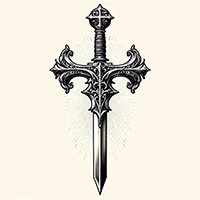
WE SHALL NEVER SURRENDER
What is PyModule_GetName? đIn a nutshell, PyModule_GetName is like a name tag for Python modules. Just as you wouldnât want to go through an entire party without knowing whom youâre talking to, you wouldnât want to dive deep into module internals without knowing the moduleâs name. PyModule_GetName provides a straightforward way to retrieve the name of a module when youâre working in C extensions.
How to Use PyModule_GetName đLetâs take a pit stop and see how to use this function.
What is PyBytes_FromObject? đThink of PyBytes_FromObject as a magical converter tool in Pythonâs C API. Its job is to take different data objects and turn them into immutable sequences of bytes. Whether youâre dealing with strings, byte arrays, or any other Python object that can sensibly be reduced to bytes, PyBytes_FromObject is your go-to function.
How is PyBytes_FromObject Used? đHereâs a simple picture: Imagine youâre a factory manager, and you need to standardize various raw materials into uniformly sized, packaged products.
What Is PyDateTime_TIME_GET_MINUTE? đIn simple terms, PyDateTime_TIME_GET_MINUTE is a macro from Pythonâs C API. It extracts the minute component from a datetime.time object. Imagine you have a detailed painting, and you want to know specifically the shade of blue used in the sky â this macro zooms in and tells you exactly that about your time object.
How to Use It đTo use PyDateTime_TIME_GET_MINUTE, youâll need to write some C code that interacts with Pythonâs C API.
What is PyMem_SetupDebugHooks? đImagine your Python program as a beautiful mansion. Every piece of memory that Python allocates is like a room in this mansion. Sometimes, unwanted guests (memory bugs) sneak into these rooms. PyMem_SetupDebugHooks acts like a meticulous security guard, patrolling every nook and cranny to catch these intruders.
In technical terms, PyMem_SetupDebugHooks installs debug hooks on Pythonâs memory allocation functions. This tool checks for memory issues like buffer overruns and uninitialized reads.
What Is PyList_GET_SIZE? đImagine you have a mystical treasure chest. That chest is your Python list, and PyList_GET_SIZE is like a magical device that tells you exactly how many items (or treasures) are in it. Sounds cool, right? So, what is PyList_GET_SIZE? Simply put, itâs a function used in C extensions to retrieve the number of items in a Python list.
Why Should You Care? đâWhy should I care about this esoteric function?
What is PyComplex_Check? đBefore diving into the deep end of the coding pool, letâs start with a little context. Python, like any other language, has functions that let you check the type of data youâre dealing with. PyComplex_Check is one of these functions specifically designed for complex numbers.
A complex number, in case you need a quick refresher, is a number composed of a real part and an imaginary part. It usually looks like this: a + bj, where a is the real part and b is the imaginary part.
What Is PyConfig.malloc_stats? đImagine your computerâs memory is a gigantic warehouse full of boxes where each box represents a unit of memory. Python is like the warehouse manager, efficiently keeping track of which boxes are being used and which ones are empty. PyConfig.malloc_stats is essentially a report that the warehouse manager can generate to give you an overview of how well the space is being utilized.
In technical terms, PyConfig.malloc_stats is a function that provides detailed statistics about memory allocations in Python.
What is PyDescr_NewWrapper? đImagine PyDescr_NewWrapper as a backstage technician in a theater production. Itâs not in the limelight, but it plays a crucial role in ensuring that the show runs smoothly. Specifically, PyDescr_NewWrapper is a low-level utility function used internally by Python to create new âwrapperâ descriptor objects. These wrapper descriptors act as intermediaries between the high-level Python code and the lower-level C implementation of Pythonâs built-in types and methods.
What Is PyInterpreterState_Get? đIn simple terms, PyInterpreterState_Get is a function in Pythonâs C-API that retrieves the current interpreter state. Think of the Python interpreter as the brain of your Python program. Just like how our brain keeps track of all our thoughts, senses, and actions, the interpreter keeps track of the entire state of your Python program.
Purpose of the Interpreter State đTo understand why accessing this state is crucial, letâs use a metaphor.
What is PyLong_FromDouble? đAt its core, PyLong_FromDouble is a function that allows you to convert a floating-point (double) value into a Python long integer (PyLongObject). Itâs not something youâll use in your everyday Python scripting, but if youâre delving into Pythonâs C extensions or working on performance-critical applications, understanding this function can be extremely useful.
