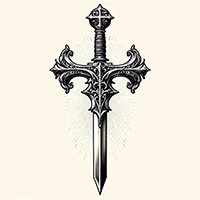
WE SHALL NEVER SURRENDER
What is PyMemberDef.offset? đTo kick things off, PyMemberDef.offset is part of the Python C API (Application Programming Interface). The C API is like the backstage pass to Pythonâs inner workings, the complex machinery that churns under the hood to make your delightful Python code run smoothly. Now, imagine if Python itself was a theater; PyMemberDef.offset would be one of the stagehands, making sure everything runs smoothly without stepping into the limelight.
What is PyModule_GetState? đAt its core, PyModule_GetState is a function provided by Pythonâs C API. In simpler terms, itâs like a chefâs secret drawer that holds the current ingredients (state) of a given module. This function retrieves the internal state of a module, which is useful when working with extension modules (modules written in C rather than pure Python).
Why Do We Need PyModule_GetState? đIf youâve ever tried to remember where you last placed your seasoning while cooking, you understand the importance of maintaining state.
What Exactly is PyNumberMethods.nb_lshift? đIn Pythonâs core, PyNumberMethods is a structure that defines behavior for numeric types like integers and floats. Think of it as an instruction manual for numbers, outlining how they should respond to various operations. One such operation is the left bit shift, represented by <<.
nb_lshift is a function pointer in the PyNumberMethods structure. This pointer tells Python how to perform the left bit shift operation (think: num « shift_amount) on our numeric types.
What is PyList_GetSlice? đSimply put, PyList_GetSlice is a function that allows you to extract a specific section (or slice) from a Python list. Think of it like slicing a cake â you decide the starting point and the ending point, and voila, you get a perfect slice without cutting the entire cake into pieces.
Now, letâs break down its components:
Basic Syntax: đPyObject* PyList_GetSlice(PyObject *list, Py_ssize_t low, Py_ssize_t high) list: The original list from which you want to extract a slice.
What is PyModule_AddType? đImagine Python as a grand stage play. On this stage, Python objects are the actors, moving and interacting in finely choreographed routines. But what if we want to introduce new characters with unique abilities to our play? Thatâs where PyModule_AddType comes inâit helps us add a new kind of actor, or a type, to our module.
In essence, PyModule_AddType is a function used in the Python/C API to introduce a new type object directly into a module.
What is PyEval_SaveThread? đImagine youâve got a fantastic juggler (Python interpreter) whoâs adept at keeping multiple plates (threads) in the air. However, this juggler can only handle one plate safely by themselves at a time due to their peculiar juggling technique. This limitation is akin to Pythonâs GIL, which ensures that only one thread executes Python bytecode at a time, even in multi-threaded applications.
Now, what if our juggler (interpreter) needs to take a break without dropping all the plates (threads)?
What is PyLong_AsVoidPtr? đTo put it simply, PyLong_AsVoidPtr is a function that converts a Python integer object (of type PyLongObject) into a void pointer (void*). If youâre venturing into integrating Python with C or C++ code, this function could become your go-to tool for translating numeric values into pointer addresses.
How is it Used? đHereâs an example to get a sense of how you might use PyLong_AsVoidPtr:
#include <Python.h> void some_c_function() { // Suppose you have a Python integer PyObject* py_int = PyLong_FromLong(42); // Creating a Python integer with value 42 // Convert the Python integer to a void pointer void* ptr = PyLong_AsVoidPtr(py_int); // Do something with the pointer (in this example, we'll just print it) printf("Void pointer: %p\n", ptr); // Decrement reference count for the PyObject Py_DECREF(py_int); } In this snippet, PyLong_FromLong creates a Python integer object from a C long, and PyLong_AsVoidPtr converts it into a void* pointer.
What is PyFloat_AsDouble? đImagine you have a magical sieve. This sieve can sift through different kinds of objects and pluck out the essential double (floating-point number) hidden inside. Thatâs pretty much what PyFloat_AsDouble does in the realm of Python. Itâs a C API function that extracts a C double from a Python float or an object that can be converted to a float. Think of it as the translator between the Python world of dynamic typing and the C world of static typing.
What Exactly is PyDateTime_TimeType? đImagine PyDateTime_TimeType as the sophisticated clockmaker in Pythonâs vast library, specializing in creating and managing time objects. Itâs essentially a data type that the Python C API uses to represent time without date information.
Where Does PyDateTime_TimeType Come From? đThe datetime module in Python is a suite of classes encompassing everything related to date and time. PyDateTime_TimeType is the internal, low-level type used by the time class within this module.
