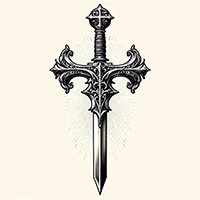
WE SHALL NEVER SURRENDER
What is PyNumberMethods.nb_power? đIn simple terms, PyNumberMethods.nb_power is a function pointer (yes, a pointer, but letâs not run off scared yet!). In the context of Pythonâs C API, itâs a part of the PyNumberMethods structureâthink of this as a recipe that tells Python how to handle arithmetic operations for a specific type of object. Specifically, nb_power is responsible for defining the behavior of the power operation, which is typically represented by the ** operator in Python.
What is PyConfig_Clear? đThink of Pythonâs PyConfig as the blueprints of a custom-built house. The PyConfig structure contains various settings that configure the Python runtime, including memory allocators, path configurations, and more. Now, imagine after building the house, you want to clean up the blueprints to make sure you leave no unnecessary clutterâthis is where PyConfig_Clear comes into play.
PyConfig_Clear is a function used to reset the PyConfig structure by clearing any dynamically allocated resources within it.
What is PyConfig.filesystem_encoding? đIn essence, PyConfig.filesystem_encoding is a configuration setting within Python that defines how file names are encoded and decoded. Think of it as a translator that ensures Python correctly interprets file names, regardless of the operating system itâs running on.
Why is this Important? đImagine youâre in a multi-lingual conference, and you donât understand the language being spoken. Youâd need a translator to ensure you comprehend all the information correctly.
What is PyFunction_GetClosure? đPyFunction_GetClosure is a function from the Python C API that allows you to access the closure of a Python function. If youâre scratching your head wondering what a âclosureâ is, donât worry. Letâs unpack it with a simple metaphor.
Think of a closure as a backpack. If you are a scout preparing for a hiking trip, you pack your backpack with essential items you may need laterâlike food, a map, and a first-aid kit.
What is PyImport_GetModule? đPyImport_GetModule is a function from Pythonâs C API - the under-the-hood C functions that Python is built upon. In essence, this function allows you to access Python modules that have already been imported, from a C extension module.
Imagine you are in a library. Youâve gathered a collection of books around you that youâre referring to (these are your imported Python modules). PyImport_GetModule is like a librarian who can fetch a book youâve already started reading and placed on your table.
What is PyNumberMethods.nb_divmod? đImagine PyNumberMethods.nb_divmod as a specialized worker in Pythonâs number handling factory. This worker is specifically in charge of the divmod() function, which, if youâve ever done arithmetic, is your go-to function for division and remainder operations.
In simpler terms, PyNumberMethods.nb_divmod is a function pointer within a C-struct that Python uses internally to define how division and modulo operations (nb_divmod) are performed on a custom object.
How is nb_divmod Used?
What is PyNumberMethods.nb_inplace_remainder? đIn simple terms, PyNumberMethods.nb_inplace_remainder is an internal method used by Python to perform in-place remainder operations. You might know this operation by its more common symbol, %=. Imagine you have two numbers, a and b, and you want to modify a to hold the remainder of dividing a by b. Thatâs exactly what a %= b does. The nb_inplace_remainder is the magic behind this operation.
How Itâs Used đWhile you may never directly interact with nb_inplace_remainder as a Python beginner (or even intermediate coder), understanding it gives you insight into how Python handles arithmetic operations under the hood.
What is PyCodec_IncrementalDecoder? đThink of PyCodec_IncrementalDecoder as a translator in the middle of a diplomatic meeting, continuously converting chunks of undecipherable language (encoded data) to something you can understand (decoded data). Unlike typical decoders that handle entire blocks of data at once, incremental decoders can handle data bit by bit. This can be particularly useful when dealing with streaming data, such as reading from a live network feed or processing large files that donât fit into memory.
What is PyDict_Values? đIn Python, dictionaries are like magical treasure chests, where each key is a unique identifier, and each value is the treasure associated with that key. The function PyDict_Values is a way to open up these treasure chests and look at all the treasures (values) inside, without worrying about the keys.
A Quick Overview of Python Dictionaries đBefore we delve into PyDict_Values, letâs briefly recap Python dictionaries:
treasure_chest = { "gold": 50, "silver": 100, "diamond": 5 } In our little treasure chest dictionary:
What is PyConfig.argv? đPicture attending a concert. You hand your ticket at the entrance, which confirms your reservation and seats you accordingly. Similarly, PyConfig.argv acts as the virtual ticket checker for your Python script. Itâs a list that stores the command-line arguments passed to your script. These are the extra pieces of information you might want to provide when running your program, making it more dynamic and versatile.
