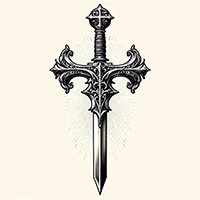
WE SHALL NEVER SURRENDER
What is PyByteArray_Concat? 🔗In simple terms, PyByteArray_Concat is a function that joins two byte arrays together. Think of it as the seamstress in a digital tailor shop — its job is to stitch two pieces of fabric (byte arrays) into one seamless cloth. Byte arrays in Python are sequences of bytes, which aren’t usually human-readable but are essential in handling binary data.
Technical Definition đź”—PyByteArray_Concat is a C function in the Python C API that takes two PyObject pointers (which should point to byte arrays) and returns a new PyObject pointer representing the concatenated byte array.
What Is PyByteArray_CheckExact? 🔗PyByteArray_CheckExact is a function in Python’s C-API, designed to check if a given object is exactly a bytearray type. It doesn’t just determine if an object behaves like a bytearray, but ensures it is precisely a bytearray object and not a subclass thereof.
Think of it like checking ID at a nightclub. The bouncer (our function) isn’t just verifying that you’re of legal age (which could be a general bytearray behavior); they’re making sure you’re exactly who your ID says you are (the true bytearray type).
What is PyByteArray_Check? 🔗Imagine you’re organizing a big party and you need to check if each guest is on your VIP list. In this metaphor, the guests are various objects in your Python program, and the VIP list is a specific type of object called a bytearray. The function PyByteArray_Check is like the bouncer at your party door—it checks whether a given object is a bytearray or not.
How Does PyByteArray_Check Work?
use PyByteArray_AsString when you need safety and type checking, especially in code that interfaces with external or less-controlled inputs. Use PyByteArray_AS_STRING when you need maximum performance and can ensure that the object passed is indeed a bytearray. Balancing safety and performance appropriately will help you write robust and efficient C extensions for Python.
PyByteArray_AsString đź”—PyByteArray_AsString is a function provided by the Python C API. It performs a check to ensure that the object passed to it is indeed a bytearray object before returning a pointer to the underlying data buffer.
What is PyByteArray_AsString? 🔗Imagine you’re trying to read a secret message written on a scroll. The scroll itself is like a byte array in Python—a sequence of bytes representing data. To understand the message, you need to unfurl the scroll and look at the characters written on it. That’s where PyByteArray_AsString comes in. This function is like a magical spell that transforms the byte array (the scroll) into a readable string (the message).
What is PyByteArray_AS_STRING? 🔗In simple terms, PyByteArray_AS_STRING is a macro provided by Python’s C API. It is used to obtain a pointer to the underlying buffer of a bytearray object. This pointer can then be used to access or manipulate the bytearray’s content directly at the C level.
Imagine a bytearray as a box full of candies. Each candy represents a byte. The PyByteArray_AS_STRING function gives you a direct hand into the box, allowing you to grab and interact with the candies (bytes) without any intermediaries.
What is PyBuffer_ToContiguous? 🔗Imagine you have a library of books (your data), but they’re scattered across different shelves and sections (memory locations). You want to gather all those books and place them neatly on one single shelf (a contiguous block of memory). PyBuffer_ToContiguous is like a librarian that helps you do this.
In technical terms, PyBuffer_ToContiguous is a function in the Python C API that copies data from a possibly non-contiguous buffer into a contiguous buffer.
What is PyBuffer_SizeFromFormat? 🔗Imagine you’re building a house (your data buffer) and you have a list of materials (the format string) that tells you how much of each material you need. The PyBuffer_SizeFromFormat function is your construction guide that calculates the total amount of materials required. In simpler terms, it calculates the total size (in bytes) of the buffer needed to store data described by a given format string.
How is it Used?
What is PyBuffer_Release? 🔗PyBuffer_Release is a function in Python’s C API, specifically designed to manage memory when dealing with buffer objects. Think of a buffer as a temporary storage space for data being moved from one place to another, like a waiting room for data in transit.
When Python code interacts with C extensions or low-level operations that handle data buffers, proper management of these buffers is crucial to avoid memory leaks and other issues.
What is a Buffer? đź”—Think of a buffer as a line of people waiting to get into a movie theater. Each person (or piece of data) has a specific spot. In Python, a buffer is a block of memory that stores data in a specific format.
What Does Contiguous Mean? đź”—Contiguous means that the data in memory is stored without any gaps. Imagine our line of people again, but now, everyone is holding hands.