What is PyCapsule_GetPointer? đImagine you have a treasure chest (the PyCapsule) that holds a precious gem (a pointer to a C object). PyCapsule_GetPointer is like the key to that treasure chest. It retrieves the gem inside, allowing you to access the valuable object stored within.
In precise terms, PyCapsule_GetPointer is a function in Pythonâs C API used to extract a pointer to an object that is wrapped inside a PyCapsule. If youâve wrapped a C pointer in a Python capsule object, this function helps you retrieve it.
What is PyLong_FromUnicodeObject? đAt its core, PyLong_FromUnicodeObject is a function in Pythonâs C API that takes a Unicode object (essentially a string) and converts it into a Python integer (PyLongObject). This function is particularly useful when youâre writing C extensions or embedding Python in a C program and need to handle numeric data stored as text.
Hereâs the magical incantation for PyLong_FromUnicodeObject:
PyObject* PyLong_FromUnicodeObject(PyObject *u, int base); It takes two parameters:
What is PyCode_GetFirstFree? đTo describe PyCode_GetFirstFree, letâs take a quick trip under Pythonâs hood, particularly into the world of Python bytecode. When you write a Python script, the interpreter converts your high-level code into bytecode, a low-level, intermediate representation of your program. This bytecode is then executed by the Python Virtual Machine (PVM).
PyCode_GetFirstFree is a function in the Python/C API. Simply put, it returns the index of the first free variable in the given code objectâs list.
What is PyNumberMethods.nb_bool? đBefore diving in, letâs break down the jargon. PyNumberMethods is a structure in Pythonâs C API that holds pointers to functions to implement various numerical operations on objects. Think of it as a blueprint for how Python objects should behave with numbers.
Among these operations, nb_bool stands out. Itâs a function pointer used to determine an objectâs truth value. In simpler terms, nb_bool helps Python decide if an object should be considered True or False.
What is PyByteArray_FromStringAndSize? đIn essence, PyByteArray_FromStringAndSize is a function from the Python C API (Application Programming Interface) that allows developers to create a new bytearray object from a string, or more precisely, a sequence of bytes. Think of it as a factory that takes raw ingredients (bytes) and assembles them into a bytearray.
Bytearray: The Building Blocks đFirst, letâs get acquainted with bytearray. A bytearray in Python is a mutable sequence of bytesâessentially a list of integers where each integer represents a byte, ranging from 0 to 255.
What is PyBytes_AsString? đImagine a Python bytes object as a string of pearls, where each pearl represents a byte. What PyBytes_AsString does is quite magical: it turns this string of pearls into a long, coherent strandâa C-style string, to be exact. In other words, it provides a conversion from a Python bytes object to a C char*.
When and Why Would You Use It? đWhy would you need to make this conversion?
What Is PyBytes_AsStringAndSize? đImagine PyBytes_AsStringAndSize as a magical key that unlocks direct access to the contents of a Python byte string object and its size. From a more technical standpoint, this function allows us to obtain a pointer to the actual byte data contained within a bytes object and simultaneously retrieve the length of this data.
In more relatable terms, think of PyBytes_AsStringAndSize as a librarian. When you give it a specific book (a bytes object), it opens the book and hands you a slip of paper that tells you exactly how many pages are in the book (the length) and lets you look directly at the content inside the book (the raw bytes).
What is PyBytes_Concat? đPyBytes_Concat is a function used in the CPython API to concatenate two byte objects. Letâs emphasize that these are byte objects (bytes), not strings (str). Itâs a bit like if strings were sandwiches and bytes were energy barsâboth are essential but serve different cravings.
Function Signature đHereâs the concise way to declare this function in C:
void PyBytes_Concat(PyObject **bytes1, PyObject *bytes2); Parameters: đ PyObject **bytes1: A pointer to the first byte object.
What is PyBytes_GET_SIZE? đTo keep it straightforward, PyBytes_GET_SIZE is a function used in Pythonâs C API to retrieve the size of a bytes object. Think of it as a measuring tape specifically designed for bytesâwhen you need to know how many bytes are in your byte object, you reach for this tool.
Why Does Size Matter? đBefore we jump into the function itself, letâs touch upon the significance of knowing the size of a byte object.
What is PyBytes_Size? đThink of PyBytes_Size as a trusty tape measure for your Python bytes objects. Just as a tape measure tells you how long your lumber is, PyBytes_Size tells you the sizeâor lengthâof a bytes object in Python.
What Does It Do? đThe PyBytes_Size function is part of Pythonâs C API, a set of functions provided to interact with Python objects at the C level. Specifically, this function returns the size of a bytes object.
