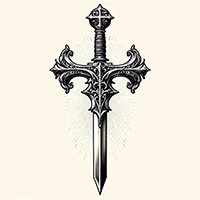
WE SHALL NEVER SURRENDER
What is PyLong_AsLongLong? đTo put it simply, PyLong_AsLongLong is a function used in Pythonâs C extension module to convert a Python integer object to a C long long type. If youâre scratching your head right now, donât worry! Letâs break it down.
Python and C: The Best Buddies đPython is a high-level, dynamically-typed language, and C is a low-level, statically-typed language. Sometimes, when writing C extensions for Python, youâll need to convert Python types to C types and vice versa.
What Exactly is PyFloat_Pack2? đImagine you have a suitcase and youâre going on a trip. You want to pack all your essentials but in the most compact way possible. Similarly, PyFloat_Pack2 is Pythonâs tool for packing floating-point numbers into a smaller, more efficient format. Itâs like vacuum-sealing your clothes to save space in your suitcase.
Specifically, PyFloat_Pack2 packs a floating-point number into a 16-bit binary representation. This is particularly useful for saving memory when precision isnât the utmost priority.
What is PyFloat_Unpack2? đAt its core, PyFloat_Unpack2 is a function used in Pythonâs C API that converts a two-byte (16-bit) binary representation into a Python float object. As the name suggests, it âunpacksâ data packed in a binary format into a floating-point number. This function is particularly useful for reading and processing binary data formats where numbers are stored in a compact form, like in networking protocols, file formats, or even hardware interfacing.
What is PyFloat_Unpack8? đImagine a packed suitcase by a meticulous travelerâneatly organized and compressed to optimize space. PyFloat_Unpack8 is like the adept hand that unpacks this suitcase, laying out everything neatly so you can see the individual items (or in our case, the floating-point numbers). PyFloat_Unpack8 is a function in Pythonâs C API that unpacks an 8-byte object into a double-precision floating-point number.
How is PyFloat_Unpack8 Used? đContext: The C Underbelly đIn Python, the float type represents double-precision floating-point numbers.
What is PyBytes_Check? đIn the simplest terms, PyBytes_Check is a function in the Python C API that checks whether a given object is a bytes object. Think of it like a bouncer at an exclusive club. The bouncerâs job is straightforward: to decide whether or not you fit the entry criteria. In our case, the âclubâ is the category of bytes objects, and PyBytes_Check is the bouncer checking the âIDâ of the object.
What is PyContext_Copy? đAt its core, PyContext_Copy is a function introduced in Python 3.7 as part of the contextvars module. If that sounds like a mouthful, donât worry! Think of it as a tool in Pythonâs toolbox designed to handle context management more efficiently.
To put it simply, PyContext_Copy is used to create a copy of the current context. Now, you might be wondering, what exactly is a âcontextâ? Good question.
What is PyEval_SetProfileAllThreads? đIn simple terms, PyEval_SetProfileAllThreads is a function that tells Python to track the execution details (like function calls and returns) across all threads in a Python program. If youâve ever worked with multi-threading in Python, youâd know it can get pretty wildâthink of it as juggling multiple balls at once. This function ensures that no ball is left unmonitored.
Diving Deeper: How Itâs Used đTo use PyEval_SetProfileAllThreads, you need to have C extensions or be comfortable embedding Python in C programs.
What is PyList_New? đPyList_New is a function in the Python C API that creates a new list object. For those unfamiliar, the Python C API is the toolkit that allows you to write C extensions for Python or dive deeper into Pythonâs internal workings. So, when youâre writing Python code, and you create a list, itâs PyList_New thatâs setting up the groundwork at the C level.
How is PyList_New Used? đImagine youâre a Python developer working within the C API to build a faster, more efficient module.
What is PyMem_Del? đImagine youâve thrown a huge party in your home. Now that the guests are gone, someone has to clean up the mess. In the realm of Python, when your program has finished using a block of dynamic memory, PyMem_Del is like that diligent cleaner who tidies everything up.
In tech speak, PyMem_Del is a function used to deallocate memory that was previously allocated by Pythonâs memory manager. This is crucial to prevent memory leaksâthose sneaky bugs that waste space by holding onto memory you no longer need.
What Is PyMemoryView_Check? đThink of PyMemoryView_Check as a bouncer at a swanky club, deciding who gets in and who doesnât. In this case, the âclubâ is the realm of memory views, and only genuine memory view objects get the thumbs up. This function checks if an object is a memoryview.
