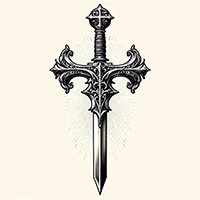
WE SHALL NEVER SURRENDER
What is PyNumberMethods.nb_rshift? đAt its core, PyNumberMethods.nb_rshift is a function used to perform the binary right shift operation in Python. This operation is commonly invoked using the >> operator. The PyNumberMethods.nb_rshift is a part of the PyNumberMethods structure that defines a set of function pointers for various numeric operations in Python.
The Essence of Right Shifting đBefore delving into the specifics of nb_rshift, letâs demystify the right shift operation with a simple metaphor.
What is PyCodec_StreamReader? đThink of PyCodec_StreamReader like a digital translator. When you travel to a foreign country, your translator helps you understand the local language by converting it into words you comprehend. Similarly, PyCodec_StreamReader reads data from a stream (like a file or network socket) and converts it into Pythonâs native representation, handling character encoding along the way.
How is PyCodec_StreamReader Used? đBefore we dive into the nitty-gritty, letâs set the stage with a basic example.
What is PyConfig.module_search_paths? đThink of Python as a treasure hunter, and PyConfig.module_search_paths as the map guiding it to treasure chests filled with precious modules. In more technical terms, this configuration parameter in Pythonâs initialization structure tells the interpreter where to look for modules. These directories can include standard library locations, site-packages from third-party libraries, or even custom directories youâve specified.
Why is it Important? đWithout PyConfig.module_search_paths, Python wouldnât know where to find the modules it needs to execute your code.
What is PyContextVar_Type? đImagine you are hosting a dinner party (your Python program), and youâve got multiple guests (tasks or coroutines) each with their own requirements (context). You want to ensure that each guest gets what they need without disrupting others. This is where PyContextVar_Type steps in â itâs like a magical butler who remembers the preferences of each guest, ensuring they are catered to individually.
In technical terms, PyContextVar_Type is part of Pythonâs contextvars module, which provides support for context-local state.
What is PyComplex_RealAsDouble? đPyComplex_RealAsDouble is a function provided by Pythonâs C API that extracts the real part of a complex number and returns it as a double-precision floating-point number (a standard Python float).
Think of complex numbers as a pair of numbers, one representing the real part and one representing the imaginary part. If you imagine a complex number as a house, the real part is the street address, and the imaginary part is like an apartment number within the building.
What is PyDict_New? đPyDict_New is a function in Pythonâs C API that creates a new, empty dictionary. Itâs like the blank canvas that an artist starts with before painting a masterpiece.
How is PyDict_New Used? đIn Python land, a dictionary is an essential data structure. When you call PyDict_New, youâre essentially saying, âHey, Python, I need a fresh, clean dictionary to store my key-value pairs.â Hereâs how you typically invoke it in C:
What is PyCFunction_NewEx? đPyCFunction_NewEx can be thought of as a key master in a grand castle of Python functionality. It deals with the creation and initialization of Python callable objects â think of these as specially-empowered keys that can open doors (run functions) faster than the usual keys (interpreted Python functions). Specifically, PyCFunction_NewEx focuses on creating built-in functions written in C, thereby combining Pythonâs user-friendliness with Câs performance.
How is it Used?
What is Garbage Collection? đBefore we tackle PyGC_Enable, letâs talk about the garbage collector. Imagine your computerâs memory as a big closet. Over time, you store various items (data). If you never clean it, the closet gets so full that you cannot find space for new items. Thatâs where the garbage collector comes inâitâs like a self-organizing system that automatically decides which items you no longer need and throws them away, ensuring that thereâs always room for new ones.
What is PyComplex_FromDoubles? đAt the simplest level, PyComplex_FromDoubles is a function in Pythonâs C API that creates a new complex number from two double-precision floating-point numbers. If youâve ever worked with complex numbers in Python, youâre used to seeing them as something like 3 + 4j. The PyComplex_FromDoubles function is like the backstage crew that makes this possible in the world of Python extensions and embedding C.
In English, it turns two real numbers into a complex number.
What is PyFloat_FromString? đSo, what exactly is PyFloat_FromString? Think of it as the invisible fairy godmother of numerical types in Python. Specifically, PyFloat_FromString is a C function in the Python/C API that turns a string into a floating-point number. Imagine you have a string that says â42.42â and you want to turn it into a floating-point number 42.42. Thatâs where PyFloat_FromString casts its spell and gets the job done.