What is PyCallable_Check? đImagine youâve just arrived at a magical library. In this library, certain books have the ability to read themselves to you, while others do not. Your task is to determine which books have this magical ability. In this metaphor, PyCallable_Check is like a magical spell that reveals whether a particular book (or, in our case, an object) has the ability to âcallâ (i.e., perform a callable operation).
What Is It Used For? đConsider situations where you have a generator or an object you want to iterate over using a defined set of rules. This function is your go-to tool for these custom iteration patterns. A common use case could be reading lines from a file until a particular line is encountered.
How Does It Work? đThink of PyCallIter_New as a factory for iterators. This factory needs two pieces of information to start rolling out its custom-made iterators:
What is PyCapsule_GetContext? đImagine youâre a librarian, and youâve got a special library card that gives you access to some exclusive, behind-the-scenes books that regular members canât even see. In this metaphor, PyCapsule_GetContext is like a hidden key that lets you access those exclusive books.
In technical terms, PyCapsule_GetContext is a function from Pythonâs C API used to retrieve the context associated with a capsule object. Capsules in Python are opaque pointers that are meant to hide the details of the underlying data to promote safety and encapsulation.
What is PyCapsule_GetDestructor? đImagine you have a way to encapsulate (or package) a certain piece of data and its magical way of dismantling (destructing) itself when itâs no longer needed. Thatâs what a PyCapsule does for C extensions in Python. In simpler terms, PyCapsule allows Python to hold a reference to a piece of C code or data structures cleanly.
The PyCapsule_GetDestructor function fetches the cleanup function (or destructor) associated with a PyCapsule.
What Is a PyCapsule? đImagine youâre moving into a new house. You have some precious itemsâfamily heirlooms, invaluable antiquesâthat you want to store safely. You decide to store them in a capsule, which is a robust container ensuring their safety.
In Python, a PyCapsule is like that container. Itâs used to safely store and manage pointers to C objects, making sure they are handled correctly when used in Python applications. This is incredibly important when you are interfacing Python with C/C++ code.
What is PyCapsule_SetName? đIn laymanâs terms, PyCapsule_SetName is a function that sets or changes the name of a PyCapsule object in Pythonâs C API. To truly appreciate its utility, letâs first break down what a PyCapsule is.
Imagine a PyCapsule as a secure package (like a little digital gift box) that can store a pointer to a C object along with some metadata. This package allows us to safely pass around C pointers within Python code without worrying about accidentally messing with Pythonâs memory management system.
What is PyCapsule_SetPointer? đImagine a Python capsule as a magic box that stores C pointers (think: addresses to specific pieces of data or functions in C). PyCapsule_SetPointer is the function that changes this hidden pointer inside the capsule.
In other words, PyCapsule_SetPointer is like the wizard who opens the box, swaps out the current magic trick with a new one, and then seals it back up. This allows you to manipulate low-level C data types from within Pythonâa nifty trick for interfacing with C libraries, optimizing performance, or handling complex tasks requiring fine-grained control.
What is pyCode_ClearWatcher? đThink of pyCode_ClearWatcher as a vigilant lifeguard at the swimming pool of your Python code. It constantly watches over variables, alerting you when something goes awry. This tool belongs to a family of âwatchersâ used in debugging processes to monitor various elements within your program.
Why Use pyCode_ClearWatcher? đWhen debugging, knowing the state of your variables at any given moment is crucial. If a variable unexpectedly changes or holds an unintended value, it can break your code.
What is PyCode_GetCode()? đIn simplest terms, PyCode_GetCode() is a lower-level function used internally by Python to retrieve the bytecode of a compiled code object. Think of it as a way to tap into the âDNAâ of your Python script, revealing the series of low-level instructions (bytecode) that the Python interpreter will execute.
How is it Used? đTypically, you wonât have to use PyCode_GetCode() directly in most everyday coding tasks. However, understanding it can give you insights into how Python executes your code, which can be particularly useful for advanced debugging or crafting Python extensions in C.
What is PyCode_GetFreevars? đPyCode_GetFreevars is a function provided by Pythonâs internal C-API that allows you to retrieve the names of the free variables used by a specific code object. Before your eyes glaze over, letâs break that down.
To understand PyCode_GetFreevars, you need to grasp what âfree variablesâ are. In Python, free variables are variables that are used in a function but are not defined there. Instead, they are defined in an outer scope.
What is PyCode_Type? đLetâs start with the basics. PyCode_Type is an internal type in Python that represents code objects. Code objects are essentially the low-level building blocks of executable Python code. Think of them as the special recipes that tell the Python interpreter precisely how to execute your high-level instructions.
What PyCode_Type does is encapsulate the bytecode, the stack size, the constants, and other crucial details that the Python interpreter needs to run your code.