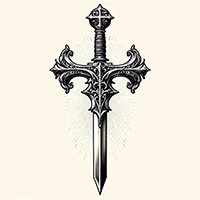
WE SHALL NEVER SURRENDER
What is PyImport_ImportModuleNoBlock? đPyImport_ImportModuleNoBlock is a function in Pythonâs C API that allows you to import a module without blocking, meaning it avoids waiting for potential problems like deadlocks when importing modules. This can be particularly useful when dealing with multi-threaded applications.
How to Use It đThis function isnât something youâd commonly use directly in typical Python programming. Instead, itâs a tool that comes into play when extending Python with C, or when youâre embedding Python into a C application.
What is PyInstanceMethod_Type? đTo put it simply, PyInstanceMethod_Type is a custom type provided by Pythonâs C API. Think of it like a specific toolkit designed to handle bound instance methods in Python. When you bind a method to an instance of a class, youâre essentially tacking on an operation to that instance. Internally, Python uses PyInstanceMethod_Type to manage this, creating a seamless experience for us developers!
How is it Used? đOkay, so how do you actually use this?
What is PyList_SetItem? đThink of PyList_SetItem as a tool in Pythonâs C API that lets you modify items in a Python list directly from C code. Yes, itâs that special wrench that you wished you had when playing with Python toys on a C-level playground.
How Does PyList_SetItem Work? đBefore diving into the mechanics, letâs lay down some context. Python lists are mutable, meaning you can change the items inside. In C, Python lists are represented by the PyListObject type, and PyList_SetItem provides a way to alter these lists.
What is PyLong_AsDouble? đIn the vast ecosystem of Pythonâs C API, PyLong_AsDouble is a function that takes a Python integer (of type PyLongObject) and converts it to a C double (floating-point number). Imagine Python integers as hefty dictionaries brimming with data. What PyLong_AsDouble does is akin to plucking out the essence from one of these dictionaries and presenting it in a smaller, more digestible form â a floating-point number.
How to Use PyLong_AsDouble đWhen developing Python extensions in C or C++ or embedding Python within another C application, you might need to switch between Python and C data types seamlessly.
What is PyLong_AsLong? đImagine you have a treasure chest filled with precious integers, but itâs locked inside a complex vault called Python objects. If you want to extract a simple integer (a 64-bit signed long) from this vault, you can use PyLong_AsLong. Think of PyLong_AsLong as the key to that treasure chest.
In technical terms, PyLong_AsLong is a part of the Python C API. Itâs used to convert a Python object (specifically a Python integer object, like those created using the int type) into a C long.
What is PyLong_AsUnsignedLongLongMask? đImagine PyLong_AsUnsignedLongLongMask as a magical sieve in the kitchen of Pythonâs C API. Just as a sieve separates fine flour from coarse chunks, this function helps you extract usable bits from Python integers. Specifically, it converts a Python object into an unsigned long long integer, but with a twist: it uses a bitmask to ensure the value fits within the bounds of an unsigned long long.
How to Use It?
What Is PyMapping_Check? đImagine youâre at a party and thereâs a bouncer by the door. This bouncerâs job is to check your ID to see if you are of legal age to enter. In the world of Python, PyMapping_Check acts like a bouncer for Python objects, determining if they can be considered as mappings (think dictionaries). In simpler terms, it checks whether an object behaves like a dictionary or another mappings type.
What is PyMappingMethods.mp_length? đPicture PyMappingMethods as a blueprint used to define behaviors for Pythonâs mapping objects (think dictionaries). Within this blueprint, mp_length is a method designed to measure the size of the mapping objectâessentially, itâs your measuring tape.
In technical terms, mp_length is a function pointer that returns the number of items in a mapping object. Itâs part of the PyMappingMethods structure, which provides a way to implement the mapping protocols (__getitem__, __setitem__, __delitem__) for Python objects.
What is PyMarshal_WriteObjectToFile? đIn the simplest terms, PyMarshal_WriteObjectToFile is a function that allows you to serialize a Python object and write it directly to a file. Serialization (or âmarshallingâ) is the process of converting an object into a format that can be easily stored or transmitted and then reconstructed later. Think of it as putting an object into a time capsule so that it can be resurrected in the exact same state sometime in the future.
What is PyMarshal_WriteObjectToString? đPicture this: youâve spent hours concocting a marvelous Python objectâa complex dictionary, a multi-level list, you name it. Now, you need to save it somewhere, say to a file, or send it over a network to another program. The crux is, how do you translate this living, breathing Python object into a format thatâs easily transferable or storable?