How to Use PyFloat_FromString đWhile many of us enjoy the comfort of high-level Python functions like float(), itâs useful to peek behind the curtains.
What is PyNumberMethods.nb_or? đIn Python, PyNumberMethods is a structure that defines various methods for numerical operations such as addition, subtraction, multiplication, and more. Among these methods is nb_or, responsible for handling bitwise OR operations.
Bitwise OR Operation đBefore we dive deeper, letâs understand what a bitwise OR operation is. Imagine you have two binary numbers:
1010 (10 in decimal) 0110 (6 in decimal) A bitwise OR operation compares each bit of these numbers and returns a new binary number.
What is PyConfig.use_environment? đThink of PyConfig.use_environment as a switch that tells your Python interpreter whether to look at the environment variables for certain configurations. Like flipping a light switch to either illuminate a room or keep it dark, setting PyConfig.use_environment to 1 or 0 directs Python to either use environment settings or ignore them.
Environment variables are like invisible assistants in your operating system that provide essential configuration data to programs.
What is PyCFunction_New? đIn plain English, PyCFunction_New is a function in the Python C API that creates a Python-callable function object from a C function and a method definition. This allows you to write performance-critical code in C, and seamlessly call it from within Python as if it were a native Python function.
Think of PyCFunction_New as a bridge. On one side, you have Python, with its ease of use and flexibility.
What is PyConfig.int_max_str_digits? đThink of PyConfig.int_max_str_digits as a guard at the gate of numerical conversions. This setting is part of Pythonâs PyConfig structure, which allows you to configure various aspects of the Python runtime environment before it starts. Specifically, int_max_str_digits sets a boundary on the number of digits that can be converted when parsing a string to an integer.
In simpler terms: imagine you have a really, really long number stored as a string.
What is PyConfig.interactive? đBefore we dive deep, letâs start with a simple definition. PyConfig.interactive is a configuration setting within Pythonâs PyConfig structure. This setting determines if Python should operate in interactive mode, which is a mode where you can enter and execute Python commands one at a time, receiving immediate feedback.
Think of it like chatting with a friendly, witty interlocutor who gives you instant responses, rather than sending letters back and forthâremember those?
What Is PyModule_GetFilename? đPyModule_GetFilename is a function provided by Pythonâs C-API. While Python itself is written in C, it allows you to blend C with Python, giving you a way to extend Pythonâs capabilities. The PyModule_GetFilename function is your go-to method when you need to retrieve the filename from which a particular Python module was loaded.
How Does It Work? đBefore diving into how you use it, letâs peek under the hood and see how it works.
What Is PyEval_GetBuiltins? đThink of Python as a magical world where every wizard (programmer) can summon various spells (functions). In this analogy, PyEval_GetBuiltins is like a key to the grand library of these spells.
The Basics đPyEval_GetBuiltins is a function in Pythonâs C API that retrieves the dictionary of built-in objects. In simpler terms, it allows you to access all the pre-defined functions and variables that Python provides out of the box, like print(), len(), and None.
What is PyDict_AddWatcher? đImagine having a magical book in which every time someone made a note, you got an instant alert. In the Python world, dictionaries (dict objects) are akin to such books, holding key-value pairs. PyDict_AddWatcher is a function that allows us to âwatchâ for changes in these dictionariesâacting like a sensor that notifies us when modifications occur.
In technical terms, PyDict_AddWatcher allows you to register a callback function, often termed a âwatcher,â which will be called whenever a dictionary is modified.
What Is PyImport_ImportModuleEx? đIn simple terms, PyImport_ImportModuleEx is a function in Pythonâs C API that helps you import a module, along with optional globals and locals definitions. Think of it as a powerful reading assistant that fetches Python modules and makes them available for use within your code.
How to Use PyImport_ImportModuleEx đTo use this function, you generally would write code in C or C++ that interacts with the Python interpreter.
What is PyImport_ExecCodeModuleEx? đAt its core, PyImport_ExecCodeModuleEx is a function that allows you to dynamically create a module in Python, execute some code within it, and optionally assign it a unique name. Think of it as the Pythonic equivalent of a magic spell that conjures a new book (module) out of thin air, fills it with knowledge (code), and places it on your bookshelf (the module namespace) with a bespoke label if you so choose.