How is PyConfig.
What is PyFloat_FromDouble? đIn simple terms, PyFloat_FromDouble is a Python C API function that converts a C double (a type of floating-point number) into a Python float object. Think of it as a translating dictionary that converts one language (C double) into another (Python float). Why do we need this? Well, Python is written in C, and sometimes, when youâre writing extensions or interfacing Python with C code, youâll need to convert between these types.
What is PyNumberMethods.nb_inplace_add? đAt the core of Pythonâs numerical operations lies the PyNumberMethods structure, which defines a suite of numeric operations for Python objects. Imagine PyNumberMethods as a grand toolkit for your numeric data types. Among these tools is nb_inplace_add, which handles in-place addition.
In simpler terms, nb_inplace_add is what allows the magic of the += operator to happen. Instead of creating a new object to store the result of adding two numbers, nb_inplace_add modifies the existing object directly, if possible.
What is PyContextVar_Reset? đImagine you have a magical whiteboard where you can scribble notes. Now, letâs say you want to temporarily write something down and then erase it, restoring the original notes exactly as they were before. PyContextVar_Reset is like that eraser for your context variables in Python.
Pythonâs contextvars module, introduced in Python 3.7, provides support for context-local storageâessentially, variables that are unique to specific contexts. But what happens when you want to reset a context variable to its default state or restore a previous state?
What is PyMapping_Values? đImagine you have a magic dictionary. This dictionary not only holds words and their meanings but can also produce a list of all the meanings without you explicitly asking for the words. This magical functionality is similar to what PyMapping_Values does in the Python C API realm.
In Python, we often use mappings like dictionaries (dict). The C API provides various functions to interact with these mappings. PyMapping_Values is such a function.
What is PyLong_FromString? đThink of PyLong_FromString as the gatekeeper for turning string representations of numbers into actual Python integers. Itâs a function in Pythonâs C API that takes a string (with digits or even a little mathematical flair like â+â or â-â) and converts it into a PyLongObjectâPythonâs way of handling long integers.
How is PyLong_FromString Used? đYou might not use PyLong_FromString directly in your everyday Python scripts, but itâs good to know itâs there, especially if you ever dive into writing Python extensions in C.
What is PyMapping_HasKey? đThink of PyMapping_HasKey as a vigilant security guard in a Pythonic fortress made of data structures (mostly dictionaries). This guardâs job is simple yet crucial: They check if a specific key exists in a mapping object (like a dictionary) and tell you the result.
In technical terms, PyMapping_HasKey is a C API function provided by Pythonâs C API. It checks whether a given key exists in a mapping object, which is typically a dictionary.
What is PyCell_SET? đImagine you have a magic box, and this box can hold a single valuable item. You can put something in the box, inspect the item inside, or even replace it with something else. This magic box in Python terms is known as a âcell,â and PyCell_SET is the function that allows you to place or replace items in this box.
In technical terms, PyCell_SET is a function used in Pythonâs C-API that sets or updates the value contained in a cell object.
What is PyConfig.dev_mode? đImagine you have a personal assistant who behaves impeccably during a formal event but lets loose at home, revealing their true personality. Thatâs PyConfig.dev_mode in a nutshell. Itâs a configurable mode that helps you catch errors and inefficiencies in a development environment without the formal restraints imposed during production.
In Python, PyConfig.dev_mode is part of the PyConfig structure in the Python C API. It fundamentally toggles the interpreter to operate in a âdevelopment modeâ, which offers more stringent checks, helpful warnings, and generally more information about the state of your program.
What is PyFrame_GetLocals? đIn simple terms, PyFrame_GetLocals is a function in Pythonâs C-API, primarily utilized by developers working on Python internals or extending Python with C/C++. This function retrieves the local variables from a given frame object â imagine it as sneaking a peek into a magicianâs hat to see what tricks (local variables) are hidden inside.
How is PyFrame_GetLocals Used? đFor most Python developers, you might not directly use PyFrame_GetLocals, but understanding its role can deepen your comprehension of Pythonâs inner workings.
What is PyImport_ImportFrozenModule? đImagine you have a library of video games, and you decide to lock some of them in a vault for safekeeping. You can access and play these games later without worrying about them being altered or lost. In this metaphor, the video games are Python modules, and the vault represents the freezing process.
