread is like giving the bouncer a VIP pass for a specific thread, allowing it to re-enter the dance floor.
In plainer terms, PyEval_RestoreThread is a C API function that reacquires the GIL for the thread that released it earlier. This function is typically used when embedding Python into C programs or dealing with C extensions to ensure that Pythonâs interpreter gets control back from the C code safely.
What the Heck is PyFloat_Type? đThink of PyFloat_Type as the behind-the-scenes operator managing all the float numbers in your Python applications. If Python were a theater, PyFloat_Type would be the stage manager ensuring all the floating-point numbers perform their roles correctly.
In more technical terms, PyFloat_Type is a type object in Pythonâs C API. It represents the floating-point number type and is an instance of PyTypeObject. This type object provides the blueprint for creating and managing float objects in your Python code.
Demystifying PyImport_ExecCodeModuleWithPathnames: How to Import Modules in Python with Custom Paths
What is PyImport_ExecCodeModuleWithPathnames? đImagine youâre organizing a library. Books are placed on specific shelves, and to find a book, you need to know its exact location. In this metaphor, PyImport_ExecCodeModuleWithPathnames is like a super-efficient librarian who can find and present a book from any shelf, even if itâs in an unmarked section or off-site storage.
In more technical terms, PyImport_ExecCodeModuleWithPathnames is a C API function in Python that allows you to execute the code of a module directly from a given path and then import that module into the current Python environment.
What is PyInterpreterConfig.check_multi_interp_extensions? đPyInterpreterConfig.check_multi_interp_extensions is a configuration setting in Pythonâs interpreter that affects how the interpreter handles multi-interpreter extensions. Think of it as a traffic light that controls the flow of certain features in a multi-lane highway (where each lane represents a Python interpreter). This setting determines whether specific restrictions are applied when youâre running multiple interpreters within the same process.
Why Should You Care? đIf youâre just dipping your toes into Python, you might not worry too much about this right now.
What on Earth is PyInterpreterState_Clear? đPicture Python as a bustling city. In this city, the âinterpreter stateâ is akin to the operational center governing all the activitiesâthe programâs state, running threads, loaded modules, and various other integral pieces. PyInterpreterState_Clear can be thought of as the sophisticated cleanup crew that comes in to wipe the slate clean, removing the current state in our Python city without knocking down the essential infrastructure.
What is PyInterpreterState_New? đIn the world of Python, an interpreter state manages the environment in which your Python code runs. Just as a conductor leads an orchestra, the interpreter state oversees the execution of the code, handling everything from memory management to module imports. When you call PyInterpreterState_New, youâre essentially summoning a brand-new conductor for a separate orchestra. This new state is isolated from others, meaning it wonât interfere with the main interpreter or any other states you may have created.
What is PyIter_Next? đPyIter_Next is a powerful function found in Pythonâs C API, a lower-level interface to the interpreter. It retrieves the next item from an iterator. Essentially, itâs the engine under the hood of the for loop and other iteration mechanisms. Itâs the worker bee, tirelessly fetching the next piece of data until the iterator is exhausted.
Think of an iterator as a conveyor belt at a sushi restaurant. You sit by the belt and keep grabbing plates of sushi (data).
What is PyList_Size? đSimply put, PyList_Size fetches the length of a list object in Python. In laymanâs terms, if a list were a train, PyList_Size would be the method to count the number of carriages (items) attached to it.
Py_ssize_t PyList_Size(PyObject *list) Here, PyObject is a pointer to a Python list, and Py_ssize_t is a type that represents the size of objects in Python. When you call PyList_Size, it returns the count of items in the list.
What is PyLong_FromUnsignedLong? đIn simple terms, PyLong_FromUnsignedLong is a function that converts Câs unsigned long integer types into Pythonâs int (formerly long in Python 2.x) objects. Think of it as a specialized bridge that lets large numerical cargo travel from the land of C to the realm of Python safely.
Why Do We Need It? đC and Python have different ways of handling integers. While Python integers can grow as large as the memory allows, C integers have a fixed size.
What Does PyLong_FromUnsignedLongLong Do? đIn Python, integers are represented using the int type. However, when working at the C level of Python, you sometimes need to create these Python integers from C data types. This is where PyLong_FromUnsignedLongLong() comes in.
Essentially, this function takes an unsigned long long value in C (which is a large, positive integer) and converts it into a Python integer object. Itâs like a bridge that allows a large C integer to safely cross over into the Python world.
What Exactly is PyMarshal_WriteLongToFile? đTo put it simply, PyMarshal_WriteLongToFile is a method provided by Pythonâs marshal module that lets you write a long integer to a file. Itâs akin to writing a note and saving it to your computer for future reference.
How Itâs Used đImagine you have a counter that keeps track of how many times your program has executed. You want to save that count to a file so that it persists between runs.
What is PyMethod_Type? đPicture PyMethod_Type as a magic spellbook in the world of Python. This spellbook helps define methods within classes, which are often called âbound methods.â In simpler terms, a bound method is a function that is associated with an instance of a class. When you call an instance method, the instance automatically gets passed as the first argumentâself.
How is PyMethod_Type Used? đTo illustrate, letâs create a class called Wizard and a method within this class.
What is PyMethodDef.ml_meth? đAt its core, PyMethodDef.ml_meth is a part of the C API for Python. Itâs a member of the PyMethodDef structure which is used to define methods for Python objects when youâre working with C code. Think of it as a translator between C functions and Python methods.
When you create a Python extension module in C, you need to tell Python which functions youâre exposing, and how to call them.
What Does PyModule_AddIntConstant Do? đPyModule_AddIntConstant is a C API function in Python that allows you to add an integer constant to a module. Think of it as pinning a sticky note with an important value onto your moduleâs bulletin board. This function enables C extensions to define constants in a moduleâs namespace.
How Is PyModule_AddIntConstant Used? đUsing PyModule_AddIntConstant is straightforward once youâre familiar with writing Python C extensions. Hereâs the basic syntax:
What is PyModule_Create? đIn simple terms, PyModule_Create is a C function provided by Pythonâs C API. Itâs used to create a new module object. Imagine youâre a wizard, and PyModule_Create is the spell you cast to conjure up a brand-new, ready-to-use module that Python can import and use just like any other native Python module.
How is PyModule_Create Used? đCreating a Python extension module involves a few essential steps. Letâs break them down:
What is PyModule_Create2? đLetâs imagine Python as a grand magical library, filled with countless spellbooks (modules) that hold powerful spells (functions). Now, what if you wanted to add your own spellbook to this library? Thatâs where PyModule_Create2 comes into play. This little wizard essentially helps you add custom-built modules to Python, enabling you to introduce new functions or functionalities.
Anatomy of PyModule_Create2 đDefinition and Input Parameters đThe PyModule_Create2 function is defined in C, since itâs often used in the realm of Pythonâs C API to create Python modules in C extensions.
What is PyModule_New? đImagine Python modules as toolboxes containing various tools (functions, classes, variables) to accomplish specific tasks. PyModule_New is like a factory that creates these toolboxes. If youâre building a house of software functionality, then PyModule_New helps you craft the specialized compartments where your invaluable tools reside.
How is PyModule_New Used? đUsing PyModule_New involves a bit of direct interfacing with Pythonâs very core, so buckle up. Hereâs the basic way to use it: