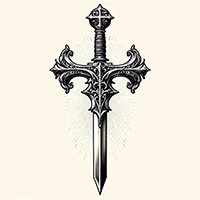
WE SHALL NEVER SURRENDER
What Does PyGC_Disable Do? đBefore we dive into PyGC_Disable, we need to talk about Pythonâs garbage collection (GC) system. Garbage collection is like a diligent janitor that cleans up unused memory so your Python program can run efficiently. This janitor runs periodically, looking for objects that are no longer in use and sweeping them away.
PyGC_Disable is a function that essentially tells this janitor to âtake a break.â When you call PyGC_Disable, it disables the automatic garbage collection.
What is PyImport_GetImporter? đPyImport_GetImporter is a lesser-known function in Pythonâs C API that retrieves the importer object for a given path string. Imagine you have a library of books (modules) spread across various shelves (directories). The PyImport_GetImporter function is like your intelligent library assistant. Give it a shelf location, and it tells you which mechanism (importer) to use to fetch the books (modules) from that shelf.
How to Use PyImport_GetImporter đBefore we delve into the inner workings, letâs look at a high-level view of how youâd typically use this function:
What is PyList_Check? đThink of PyList_Check as a vigilant gatekeeper whose sole job is to confirm the authenticity of a list. Essentially, itâs a function used in Pythonâs C API to check whether a given Python object is a list. In simpler terms, it answers the question: âHey, are you really a list?â
How is it Used? đBefore diving into how PyList_Check operates, itâs important to understand its syntax and basic usage:
What is PyLong_FromSize_t? đImagine you have a magic box that can turn any fruits (numbers, in this context) you throw at it into golden apples (Python integers). PyLong_FromSize_t is that magic box, except it works strictly with unsigned size_t values, which represent non-negative whole numbers and are commonly used to describe sizes and memory offsets in C.
In simple terms, PyLong_FromSize_t is a function that converts a value of type size_t in C to a Python integer object.
What is PyMarshal_ReadLongFromFile? đAt its core, PyMarshal_ReadLongFromFile belongs to the family of data marshaling functions in Python. Imagine marshaling as akin to packing your belongings for a trip: itâs all about serializing (packing) and deserializing (unpacking) data so it can be easily transported or stored.
So, What Does It Do? đPyMarshal_ReadLongFromFile is a function in Pythonâs internal C API. Its primary job is to read a long integer from a file that stores serialized data.
What is PyMem_RawMalloc? đImagine you are at a concert. The band needs a roadie to set up the stage, lights, and sound system. PyMem_RawMalloc is like that roadie but for Pythonâs memory management. Itâs a function from the Python C-API, specifically used to allocate raw memory. It is part of Pythonâs low-level memory management scheme, reserved for when Pythonâs built-in memory management simply isnât enough.
How Does It Work? đTo understand PyMem_RawMalloc, letâs dive into the mechanics of how memory allocation works.
What is PyMemberDef? đBefore we jump into flags, letâs first understand PyMemberDef. Imagine PyMemberDef as a blueprint for defining attributes on a Python object from within C code. It is used when extending Python with C or C++ to create new types. Think of it as telling Python, âHey, here are some attributes for this new type Iâm defining in the C realm.â
In Python, this is how you might normally define attributes on a class:
What is PyMemoryView_GetContiguous? đThink of PyMemoryView_GetContiguous as a custom tool in Pythonâs toolbox, designed for handling memory views in a more direct and frictionless manner. Specifically, it creates a new memory view object that ensures a contiguous (uninterrupted and sequential) memory layout.
In simpler terms, imagine you have a fragmented puzzle and you want it to be in a single, connected piece. Thatâs what PyMemoryView_GetContiguous does with your data.
How Is PyMemoryView_GetContiguous Used?
What is PyDateTime_DATE_GET_MICROSECOND? đImagine youâre dealing with time in your Python applications. You might need to know not just the hour and minute, but the exact microsecondâperhaps for logging events with high precision or synchronizing tasks down to the tiniest fraction of a second. This is where PyDateTime_DATE_GET_MICROSECOND swoops in like a superhero.
Technically speaking, PyDateTime_DATE_GET_MICROSECOND is a macro provided by Pythonâs C API. A macro in this context is just a shortcut to a piece of code; think of it like a pre-programmed task.
What is PyFile_SetOpenCodeHook? đAt its core, PyFile_SetOpenCodeHook is a function in Pythonâs C API that allows you to set a custom hook for opening .py files. Think of it as putting on a customized lens that Python will use whenever it needs to view or execute a Python file. This hook gives us the flexibility to alter how Python code is loaded, creating opportunities for advanced use-cases such as custom loaders or security enhancements.
What is PyCell_Check? đImagine youâre at a library. The library has many books (objects), and a librarian who keeps track of certain special books. Think of PyCell_Check as a function that helps the librarian identify if a specific book is one of these special ones. In Python terms, PyCell_Check is a built-in function intended to check if a given object is a cell.
What Are Cells in Python? đBefore delving deeper, letâs clarify what we mean by a âcellâ in Python.
What is PyConfig.pycache_prefix? đImagine you have a magical backpack in which you store tools needed for your adventure in the world of coding. PyConfig.pycache_prefix is like a special compartment in that backpack where Python keeps its compiled bytecode files. Bytecode files are the result of Python translating your human-readable code into something the Python interpreter understands more directly. Instead of creating these bytecode files wherever your scripts happen to be, you can tell Python to tidy them up into a specific directory using PyConfig.
What is PyFloat_AS_DOUBLE? đPyFloat_AS_DOUBLE is a macro provided by Pythonâs C API that extracts the underlying double value from a Python float object. Think of it as a backstage passâ while the audience (your high-level Python code) sees the float dancing on the stage, PyFloat_AS_DOUBLE lets you step behind the curtain to see its true form as a C double.
How is PyFloat_AS_DOUBLE Used? đPyFloat_AS_DOUBLE is primarily intended for use in C extensions or embedding Python in C/C++ programs.
What on Earth is PyMarshal_ReadObjectFromString? đImagine you have a treasure map, but instead of leading you to gold and jewels, it leads you to some Python objects stored as strings. The PyMarshal_ReadObjectFromString function is your pirate decoder ringâit takes these encoded strings and translates them back into usable Python objects.
The marshal module, where PyMarshal_ReadObjectFromString springs from, is all about reading and writing Python objects in a binary format. This comes in handy when you need to save Python objects to a file or send them over a network while preserving their state.
What Is PyDict_Keys? đFirst things first, PyDict_Keys is a built-in method in Python that belongs to the family of dictionary methods. For those unfamiliar, a dictionary in Python is a versatile collection type that allows you to store data in key-value pairs. Itâs like a real-world dictionary where you look up a word (the key) and get its definition (the value).
The PyDict_Keys method retrieves all the keys from a dictionary.
What Is PyFunction_SetClosure? đLetâs start with the basics. PyFunction_SetClosure is a function available in Pythonâs C APIâyep, the mysterious backstage where all the Python magic really happens. In a nutshell, PyFunction_SetClosure associates a set of free variables, known as closures, with a Python function. If youâre wondering what free variables are, donât panic. Weâre about to roll up our sleeves and get hands-on with some examples.
Why Should You Care About Closures?
What is PyInterpreterState_GetDict? đIn a way, Python is like a grand theater production. Youâve got the main cast, the support crew, and of course, the intricate script making everything run smoothly. PyInterpreterState_GetDict is part of the support crew that helps the grand production move efficiently behind the scenes. Specifically, it is a C API function in Python that allows you to access the interpreterâs internal state.
The Interpreter State đTo understand this function, you need a basic grasp of what the interpreter state is:
What is PyModule_GetDict? đAt its core, PyModule_GetDict is a function that retrieves the dictionary object associated with a module object. But what does that mean?
When you create a module in Python, under the hood, itâs essentially an object that houses a collection of attributes. Think of it as a âcontainerâ holding various items, such as functions, classes, and variables - all neatly packed and referenced in whatâs called a moduleâs dictionary (or __dict__).
What is PyNumberMethods.nb_matrix_multiply? đSimply put, PyNumberMethods.nb_matrix_multiply is a hook for handling matrix multiplication in Python. When you use the @ operator to multiply two matrices, Python uses the PyNumberMethods.nb_matrix_multiply if the objects involved support this operation.
Why Matrix Multiplication Matters đImagine youâre building a skyscraper of data operations. Matrix multiplication is like the blueprint calculations, crucial for everything to stand up. Whether youâre dabbling in machine learning, graphics, or just heavy numerical computations, understanding matrix multiplication can save you from coding nightmares.
What is PyDateTime_TIME_GET_SECOND? đThink of PyDateTime_TIME_GET_SECOND as a sharp pair of tweezers in your programming toolkit. Its sole purpose is to precisely extract the second component from a datetime.time object. In essence, itâs a highly efficient and dedicated function geared towards retrieving one specific piece of temporal data.