PyImport_ImportFrozenModule is a function in the Python C API that allows us to access these âvaultedâ or âfrozenâ modules.
What Exactly is PyMapping_Items? đImagine you have a toolbox. This toolbox is your dictionary, and the tools inside it are the key-value pairs. PyMapping_Items is the tech wizard that opens up this toolbox, showing you all the tools (or items) inside it.
In more technical terms, PyMapping_Items is a C-level function in Pythonâs C API that retrieves all items (key-value pairs) from a mapping object like a dictionary. Essentially, it gives you a list of tuples where each tuple is a key-value pair from the dictionary.
What is PyCoro_Type? đWhile this sounds like something out of a science fiction novel, PyCoro_Type is actually a type object in Python that represents coroutines. To put it simply, itâs a special Python type that backs the magic which allows coroutines to function.
Imagine if coroutines were vehicles. If coroutines were Teslas, then PyCoro_Type would be the electric engine driving these sleek machines. While many use the car without knowing the inner workings of the engine, understanding PyCoro_Type gives you that extra edge.
What is PyBytes_ConcatAndDel? đIn essence, PyBytes_ConcatAndDel is a function used in the Python/C API that performs two main actions in one go:
Concatenates two byte objects. Deletes the second byte object after itâs been concatenated. Think of it as a sophisticated bakery machine that not only glues two halves of a cookie together but also sweeps away the crumbs left behind.
Hereâs a closer look at its signature:
void PyBytes_ConcatAndDel(PyObject **pbytes, PyObject *newpart); Letâs break down the parameters:
What is PyComplex_Type? đIn Python, complex numbers are numbers composed of a real part and an imaginary part. They look like this: a + bj. Here, a is the real part, and b is the imaginary part. PyComplex_Type is the internal designation within the Python/C API that is used to represent these complex numbers.
When youâre working with complex numbers directly in your Python code, youâll mostly deal with the complex class, which is Pythonâs high-level abstraction.
What Is PyImport_ImportFrozenModuleObject? đTo simplify, PyImport_ImportFrozenModuleObject is a function in the Python C API that allows you to import modules that have been âfrozen.â When we say a module is âfrozen,â we mean it has been converted into a static C object code that can be embedded directly into a Python interpreter. Think of it as freeze-drying your favorite snack so you can carry it wherever you go, instantly ready to consume without needing to prepare it again.
What is PyMem_Free? đPyMem_Free is a part of Pythonâs C-API, a toolkit for writing Python extensions in C. Essentially, PyMem_Free helps to free up memory that was previously allocated. If you think of memory as that whiteboard, then PyMem_Free is the eraser!
Hereâs the technical definition: PyMem_Free(void *p) frees the memory block pointed to by p, which must have been returned by a previous call to PyMem_Malloc, PyMem_Realloc, or corresponding functions in the PyMem family for managing memory.
What is PyByteArray_Size? đImagine you have a treasure chest filled with gold coins, and you want to know exactly how many coins are in there. In Python terms, when you have a byte array (think of it as your treasure chest filled with bytes), PyByteArray_Size is the function that tells you the count of bytes (coins) in your byte array (treasure chest).
Hereâs the official description: PyByteArray_Size returns the size of the bytearray object provided.
Whatâs the Deal with PyFrame_GetBack? đPyFrame_GetBack is a function used internally by Python to manage framesâspecifically, it helps to retrieve the previous, or âback,â frame in the call stack. To put it simply, Python programs are composed of a series of function calls. These calls are stacked on top of each other in whatâs called a call stack. Each function call creates a frame, and when a function calls another function, a new frame is added to the stack.
What is PyDateTime_TIME_GET_MICROSECOND? đPyDateTime_TIME_GET_MICROSECOND is a macro provided by the Python C API that retrieves the microseconds component from a datetime.time object. Essentially, itâs like having a super-fine-tuned watch that can tell you exactly what the time is down to the millionth of a second. This macro is particularly useful when youâre working with time objects and need to extract thrillingly precise details about a specific moment.
How is it Used?
What is PyFile_WriteString? đAt its core, PyFile_WriteString is a function that allows you to write a string to a file object in Python. Imagine opening a diary, grabbing a pen, and jotting down a few lines. In the computational world, PyFile_WriteString is the pen that helps your Python code âwriteâ into a file.
Hereâs the technical description: PyFile_WriteString is a C function provided by Pythonâs C-API for internal use. It sends a string to a specific file pointer.