Definition đIn Python C-API, PyMemoryView_Check is a function that checks if a given object is a memory view object (or memoryview).
What is PyConfig.prefix? đImagine youâre the proud owner of a shiny new electric car. One of the crucial things you need is a charging station. Think of PyConfig.prefix like the home base where your electric car gets its juice. In simple terms, PyConfig.prefix is a variable within Pythonâs configuration that tells the Python interpreter where it can find its essential resources like libraries and other dependencies.
How is PyConfig.prefix Used? đImagine that every time you wanted to charge your car, you had to rebuild the charging station from scratch.
What is PyFrame_GetGenerator? đImagine youâre watching a magic show. Before the grand reveal, the magicianâs assistant signals a little behind-the-scenes to make sure everything goes smoothly. In the grand Python performance, PyFrame_GetGenerator could be considered that discreet assistant.
PyFrame_GetGenerator is a function deep in Pythonâs C API. Its main job is to retrieve the generator associated with a particular frame object. Now, frames are essentially execution records. Every time Python runs a piece of code, it creates a frame to keep track of where it is and what itâs doing.
What Exactly is PyFunction_GetModule? đThink of PyFunction_GetModule as a treasure map. Its primary duty is to take a function and point us directly to the X that marks its module. Essentially, this function retrieves the module object from which a given Python function was defined.
Why Would You Use PyFunction_GetModule? đImagine youâre in a giant library where every function is a book. You know the name of the book (the function), but you need to find out which section of the library (the module) itâs in.
What Is PyLong_CheckExact? đImagine youâre a teacher with a classroom full of students. Each student is holding a sign that identifies them. Some signs say âIâm a string!â while others say âIâm an integer!â When you question a student with, âAre you exactly an integer?â, only those with the pure integer sign should raise their hands. In Pythonâs world, PyLong_CheckExact is the function that asks this very question of its variables.
What is PyConfig.dump_refs? đThink of Python as an intricate web of interconnected pieces. Sometimes, we need to see how these pieces are connected to better understand, debug, or optimize our programs. Enter PyConfig.dump_refs, a tool that helps you examine the inner workings of Python by dumping referencesâa bit like turning on the lights in a dark room to see whatâs really going on.
In simple terms, dump_refs is a flag used to enable or disable the dumping of internal references created by Python during execution.
The Function in a Nutshell đImagine youâre hosting a grand party, and you have a VIP guest list. Not everyone can just waltz in; you need a meticulous door guard to check the list and handle gatecrashers with finesse. PyErr_SetImportErrorSubclass is like that vigilant door guard but in the realm of module imports. It enables the creation of a custom ImportError subclass when an import operation fails.
Why Is This Important?
What is PyCMethod_New? đAt its core, PyCMethod_New is a function in Pythonâs C API that helps create a new method object. If youâre building or extending Python in C, this function is a crucial tool in your toolkit. Think of it as the wizardâs wand that brings new functionalities (methods) to life in your Python objects.
How is it Used? đTo use PyCMethod_New, you need to be familiar with some C programming basics and have a good grasp of Pythonâs core mechanics.
What is PyEval_InitThreads? đIn the vast ecosystem of Python, PyEval_InitThreads is like the unsung hero of a bustling cityâoverlooked by many but indispensable to the few who rely on it. In technical terms, PyEval_InitThreads is a function within Pythonâs C API that initializes support for multi-threading in the CPython interpreter.
Why Should You Care? đOkay, so youâre not writing your own interpreter. Why does this function matter? Well, if you ever find yourself dealing with multithreading or extending Python with C/C++ libraries, understanding PyEval_InitThreads can save you from a world of pain.
What is PyMapping_DelItem? đImagine you have a magical box (your Python collection) where you store numerous items. Occasionally, you might want to remove a specific item from this magic box. In high-level Python, you might use del my_dict[key] for a dictionary or your_list.remove(item) for a list. However, in the world of Pythonâs C API, thereâs a special wizard called PyMapping_DelItem that helps you achieve this.
PyMapping_DelItem is a function provided by Pythonâs C API specifically designed to remove an item from a mapping object.
What is PyException_GetContext? đIn Python, exceptions are the bread and butter of error handling. However, sometimes, exceptions can be tangled up in a complex web where one exception is raised while another is already being handled. This is where PyException_GetContext steps in.
Simply put, PyException_GetContext is a C-API function that retrieves the context of an exception object. The context is another exception that was active (currently being handled) when the new one was raised.
