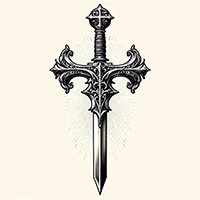
WE SHALL NEVER SURRENDER
What Exactly is PyException_GetCause? đImagine youâre reading a mystery novel, and every thrilling event is connected by a cause and effect chain. The climax of the story wouldnât make sense without understanding what causes each event, right? In Python, exceptions work similarly. When an error (or exception) occurs, it might be caused by another underlying problem. PyException_GetCause is the Sherlock Holmes of Python internals, helping you trace back the root cause of an exception.
What is PyException_SetArgs? đIn laymanâs terms, PyException_SetArgs is like a detectiveâs notepad in a Python program, recording details (arguments) about exceptions that occur. When your code runs into an error, exceptions pop up. These exceptions often carry messages with them, explaining what went wrong. PyException_SetArgs allows us to set these messages so that theyâre more informative.
The Role of PyException_SetArgs đImagine youâre baking a cake, and mid-way, you realize youâve run out of eggs.
What is PyException_SetCause? đImagine errors in Python as a stack of books. Sometimes, one error (letâs call it a book) is caused by another underlying error (another book). The function PyException_SetCause allows us to explicitly set this âcause,â essentially linking one error to another so that when you look at the stack of books, you understand the sequence of events that led to the topmost error.
How Itâs Used đPyException_SetCause is part of the Python/C API, so itâs not something you typically use in everyday Python scripts.
What is PyException_SetContext? đIn laymanâs terms, PyException_SetContext is like setting the stage for the next act in a play. When an exception occurs in Python, this function allows you to link the current exception to a new one. Imagine youâre watching a TV series; PyException_SetContext would be the âPreviously onâŚâ segment, reminding you where the story left off.
In more technical terms, PyException_SetContext sets the context of the current exception, and this context is the exception that was active when the current exception was raised.
What is PyFloat_CheckExact? đImagine hosting a dinner party and wanting to verify if each guest is exactly who they said they are, with no exceptions. PyFloat_CheckExact is like the bouncer at your party, except instead of checking IDs, itâs double-checking if a given object is exactly a floating-point number in Pythonâno more, no less.
How is PyFloat_CheckExact Used? đFirst, letâs take a birdâs-eye view of why you might need this. When youâre writing Python code, itâs usually Python that manages type checking for you.
What is PyFloat_Unpack4? đAt its heart, PyFloat_Unpack4 is a function used within Pythonâs C APIâsophisticated territory where Pythonâs high-level abstractions meet low-level operations. Specifically, PyFloat_Unpack4 takes a 4-byte string (you can think of it as a compact byte-sized treasure chest) and converts it into a Python float object.
How Is It Used? đWhile you wonât typically use PyFloat_Unpack4 in your everyday Python scripts, understanding its mechanism can enrich your comprehension of how Python works under the hood.
Understanding PyFrame_Check đImagine running a Python script as navigating through an intricate magic labyrinth. Each time you enter a new room (a new function or a new block of code), Python creates a detailed map of that room, known as a âframe object.â At the core of managing these frame objects is a function called PyFrame_Check.
In the simplest terms:
PyFrame_Check is a macro in Pythonâs C API that checks whether a given object is a frame object.
What is PyFrame_GetBuiltins? đThink of PyFrame_GetBuiltins as a backstage pass to Pythonâs built-in functions and variables. These built-ins are like the foundational blocks that help you perform essential operations without needing to define them yourself. Need to sum numbers, find the length of a list, or open a file? Pythonâs built-ins have got you covered.
How is PyFrame_GetBuiltins Used? đIn the realm of Pythonâs internal mechanics, PyFrame_GetBuiltins is utilized within the C APIâthe lower-level layer that underpins the high-level Python code you write.
Understanding PyFrame_GetGlobals đWhat is PyFrame_GetGlobals?
In simple terms, PyFrame_GetGlobals is a function provided by the Python C API. It retrieves the global namespace of a given stack frame. A stack frame, in Pythonâs context, is an execution contextâessentially, where the play is happening in memory. The global namespace is the collection of all globally available variables and functions at any point during execution.
Hereâs the formal definition:
PyObject* PyFrame_GetGlobals(PyFrameObject *frame)
What is PyFrame_GetLineNumber? đSimply put, PyFrame_GetLineNumber is a function from Pythonâs C API that allows us to retrieve the current line number being executed in a Python frame object. Think of a frame object as a snapshot of all the variables and execution contexts at a certain point in your code.