Think of it as a wizardâs spell that transforms a slippery fish (your floating-point number) into a solid, unyielding rock (a long integer).
What is PyDelta_CheckExact? đImagine youâre a detective and youâve got a deceivingly simple question to answer: âIs this object exactly what I think it is?â In the world of Python, PyDelta_CheckExact is like your trustworthy detective tool to ask, âIs this object exactly a timedelta?â
In more technical terms, PyDelta_CheckExact is a macro in the CPython API (the default and most widely-used implementation of the Python programming language) used to determine if an object is precisely an instance of the timedelta type, not a subclass or anything else.
What is PyMarshal_ReadLastObjectFromFile? đIn Python, PyMarshal_ReadLastObjectFromFile is a function in the marshal module, which is primarily used for reading and writing Python objects to and from binary files. Think of it as a treasure hunter who, after exploring an ancient cave (or file, in our case), retrieves the last hidden artifact. This functionâs job is to read the last marshaled object from a file, simplifying the process of fetching data stored on disk.
What is PyFloat_GetMax? đIn simple terms, PyFloat_GetMax is a function that returns the maximum positive floating-point number that your Python environment can handle. Think of it as discovering the tallest mountain your Python program can scale when it comes to floating-point numbers.
Using PyFloat_GetMax đSo, how do you use this function, you ask? Well, hereâs where it gets interesting. Unlike many Python functions you might be familiar with, PyFloat_GetMax is not part of the standard Python library thatâs accessible directly in your code.
What is PyCapsule_GetName? đFirst off, letâs set the stage. Imagine you have a suitcase (PyCapsule) thatâs locked tight. This suitcase is an opaque object that holds a pointer to some C data â think of it like a treasure chest holding precious gems. The PyCapsule_GetName function is your luggage tag. It gives you a name or identifier for whatâs inside that suitcase.
In more technical terms, PyCapsule_GetName is a function used to retrieve the name associated with a particular PyCapsule object.
What Does PyCapsule_New Do? đImagine youâve got a precious nugget of dataâperhaps a C pointerâthat you want to safely pass around while ensuring it stays intact. PyCapsule_New is your treasure chest. It lets you wrap this nugget in a Python object, providing a safe, magical box that can be passed around, keeping its contents secure and unaltered.
How PyCapsule_New is Used đUsing PyCapsule_New is fairly straightforward. Its primary role is to create a new capsule object containing a pointer to your data, a name for identification, and optional destructor functions.
What Does PyErr_WriteUnraisable Do? đEver encountered a situation in Python where an error happens, but you canât or donât want to let it crash your program? Thatâs where PyErr_WriteUnraisable comes into play. Think of it as a safety net for exceptions. When an unforeseen error pops up, but you canât or donât want to handle it explicitly, this function is like putting your troubles in a âresults pendingâ drawer: you note it down and move on.
What is PyFunction_GetCode? đIn simple terms, PyFunction_GetCode is a C function that allows you to peek inside another Python functionâs internals to get its âcode objectâ. Just like a recipe card that describes how to bake a cake, a code object lists the exact steps Python follows to execute a function.
How is it Used? đImagine youâre a wizard with the ability to look into magical scrolls (Python functions). You want to understand a spell (function) better.
What Exactly is PyCapsule_IsValid? đPyCapsule_IsValid, a function provided by the Python C API, is like the bouncer at an exclusive club; it checks if the âcapsuleâ youâre holding has the right credentials to get in. To put it simply, it determines whether a given PyCapsule object is valid and can be safely used.
A Quick Dive into Capsules đCapsules in Python are like containers or capsules in real life: capable of holding something inside them.
What Exactly is PyErr_Occurred? đThink of PyErr_Occurred as a vigilant security guard. It stands at the gate, constantly checking if an error has just sneaked in. In more technical terms, PyErr_Occurred is a function used in the CPython API (the core implementation of Python) to detect whether an error has occurred during the execution of Python C extension code.
How to Use PyErr_Occurred đHereâs a simple example to demonstrate how you might encounter PyErr_Occurred in a Python C extension:
What Exactly is PyInterpreterState_GetID? đSimply put, PyInterpreterState_GetID is a function that returns a unique identifier for an interpreter state in Python. Python generally operates with one interpreter state per running process. However, when dealing with sub-interpreters or embedding Python in another application, various interpreter states come into play. Each of these states needs a unique identifierâthis is where PyInterpreterState_GetID comes in.