What is PyException_GetTraceback? đImagine youâre trying to follow a breadcrumb trail through a forest. If you find yourself lost, those breadcrumbs can help you retrace your steps. In the world of Python programming, a traceback serves a similar purpose. It provides a trail you can follow to understand how your program ended up in its current stateâparticularly useful when debugging.
PyException_GetTraceback is like a special tool that Python provides to retrieve that breadcrumb trail.
What is PyConfig.show_ref_count? đImagine Python as a bustling city where objects are lively citizens. Now, keeping tabs on how often each citizen (object) is being used can be quite the task. Thatâs where PyConfig.show_ref_count comes in. Itâs like a digital census report for Python objects, showing you how many times each object is referenced.
import sys print(sys.gettotalrefcount()) In official terms, PyConfig.show_ref_count is a config setting in the CPython implementation that, when set to True, makes Python display the reference counts of all objects at shutdown.
What is PyContextVar_New? đImagine you are at a bustling cafĂ©, and the barista tracks each customerâs orders without mixing them up. Similarly, PyContextVar_New helps you create context variables that keep track of state specific to each execution context in a Python application, such as individual threads or asynchronous tasks. With it, you can create context-specific values that wonât interfere with each other, ensuring thread safety and proper execution flow.
How is PyContextVar_New Used?
What is PyDescr_IsData? đPython is like a big, friendly library with lots of cool books. But, sometimes, we need a librarian to tell us whether a certain book can be borrowed or is only for reading in the library. In this metaphor, PyDescr_IsData is like that librarian.
Specifically, PyDescr_IsData is a function from the CPython (the reference implementation of Python) that helps us identify whether an object (in this case, a descriptor) is a âdata descriptor.
What is PyDictProxy_New? đAt its core, PyDictProxy_New is a function in the Python C-API. This might already sound intimidating, but think of it as the gatekeeper that allows you to indirectly access and manage the hidden treasures buried within class namespaces â the attributes and methods.
An Analogy: The Library Catalogue đImagine a library with a vast collection of books (our Python class namespace). Now, you want to access these books but not directly manipulate them.
What is PyFrame_GetVar? đSimply put, PyFrame_GetVar is a function from Pythonâs C API used primarily for debugging and introspection. It allows programmers to access the local variables within a particular execution frame. Think of it as a special set of glasses that lets you see the variables and their values as the Python interpreter executes your code.
How is PyFrame_GetVar Used? đWhile PyFrame_GetVar isnât something you use in everyday Python coding, it becomes invaluable in more advanced scenarios like developing debugging tools or performance profiling.
What is PyFunction_GetAnnotations? đBefore we dive into the technical nitty-gritty, letâs get acquainted with function annotations in Python. Function annotations allow you to add arbitrary metadata to function arguments and return values. Think of them as little post-it notes that provide extra information about your functionâs parameters and return type.
For example:
def greet(name: str) -> str: return f"Hello, {name}!" Here, name: str and -> str are function annotations.
Now, onto PyFunction_GetAnnotations.
What is PyList_GET_ITEM, Anyway? đIn simple terms, PyList_GET_ITEM is a macro provided by the Python/C API that retrieves an item from a Python list at a given index. Think of it as a specific instruction telling Python, âHey, give me the item in this list over here at this exact position.â
Why Should You Care? đIf youâre working at the Python script level, you might not immediately need PyList_GET_ITEM. However, it becomes your best friend when you delve into lower-level manipulationsâespecially when writing C extensions for Python.
What is PyLong_Type? đImagine you are a carpenter. You need diverse tools to create different pieces of furniture. Similarly, in Python, whenever you see an integer like 5 or 123456789123456789, Python uses a special tool behind the curtains called PyLong_Type to handle these numbers. Essentially, PyLong_Type is Pythonâs internal representation for all integer objects.
How is PyLong_Type Used? đWhen you write Python code and create an integer, Python automatically takes care of assigning this integer to a PyLong_Type object.
What is PyMethodDef.ml_name? đThink of Pythonâs PyMethodDef.ml_name as the name tag at a networking event. Itâs a string that labels a function within a module. If youâve ever attended a party where everyone wore name tags to introduce themselves, youâll get this. The ml_name is basically the functionâs identifier, allowing Python to recognize and call it.
How is PyMethodDef.ml_name Used? đWithin the arcane realm of C extensions for Python, PyMethodDef is a structure that describes a single method of a module.