In simpler terms, if your datetime.time object were a burger, PyDateTime_TIME_GET_SECOND is the utensil that picks out just the picklesâthe secondsâfrom the entire melange of ingredients making up your delicious concoction of time.
Whatâs the GIL Anyway?
Before we dive into PyGILState_Release, letâs brush up on the GIL. Think of the GIL as a super-strict librarian in the Python world. This librarian allows only one âthreadâ (think of it as a person) in the library (your CPU) at a time. While this may seem like a bottleneck, the GIL ensures that the Python interpreter remains predictable and free from data corruption in multi-threaded programs.
What in the World is PyDateTime_TIME_GET_TZINFO? đLetâs start at the top. PyDateTime_TIME_GET_TZINFO is a macro function that comes from the Python C API, specifically utilized for working with date and time objects. To put it plainly, imagine it as a magic key that allows you to unlock the time zone information embedded in a Python time object.
In Pythonâs datetime module, a time object keeps track of time down to the microsecond but doesnât handle dates.
What Exactly is PyLong_Check? đImagine you have a party, and you want to ensure only invited guests enter. In our analogy, these guests are integers, and PyLong_Check is the bouncer at the door. This function, found in the C-API of Python, checks whether an object is a Python integer (specifically, a PyLongObject).
In technical terms, PyLong_Check is a macro defined in the Python C-API that verifies if a given object is an instance of the int type.
What Exactly Is PyBytes_AS_STRING? đImagine you have a magical box that can transform complicated data into simple, readable text. Thatâs what PyBytes_AS_STRING does in the world of Python. Specifically, it is a C API function that converts a Python bytes object into a C-style string (a plain array of characters).
Why Youâd Use PyBytes_AS_STRING đYou might wonder why this matters. If youâve ever worked with Python bytes objects, you know theyâre essential for handling binary data like images, audio files, or any data that requires precise binary formatting.
What Are Coroutines? đBefore we dive into PyCoro_New, letâs talk coroutines. Imagine a chef in a bustling kitchen who can stop cooking at any time to taste a dish, chop vegetables, or stir a pot, seamlessly resuming their previous task without starting over. In programming, coroutines are similar â they allow functions to pause execution (yield) and resume (__next__), maintaining their state in the meanwhile. This is especially useful for asynchronous programming, where waiting is inevitable.
What is PyDescr_NewMember? đImagine youâre at a fancy dinner party, and youâre introduced to a less-known yet vital guest: âPyDescr_NewMember.â At first, you may be puzzled about their role at this gathering of Python features. Well, PyDescr_NewMember is a function used in Pythonâs C-API, which is the underlying technology that makes Python tick. Specifically, it helps in the creation of member descriptors for types in extension modules.
In simpler terms, itâs like a backstage crew member that ensures each actor (data member) knows their role in your Python class.
What is PyDict_SetItem? đIn simple terms, PyDict_SetItem is a function that adds or updates a key-value pair in a Python dictionary. Think of it as the diligent librarian of Pythonâs dictionaries, meticulously recording and updating entries in the grand ledger of data.
How is PyDict_SetItem Used? đBefore we delve into the details, itâs important to note that PyDict_SetItem is a part of the Python/C API. This means itâs typically used in C extensions or other situations where C code interacts with Python objects.
What is PyDict_Watch? đImagine youâre Sherlock Holmes and youâre given a mission to monitor changes in a Python dictionaryâadditions, deletions, and modifications alike. PyDict_Watch is your Watson, your trusty partner in this high-stakes game of dictionary surveillance. Essentially, itâs a function that allows you to hook into dictionary operations and react to changes in real-time.
How is PyDict_Watch Used? đNow, before you go diving headfirst into the code, itâs worth noting that PyDict_Watch is not part of the standard Python libraryâmeaning itâs more of a hidden gem for those who dig deep.
What Is a frozenset? đBefore diving into PyFrozenSet_Check, letâs quickly recap what a frozenset is. Imagine you have a giant bookshelf (your data collection). You have regular books (mutable sets) and some books encased in glass frames that canât be altered (immutable sets or frozensets). You can still read and admire these encased books, but you canât rearrange or change them.
In Python, frozenset is an immutable version of a set.
What is PyNumberMethods.nb_negative? đThink of PyNumberMethods.nb_negative as a magic wand in Pythonâs extensive toolkit. Specifically, itâs a part of the C-API that Python uses internally to handle unary operations on numbers. In plain English, this means itâs a function that deals with the operation when you want to negate a number, i.e., when you use the unary minus (-) operator.
Why Should You Care? đYou might be thinking, âWhy should I care about this low-level detail?