Why Should Beginners Care? đWhile working on Python projects, you may never need to directly interact with PyInterpreterState_GetID, but understanding what it does can help you appreciate Pythonâs flexibility and robustness.
What is PyBytes_FromFormatV? đImagine you are a chef, and you want to prepare a dish that combines various ingredients in a precise manner. PyBytes_FromFormatV is like your kitchen mixer which allows you to blend those ingredients (data) into a unified, formatted byte string. Essentially, PyBytes_FromFormatV is a lower-level C function used within Pythonâs C API to create byte objects from a formatted string, much like how you use printf in C.
What is PyCapsule_CheckExact? đImagine youâre at a party, and someone hands you a mysterious gift-wrapped box. Youâd naturally want to check whatâs inside, right? Well, PyCapsule_CheckExact is kind of like your gift-checking toolkit in the Python world. Itâs a function in Pythonâs C API that helps you determine whether a given object is a Capsule object.
In simpler terms, Capsules in Python are used to store C pointers within Python objects.
What is PyCapsule_Import? đImagine you have a magic box, and inside it, there are powerful tools that you can access without really knowing how they work. In Python, that magic box is represented by a capsuleâspecifically, a PyCapsule. PyCapsule_Import is the function you use to fetch the contents of this magical container and make it accessible in your code.
How Does PyCapsule_Import Work? đTo better understand this, letâs delve into a simple analogy.
What is PyCode_GetNumFree? đSimply put, PyCode_GetNumFree is a function in the Python C API that retrieves the number of free variables in a given code object. But what does this mean, exactly? To get a grip on this, letâs break it down.
Imagine youâre at a concert. The musicians (variables) are either on stage (local variables) or waiting in the wings (free variables that arenât defined in the current scope but are used).
What is PyCode_NewEmpty? đThink of PyCode_NewEmpty as a blueprint generator that creates an empty âcode objectâ in Python. A code object is a low-level representation of compiled source code. Itâs like the DNA of a function or a script, containing all the necessary information to execute the given code.
But what if you need an empty canvas? Thatâs where PyCode_NewEmpty comes in. It provides a mechanism to generate an empty code object without any actual executable content.
What is PyCodec_Encoder? đImagine you have a message written in English, and you need to send it to someone who only understands Morse code. Youâd need a translator to convert your English message into Morse code. PyCodec_Encoder serves a similar purpose in Python; it translates data from one format into another.
Specifically, PyCodec_Encoder is part of Pythonâs codec (coder-decoder) system. It can encode data into a specific format, such as bytes, strings, or other serializable types.
What is PyCodec_StreamWriter? đThink of PyCodec_StreamWriter as a scribe whoâs exceptionally good at writing messages in multiple languages. This scribe knows how to handle various scripts and encodings like UTF-8, ASCII, and so on. Essentially, PyCodec_StreamWriter is a class in Pythonâs codecs module that extends the basic stream writer capabilities to accommodate different encoding schemes. But Why Do We Need It? đIma
What is PyConfig_SetString? đThink of PyConfig_SetString as the utility knife of Pythonâs C APIâsmall, but exceptionally handy. Its sole purpose is to set specific string configuration options for Pythonâs runtime environment. Essentially, it allows you to manipulate the interior workings of Python before the interpreter even initializes.
How is PyConfig_SetString Used? đLetâs start with the basics. PyConfig_SetString is a function belonging to the configuration struct PyConfig, which houses various runtime settings for the Python interpreter.
What is PyConfig.base_exec_prefix? đThink of PyConfig.base_exec_prefix as a GPS coordinate in the Python landscape. Itâs a setting within Pythonâs initialization configuration (PyConfig) that points to the location where standard library executables are stored. This is crucial for Python environments to know where to look for certain executables when running scripts.
How is it used? đImagine youâre the captain of a ship, and you have a map that tells you where all the treasure is buried.
What is PyConfig.executable? đLetâs kick things off with an analogy. Imagine youâre on a quest and youâve got a magical map that guides you to treasure (Python scripts). Now, this map needs a starting point to navigate your journey. This starting point is what PyConfig.executable serves asâit tells Python where to begin its search. More technically, PyConfig.executable specifies the path of the Python interpreter executable, which is essentially the backbone of your Python environment.