This is where PyMarshal_WriteObjectToString comes into play. Itâs like shrinking a massive painting down to fit inside a tiny postcard.
What is PyMem_Malloc? đIn simple terms, PyMem_Malloc is a function used within Pythonâs C API to allocate memory dynamically. Imagine your computerâs memory as a vast library. Each book represents a chunk of memory, while PyMem_Malloc acts like the librarian who helps you find exactly the book you need, reserved just for you for as long as you need it.
Why Use PyMem_Malloc? đWhile Python conveniently handles most memory management for you, certain situations in CPythonâs implementation require explicit memory allocation.
What is PyMemoryView_FromObject? đImagine youâre reading a book. This book has pages, and those pages contain text you want to read. PyMemoryView_FromObject is like a magical magnifying glass that lets you zoom into any part of this book, without having to rip any pages out. Specifically, it allows Python to create a memory view object from another object that supports the buffer protocol.
The Buffer Protocol đBefore we get into the nitty-gritty, letâs take a detour to understand the buffer protocol.
What is PyMethod_GET_FUNCTION? đImagine youâre an artist and your paintbrushâa methodâcan work wonders on a canvasâan object. Sometimes, you might want to inspect this brush to see what special things it can do. This is where PyMethod_GET_FUNCTION comes in. Itâs like a magnifying glass that allows us to peek into the innards of a method object and extract the underlying function.
In Pythonâs C API, a method object is actually an instance of the PyMethodObject structure.
What is PyMethod_GET_SELF? đPyMethod_GET_SELF is a C API function used primarily in Pythonâs C internals. Its primary role is to retrieve the self object from a bound method. In simpler terms, it helps us access the instance on which a method is bound.
To put it in a metaphor: imagine a method bound to a class instance is like a carrying bag attached to your shoulder. The PyMethod_GET_SELF function is the hand that reaches inside that bag to pull out your water bottle (the instance in this analogy).
What is PyModule_Check? đThink of PyModule_Check as a bouncer at a nightclub, deciding who gets in and who doesnât. Just as the bouncer checks IDs to determine if someone is old enough to enter, PyModule_Check scrutinizes objects to verify if they are Python modules.
Why Should You Care? đIf youâre just starting with Python, you might wonder why youâd ever need to know about this function. In practice, PyModule_Check becomes important when you start delving into C extensions or working with the Python C API.
What is PyNumberMethods.nb_and? đIn Python, PyNumberMethods is a structure used in the Python C-API to define operations on numeric types. Basically, itâs like a blueprint for how numbers should behave when you perform various operations on them.
One of these operations is the bitwise AND, represented by nb_and. When you perform a bitwise AND operation (using the & operator) between two numbers, Python uses the nb_and slot to define what action should occur.
What is PyNumberMethods.nb_inplace_and? đBefore we dive in, itâs important to understand that Python is built in C, and many operations you perform in Python are defined in C structures. One such structure is PyNumberMethods, which contains function pointers for numeric operations for a given type.
nb_inplace_and is one of the function pointers in PyNumberMethods. It specifically handles the in-place bitwise AND operation (i.e., &=) for Python objects that support these operations.
What is PyNumberMethods.nb_remainder and How Does It Work? đHave you ever been divided and left wondering what remained? No, weâre not talking about a dramatic breakup. Weâre talking about the remainder in Pythonâs numerical operations, specifically via PyNumberMethods.nb_remainder. This little technical gem is crucial for understanding divisionâs leftovers in Python.
What is PyNumberMethods.nb_remainder? đThink of PyNumberMethods.nb_remainder as the superhero behind the scenes, making sure your calculations for remainders (or moduli) work seamlessly.
What is PyNumberMethods.nb_reserved? đAt its core, PyNumberMethods is a structure in Pythonâs C-API that binds together a suite of function pointers relevant to numeric operations. These function pointers allow Python objects to support various arithmetic operations, like addition, subtraction, multiplication, and division, among others.
Nested within this structure, almost hidden like a silent librarian in an old library, is nb_reserved. This nb_reserved attribute is basically a placeholder, unused by Python. It doesnât participate directly in any functionality related to numeric operations.
What on Earth is PyErr_SetFromWindowsErrWithFilename? đImagine youâre sailing the seas of Python programming, and you suddenly hit an iceberg - an error. In the expansive ocean of code, error handling is your lifeboat. PyErr_SetFromWindowsErrWithFilename is one such critical feature that helps manage errors gracefully, especially when youâre navigating the tricky waters of Windows-specific issues.