In essence, PyFrame_GetLineNumber tells us, âHey, Sherlock! Your code is running over here.â
How Do We Use It? đTo use PyFrame_GetLineNumber, we need to delve into some internals of Python, often for advanced debugging or profiling.
What is PyFrame_GetVarString? đPyFrame_GetVarString is a function not commonly discussed in the context of everyday Python programming but deeply embedded within the Python interpreterâs C API. This function primarily serves those who delve into Pythonâs internals or those developing advanced debugging tools. It allows you to retrieve the value of a local variable within a specified frame and return it as a string.
How is PyFrame_GetVarString Used? đIn general Python programming, you typically wonât encounter PyFrame_GetVarString directly.
Whatâs in a (Frozen)Set? đBefore diving deep, letâs take a moment to understand the concept of a frozenset. While a regular set in Python is mutable (think of it as a magical bag where you can add and remove items freely), a frozenset is immutable (like a perfectly preserved artifact in a museumâuntouchable and unchangeable). This immutability guarantees that once created, the contents of a frozenset cannot be altered, making them hashable and thus usable as keys in dictionaries or elements of other sets.
What Is PyFrozenSet_Type? đThink of PyFrozenSet_Type as the ironclad sibling of PySet_Type. While regular sets in Python are mutable (you can add and remove items), frozensets are immutableâthey cannot be altered once created. This immutable nature makes frozensets hashable, meaning they can be used as keys in dictionaries or elements of other sets, neither of which mutable sets can do.
How Is It Used? đCreating a frozenset đCreating a frozenset is as straightforward as pie:
What is PyFunction_ClearWatcher? đImagine youâre the director of a live play. You have a number of actors (functions) performing on stage (your program), and a crew of watchers (callbacks) observing them to ensure everything runs smoothly. Sometimes, though, you need to send some watchers home because their job is done or their presence is no longer necessary.
PyFunction_ClearWatcher is like the director in this analogy. Itâs a Python C API function that clears, or removes, watchers (or callbacks) monitoring Python functions via the internal function watcher mechanism.
What is PyFunction_GetGlobals? đIn Python, the PyFunction_GetGlobals function is part of the CPython API, which is the implementation detail behind Python. This function serves to fetch the global variables associated with a given Python function. If youâre imagining Python functions as magic spells, then global variables are like ancient scrolls kept in a library. And PyFunction_GetGlobals is the library assistant that fetches these scrolls for you!
How is PyFunction_GetGlobals Used? đBefore we get hands-on, letâs make one thing clear: PyFunction_GetGlobals is typically used in the internals of Python and in C extensions, meaning you wonât often need it just for casual Python scripting.
What Does PyFunction_SetAnnotations Do? đPyFunction_SetAnnotations is a function within Pythonâs C API (Application Programming Interface). Itâs specifically designed for working with function annotations, which are a way to attach metadata to function parameters and return values. These annotations can be anythingâtypes, descriptions, or even custom objects. Essentially, this function allows you to set the __annotations__ attribute of a Python function.
Think of function annotations like adding sticky notes with extra information to the parameters and results of your function.
What is PyFunction_Type? đAt its core, PyFunction_Type represents Python functions at the C API level. If Python is the car, PyFunction_Type is a crucial part of its engine, making sure your function calls run smoothly. Itâs where all the magic happens behind the scenes when you call functions in Python.
How is PyFunction_Type Used? đFor most Python beginners, your interaction with functions looks like this:
def greet(name): return f"Hello, {name}!" print(greet("World")) You call greet and get âHello, World!
What is Garbage Collection in Python? đBefore diving into PyGC_IsEnabled(), we need to understand what garbage collection means in Python. Picture this: youâre coding away, creating variables and objects left and right. Each of these occupies some memory. Over time, you might forget about some of these variables because theyâre no longer needed. Despite being abandoned, these variables still take up space, like uninvited guests overstaying their welcome.
This is where garbage collection comes in.
What is PyGen_CheckExact? đLetâs start with the basics. PyGen_CheckExact is a function used internally in Pythonâs C code. To put it simply, itâs like a bouncer at a club door, checking IDs to confirm if an object is indeed an exact generator object. If it is exactly a generator, PyGen_CheckExact gives it a nod (returns true); if not, it sternly shakes its head (returns false).
Why is PyGen_CheckExact Important? đYou might wonder, âWhy do we need to confirm if an object is exactly a generator?