What is PyBuffer_GetPointer? đź”—In simple terms, PyBuffer_GetPointer is a function that allows you to get a direct pointer to the data in a Python buffer object. This can be incredibly useful when you need to manipulate data at a low level, such as in C extensions or when dealing with complex data structures.
How Does it Work? 🔗To understand how PyBuffer_GetPointer works, let’s first talk about what a buffer is. In Python, a buffer is essentially a contiguous block of memory.
What is PyBuffer_FromContiguous? đź”—Think of PyBuffer_FromContiguous as a master organizer at a buffet (pun intended!). It takes an existing array of data and arranges it into a continuous block of memory. This is especially handy when working with data that needs to be contiguous (i.e., in one uninterrupted block), which is a common requirement in lower-level programming and data manipulation tasks.
How is PyBuffer_FromContiguous Used? 🔗Imagine you’re sorting Lego bricks by color.
What is PyBuffer_FillInfo? 🔗Imagine you have a storage unit (a buffer) and you need to organize and provide details about its contents. PyBuffer_FillInfo is the helper function in Python that does just that—it fills in the details about a buffer. This function is part of Python’s buffer protocol, which allows different Python objects to share memory buffers efficiently.
What Does PyBuffer_FillInfo Do? đź”—PyBuffer_FillInfo populates a Py_buffer structure with information about a buffer.
What is PyBuffer_FillContiguousStrides? đź”—Imagine you have a giant spreadsheet, but instead of neatly arranged rows and columns, all the data is jumbled up in one long, continuous strip. Your task is to figure out how to navigate this strip as if it were still in its original grid form. This is essentially what PyBuffer_FillContiguousStrides helps you do in Python.
PyBuffer_FillContiguousStrides is a function in Python’s C-API that deals with the buffer protocol.
What is PyBufferProcs.bf_releasebuffer? 🔗Imagine you have a fantastic library, and you’ve borrowed a book. After reading it, you don’t just throw it back on the shelf; you return it properly so others can find it. Similarly, in Python, when you’ve finished using a buffer (a chunk of memory used to store data), you need to release it properly. That’s where PyBufferProcs.bf_releasebuffer comes into play.
PyBufferProcs.bf_releasebuffer is a function pointer in the PyBufferProcs structure.
What is PyBufferProcs.bf_getbuffer? 🔗Imagine Python as a bustling office, and data as the coffee that keeps everything running smoothly. Now, when different departments (objects) need to share their coffee (data), they don’t just pass the mug around—that would be inefficient and messy. Instead, they use a sophisticated coffee machine (buffer protocol) that allows them to share coffee seamlessly. The bf_getbuffer function is like the barista of this coffee machine, making sure each department gets their coffee just the way they like it.
What is PyBool_Type? 🔗In Python, PyBool_Type represents the type object for the boolean values True and False. It’s an internal structure used by the Python interpreter to handle boolean values, ensuring they are treated as a distinct type while also maintaining compatibility with integer operations. Essentially, PyBool_Type underpins the bool type in Python.
How is PyBool_Type Used? đź”—In everyday Python programming, you might not directly interact with PyBool_Type. Instead, you use the built-in bool type, which is a high-level representation of PyBool_Type.
What is PyBool_FromLong? 🔗PyBool_FromLong is a function in the Python C API that converts a long integer to a Boolean value. Specifically, it takes an integer (or long) and returns Py_True if the integer is non-zero and Py_False if the integer is zero. In Python terms, it’s akin to using the bool() function to convert a number to a Boolean value.
How is PyBool_FromLong Used? đź”—The PyBool_FromLong function is used in C extensions for Python.
Understanding PyBool_Check in Python 🔗What is PyBool_Check? 🔗In simple terms, PyBool_Check is a function used to verify if a given object is a Boolean (True or False). Imagine you have a box and you’re not sure if it contains a light bulb. PyBool_Check is like a tool that helps you check if the object inside the box is indeed a light bulb.
How is PyBool_Check Used? đź”—When writing Python code that interacts with C, you might come across situations where you need to check the type of an object.
What is PyAsyncMethods.am_send? 🔗PyAsyncMethods.am_send is a method in Python’s C API, specifically designed for asynchronous programming. It is part of the PyAsyncMethods structure, which defines a set of methods that an asynchronous iterator must implement. The am_send method is responsible for sending a value into an asynchronous generator.
How is PyAsyncMethods.am_send Used? 🔗To understand the usage of PyAsyncMethods.am_send, let’s first look at a high-level overview of asynchronous generators in Python. An asynchronous generator is a function that yields values asynchronously, allowing for more efficient handling of I/O-bound tasks.