In non-metaphorical terms, PyErr_SetFromWindowsErrWithFilename is a function within Pythonâs C API used to handle Windows errors. It primarily sets a Python exception based on the current Windows error code, and it can even tie this exception to a specific filename.
What on Earth is PyImport_ExecCodeModule? đImagine you have a treasure chest (your Python environment), and in this chest, you want to place treasures (Python modules) that you discover or create dynamically. PyImport_ExecCodeModule is like a magical incantation that helps you take raw code, turn it into a recognizable module, and store it in your Python environment.
What Does It Do? đPyImport_ExecCodeModule takes two main items:
A module name: Think of it like the label on your treasure chest saying what kind of treasure it holds.
What the Heck is PyEval_AcquireThread? đPyEval_AcquireThread is like the VIP pass at a concertâyou need this pass to access the main stage. In Pythonâs terminology, it allows a thread in the C code to take control of the Python Global Interpreter Lock (GIL).
The GIL: Pythonâs Master Gatekeeper đLetâs back up a bit and talk about the GIL. Imagine Python as a bustling coffee shop, and the GIL is the key to the cash register.
What the Heck is PyInstanceMethod_Check()? đImagine youâve got a Python object, and youâre like, âYo, Python! Is this an instance method?â You canât just ask it straight-up. You need a special tool to figure it out. Enter PyInstanceMethod_Check(), our trusty Sherlock Holmes in the Python C API.
The Basics đPyInstanceMethod_Check() is a C function that checks if a given object is an instance method. In simpler terms, itâs like checking if that mysterious figure in your living room is actually your pet cat or just a very confused raccoon.
Whatâs PyCell_New? đImagine youâre at a family BBQ, and youâre tasked with holding someoneâs drink while they get more food. Well, PyCell_New is sort of like that. In Python, it lets you create a âcell,â which can contain a single valueâthink of it as a specialized container or, more technically, a box.
Cells are particularly important in the context of Python closures, where you need to capture and store variables from enclosing scopes.
What on Earth is a PyCell? đBefore we tackle PyCell_GET, letâs understand what a PyCell is. Imagine a PyCell as a tiny, magical container that can hold a reference to a Python object. This container is particularly used in the context of closures and nested functions.
Picture a PyCell as a special safety deposit box in a bank. Only certain qualified personnel (nested functions) can access this box to retrieve or check the valuables (variables) stored inside.
What is pty.openpty()? đThink of pty.openpty() as your personal secret agent for creating a pair of file descriptors. These are no ordinary file descriptorsâtheyâre special ones that simulate a terminal. This pair consists of a master and a slave. The master is like the all-seeing eye, while the slave does the dirty work, pretending to be a regular terminal.
How to Use pty.openpty() đUsing pty.openpty() is as simple as convincing your cat to knock over a glass of waterâpretty straightforward once you know how to do it.
Whatâs pty.spawn Anyway? đImagine youâre a wizard and you want to control another wizard (a program) in your terminal. pty.spawn is your magical spell! It allows you to start another program and control its input and output from within your Python script. Think of it as being the puppet master of terminal programs.
Arguments đ argv: This is like the address of the wizard you want to control. Itâs a list where the first item is the command (the wizardâs name) and the rest are the arguments (the wizardâs instructions).
What is pty.fork()? đpty.fork() is a method that creates a new process (think of it as a clone of your current program) and gives it a pseudo-terminal. Imagine youâre on stage, and suddenly, a clone of you pops up with its own microphone. Thatâs pty.fork() for you!
Why Should You Care? đWell, if youâre into writing Python programs that need to interact with other terminal-based programs, pty.fork() is your new best friend.
tty.setraw() is a function from the tty module in Python that is used to put the terminal into raw mode. In raw mode, the input is made available to the program immediately, without any preprocessing, and special characters such as interrupt signals are not processed by the terminal driver. This mode is useful for applications that require complete control over input handling, such as text editors or terminal-based games.
What does tty.
tty.cfmakecbreak() is a function from the tty module in Python that is used to put the terminal into cbreak mode. This mode is a type of raw mode where the input characters are made available to the program immediately without requiring the Enter key to be pressed, but unlike full raw mode, special characters like interrupt signals are still processed by the terminal driver.
What does tty.cfmakecbreak() do? đThe tty.cfmakecbreak() function modifies a terminalâs settings to switch it into cbreak mode.