What is PyGen_New? đPyGen_New is a function in Pythonâs C API that creates a new generator object. In simpler terms, itâs like a master chef who crafts new dishes (generator objects) on demand, one at a time. When you use the yield keyword in a Python function, youâre crafting a generator function. Once your generator function starts running, PyGen_New is called under the hood to handle the creation of the generator object.
What is PyGetSetDef.closure? đTo put it simply, PyGetSetDef.closure in Python is part of the Python C-API, a collection of functions and structures that allow Python to interface with C code. If you think of Python as a beautifully painted house, the C-API is the robust framework hidden beneath the paint.
Specifically, PyGetSetDef.closure is a field in the PyGetSetDef structure. This structure is used to define properties in Python objects when extending Python in C.
What Is PyGetSetDef.doc? đTo put it simply, PyGetSetDef is a structure in Pythonâs C-API (Application Programming Interface) that allows you to define how to get and set attributes in objects written in C. The .doc part is a string attribute within this structure that provides a description or documentation for the attribute you are getting or setting.
Think of PyGetSetDef as a more formal job description for Python attributes. Just like a job description tells you what a personâs role involves, PyGetSetDef specifies how to interact with a particular attribute.
What is PyGetSetDef.get? đPyGetSetDef is a structure used in Pythonâs C API to define getter and setter functions associated with object attributes. Think of it as a gatekeeper that knows how to fetch (get) or modify (set) the attributes of an object when asked. This structure is particularly important when youâre creating custom objects in C that you want to interact with in Python.
Understanding PyGetSetDef Structure đThe PyGetSetDef structure has the following fields:
What is PyGetSetDef.name? đImagine PyGetSetDef as a closet organizer. Instead of holding clothes, it organizes the getter and setter methods for your Python object properties. Now, every item in this closet needs a label so you can quickly find it. This is where .name comes in: itâs the label for each item in your PyGetSetDef structure.
In technical terms, PyGetSetDef is a structure used in the Python C API to define getter and setter functions for object attributes.
What is PyGILState_Check? đThe function PyGILState_Check is part of the Python C API and it checks the current state of the Global Interpreter Lock (GIL). In simple terms, the GIL is a mutex (or a lock) that protects access to Python objects, preventing multiple native threads from executing Python bytecodes at once. This ensures that only one thread runs in the Python interpreter at any time.
To put it into a metaphor, imagine a single-lane bridge in a busy city.
The Global Interpreter Lock (GIL) đLetâs start with the GIL, a concept that might seem a bit like a plot twist in a superhero movie. Imagine the GIL as a traffic cop in a one-lane tunnel who ensures cars (in this case, Python threads) pass through without crashing into each other. It enforces that only one thread executes Python bytecodes at a time, preventing race conditions that can lead to the dire consequences of data corruption.
What is PyHash_FuncDef.hash_bits? đTo put it simply, PyHash_FuncDef.hash_bits is a constant that defines the number of bits used by Pythonâs hash functions. In more technical terms, this value directly impacts the way objects like strings, integers, and custom objects are stored and retrieved in data structures like dictionaries and sets.
Why Do We Even Need Hashing? đBefore we get into the nitty-gritty, letâs clarify why hashing is essential. Imagine you have a huge library of books, and you need to find a particular book.
What is PyHash_FuncDef.seed_bits? đImagine youâre baking cookies, and you want each batch to have a unique flavor. The secret ingredient isnât just the recipe, but also a little touch of randomness. In Python, PyHash_FuncDef.seed_bits is a specific parameter tied to the ârecipeâ of generating hash values. These hashes are unique and vital for data structures like dictionaries and sets, ensuring data retrieval is as efficient as a well-tuned bakery.
How is PyHash_FuncDef.
What is PyImport_AddModule? đImagine youâre hosting a grand banquet. You have guests (functions and variables) scattered all around, and you need a way to gather them into one place so they can interact seamlessly. PyImport_AddModule acts like a diligent host. It either retrieves an existing module from your Python environment (the banquet), or if the module isnât already there, it creates a new one.
In more technical terms, PyImport_AddModule is a function in Pythonâs C API that ensures a module exists in the sys.
What is PyImport_AddModuleObject? đPyImport_AddModuleObject is a function in Pythonâs C API that plays a critical role in managing Python modules from within C extensions. Think of it as the backstage manager who ensures that modules are found and staged correctly during your programâs runtime.
To put it simply, PyImport_AddModuleObject adds a module object to the internal modules dictionary, making it accessible for import within your Python environment.
How is PyImport_AddModuleObject Used?