What is PyAsyncMethods.am_await? 🔗PyAsyncMethods.am_await is a part of Python’s C API that handles asynchronous operations. Specifically, it is a function pointer that is used to define how the await operation should be implemented for a particular type. In simpler terms, when you use the await keyword in Python, this function is what gets called under the hood to manage the asynchronous behavior.
How is PyAsyncMethods.am_await Used? 🔗In typical Python programming, you won’t directly interact with PyAsyncMethods.
What is PyAsyncMethods.am_anext? đź”—PyAsyncMethods.am_anext is a method used in Python to retrieve the next item from an asynchronous iterator. An asynchronous iterator is an object that implements the asynchronous iteration protocol, allowing you to iterate over it using async for loops.
Think of an asynchronous iterator like a conveyor belt in a factory. Items (data) are produced and placed on the belt, but it might take some time for each item to be ready.
What is PyAsyncMethods.am_aiter? đź”—PyAsyncMethods.am_aiter is a method in Python that returns an asynchronous iterator. An asynchronous iterator is an object that implements the asynchronous iteration protocol, allowing you to loop over data using asynchronous methods.
Why Use Asynchronous Iterators? đź”—Asynchronous iterators are useful when dealing with I/O-bound operations like reading from a file, fetching data from a network, or interacting with databases. They allow your program to perform other tasks while waiting for these operations to complete, rather than blocking the entire program.
What Does PyArg_ValidateKeywordArguments() Do? 🔗Imagine you’re hosting a party, and you’ve got a guest list. You want to make sure that everyone who shows up is actually on the list and not some random party crasher. PyArg_ValidateKeywordArguments() is your bouncer. It checks the keyword arguments passed to a function, ensuring they are all legitimate and accounted for.
How Is It Used? đź”—This function is primarily used in the context of writing C extensions for Python.
What the Heck is PyArg_VaParseTupleAndKeywords()? 🔗Imagine you’re at a party, and you’re the host. You need to make sure everyone’s having a good time and got what they came for. In the Python world, PyArg_VaParseTupleAndKeywords() is like that meticulous party host. It takes care of the arguments your Python function receives, ensuring they’re all in order, both positional (like “chips”) and keyword (like “guacamole: extra spicy”).
So, What Exactly Does It Do?
What on Earth is PyArg_VaParse()? 🔗Imagine you’re at a magical creature petting zoo, and you’ve got a bunch of creatures (arguments) that you need to identify and sort. PyArg_VaParse() is like your trusty guidebook. It helps you decipher and handle the arguments passed to a Python function when you’re working with C code.
In plain English: PyArg_VaParse() is used in C extensions for Python to parse the arguments passed to a function.
What is PyArg_UnpackTuple()? 🔗Imagine you’ve got a suitcase packed with all your favorite things: socks, a toothbrush, and that weird souvenir you picked up on vacation. Now, you need a way to grab specific items without rummaging through the whole thing. That’s where PyArg_UnpackTuple() comes in. It’s your helpful little function for unpacking the suitcase (tuple) and making sure you’ve got exactly what you need.
How Does it Work? 🔗Here’s the basic idea: you pass a tuple to PyArg_UnpackTuple(), and it neatly unpacks it into individual items.
What’s PyArg_ParseTupleAndKeywords()? 🔗Picture this: You’re at a party. Someone hands you a list of drinks (that’s the tuple) and a list of preferences (those are the keywords). Your job? Make sense of it all without losing your mind. That’s what PyArg_ParseTupleAndKeywords() does in the Python C API world. It’s the life of the party, parsing arguments and keyword arguments passed to a function.
PyArg_ParseTupleAndKeywords() is like the ultimate party host in the Python C API.
What’s the Deal with PyArg_ParseTuple()? 🔗Imagine you’re hosting a grand feast, and you’ve invited all sorts of Python objects—integers, strings, lists, you name it. But here’s the catch: your kitchen only speaks C. To make sure your feast is a success, you need to translate those Python objects into something your C kitchen can understand. Enter PyArg_ParseTuple(), the magical translator!
The Incantation đź”—The basic spell incantation looks like this:
int PyArg_ParseTuple(PyObject *args, const char *format, .
What the Heck is PyArg_Parse()? 🔗Imagine you’re at a fancy dinner party. You’ve got a bunch of dishes laid out (that’s your arguments), and you’ve got a bunch of hungry guests (that’s your variables) who want to be fed specific dishes. PyArg_Parse() is like the host who makes sure each guest gets the right dish. In more technical terms, it’s a function used in Python C extensions to convert Python objects into C values.