What is PyByteArray_FromObject? đPyByteArray_FromObject is a function in Pythonâs C-API used to create a bytearray object from various object types. Think of it as the magical gatekeeper that transmutes different objects into a mutable sequence of bytes. This concept might feel a bit abstract initially, but fear not! Weâll break it down.
How to Use PyByteArray_FromObject đHumans are creatures of habit, preferring to see things rather than hear about them. So, letâs take an illustrative tour with some code examples.
What on Earth is PyByteArray_Type? đIn Python, PyByteArray_Type is a fundamental building block in the languageâs C API (Application Programming Interface). Now, imagine Python itself as a gigantic hardware store. While you have ready-made hammers, nails, and planks ready for use (high-level constructs like lists, dictionaries, and strings), PyByteArray_Type is something like a raw lump of ironâextremely useful but requiring some craftsmanship to put into play.
To frame it in simpler terms, PyByteArray_Type is the C structure that underpins the bytearray object in Python.
What is PyConfig.base_prefix? đImagine PyConfig.base_prefix as the home address of your Python environment. Just as your home address tells you where you live, the base_prefix tells Python where its âhomeâ isâthe directory where the interpreterâs base environment is stored. This becomes particularly important when dealing with virtual environments.
How is it Used? đPyConfig.base_prefix is an attribute found within the PyConfig structure. Itâs utilized primarily in advanced configurations and custom Python builds, often by those delving deep into Pythonâs internals or working on projects requiring a great deal of environment control.
What is PyConfig.xoptions? đImagine youâre setting up a new phone. Youâve got your main features: texting, calling, and perhaps a couple of apps. Then there are the extra settingsâthe ones that let you tweak performance, turn on Do Not Disturb, or customize your notifications. These extra settings are akin to what PyConfig.xoptions does in Python. Itâs a way to pass additional, often optional, configurations to Pythonâs runtime environment that arenât covered by the standard settings.
What is PyDict_ClearWatcher? đPyDict_ClearWatcher is part of Pythonâs internal mechanics. Think of Python as a sprawling, enchanted library. At the heart of this library, PyDict_ClearWatcher operates like a diligent librarian. Its job isnât to see whoâs checking out books, but to keep tabs on when dictionariesâthose all-important, versatile data structuresâare being cleared out.
In Human Terms: đ PyDict: Short for âPython Dictionary,â one of the most versatile and ubiquitous objects in Python, akin to a magical chest that can store key-value pairs.
What is PyEval_GetGlobals? đIn the world of Python, PyEval_GetGlobals is like a backstage pass that lets you see all the global variables being used in your code. Think of it as having a magic mirror that shows you the global state of your Python interpreter at any given moment.
But before we dive into the how and why, letâs break this down.
The Cast of Characters: Local vs. Global đFirst off, letâs remind ourselves of the basics:
What is PyEval_SetProfile? đImagine youâre a detective trying to solve a mystery. To catch the culprit (in this case, any inefficient or problematic parts of your code), youâd need to keep a close eye on their actions. PyEval_SetProfile is like your custom surveillance camera for Python code execution. It allows you to set a profile function that will be called for various events during the execution of your Python code.
What is PyEval_SetTraceAllThreads? đAt its core, PyEval_SetTraceAllThreads is like a surveillance camera for all your Python threads. It allows you to set a tracing function that will watch over every thread running in your application. This tracing function can keep tabs on whatâs happening in your code, tracking events like function calls, line executions, exceptions, and even C function calls.
Hereâs a simple way to understand it: Imagine youâre hosting a juggling competition.
What is PyFile_FromFd? đImagine youâre given a key that can unlock doors to different rooms, each storing a file. In this analogy, a file descriptor (FD) is that key, but it is rather abstract and versatile, originating from the world of C and Unix-like operating systems. PyFile_FromFd acts as an interpreter for this key, translating it into something more familiar and usable in the Python realmâa file object.
In more concrete terms, PyFile_FromFd is a function in Pythonâs C API that creates a Python file object from a given file descriptor.
What is PyFunction_GetDefaults? đAt its core, PyFunction_GetDefaults is a C API function in Python that lets you retrieve the default values for the arguments of a Python function. Imagine you have a cooking recipe (your function), and occasionally, you donât feel like adding every single ingredient (the arguments with default values). PyFunction_GetDefaults allows you to see which ingredients (defaults) the recipe will automatically add on its own.
How to Use PyFunction_GetDefaults đFirst things first: PyFunction_GetDefaults isnât something youâll find in Python scripts directly.