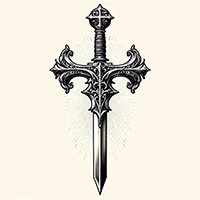
WE SHALL NEVER SURRENDER
What is PyNumberMethods.nb_subtract? đImagine Python as a kitchen full of powerful appliances. Youâve got mixers for strings, blenders for lists, and, of course, a myriad of tools for numbers. PyNumberMethods.nb_subtract is like your tried-and-true chefâs knife for subtracting numbers. This built-in function is part of Pythonâs C API, and it enables custom objects to handle subtraction operations.
How Does It Work? đWhen you type a simple subtraction in Python, like a - b, Python quietly checks if the objects a and b know how to subtract using their own dedicated subtraction method.
What is PyModuleDef.m_slots.m_reload? đIn Python, the PyModuleDef struct is a backbone for defining a moduleâa collection of methods and variables. This struct can have various slots to hold specific functions that serve different lifecycle needs of a module. One such slot is m_reload.
Imagine m_reload as a reset button on a gaming console. When you press it, the game doesnât start from scratch but rather reloads the current level. Similarly, m_reload is a function pointer that allows you to refresh or reset the state of a module when it is reloaded.
What is PyByteArray_Resize? đSimply put, PyByteArray_Resize is a function in the Python C API used to resize a bytearray object. If youâve dabbled with bytearrays in Python, youâd know they are mutable sequences of bytes. Think of a bytearray as a versatile Swiss Army knife for low-level data manipulation. Now imagine mid-task you realize your knife needs a few more toolsâor perhaps fewer. Thatâs where PyByteArray_Resize comes in. It allows you to adjust the size of your bytearray.
What is PyCallIter_Check? đAt its core, PyCallIter_Check is a function in Pythonâs C API. Itâs one of those behind-the-scenes operators that you wonât frequently encounter unless youâre delving deep into Pythonâs inner workings or working on extending Python with C modules.
In simple terms, PyCallIter_Check helps you determine if a given object is an instance of a call iterator. Wait, whatâs a call iterator? Picture an iterator: itâs that incredibly handy tool that allows you to loop over items in a collection (like a list or a dictionary) one at a time.
What is a PyCapsule? đImagine a PyCapsule as a tiny, secure container or âcapsuleâ in your Python environment. This capsule can hold a C pointer to data created in a C extension module. In plain English, a PyCapsule is like a special box where you can store delicate objects that require careful handling.
Why Use PyCapsules? đWhen working with Python extensions written in C, you often need a way to share data between Python and C without worrying about data corruption or misinterpretation.
What is PyCodec_IncrementalEncoder? đImagine that youâre translating a book. Instead of translating an entire chapter in one go, you decide to translate sentence by sentence. Each sentence gets its proper context and translation, which is efficient and convenient. The PyCodec_IncrementalEncoder is like your linguistic magic wand for computers, helping you transform small chunks of data from one encoding to another, incrementally.
Encoded correctly, data is the lifeblood of any application, whether itâs text, images, or videos.
What is PyCodec_Register? đBefore diving into the nitty-gritty, letâs break down what a codec is. A codec (short for coder-decoder) is a program that transforms data from one format to another. Think of it as a translator that lets your Python program speak multiple languages, whether itâs text, numbers, or complex data formats.
Now, PyCodec_Register is a function in Python that allows you to register a new codec. In simpler terms, itâs like teaching Python a new language.
What is PyConfig.faulthandler? đThe faulthandler module in Python is akin to having a spotlight in a dark room. It illuminates the specific point in your code where things go awry, making those hard-to-find bugs much easier to locate. When the faulthandler is enabled, it helps you capture low-level errors such as crashes by printing a traceback of where the error occurred. This can be a lifesaver when dealing with unpredictable behavior like segmentation faults, especially in more complex applications or when interfacing with C extensions.
What Is PyConfig.inspect? đImagine setting up a sophisticated coffee machine. There are various parts, buttons, and configurations you need to get just right for that perfect cup. In Python, PyConfig.inspect performs a similar roleâitâs part of the larger configuration framework that helps set up the Python runtime environment.
The PyConfig structure in Python, where PyConfig.inspect resides, is designed to handle numerous initialization settings. Specifically, PyConfig.inspect is a flag (boolean value) that determines whether Python should enter inspection mode during interactive sessions and when executing scripts.
What Is PyConfig.optimization_level? đThink of Python as a sophisticated vehicle where the engineâs performance can be tweaked for either fuel efficiency or speed. In this metaphor, PyConfig.optimization_level is like the gearbox that allows you to switch between different drive modesâeach offering a different balance of performance and resource consumption.
Specifically, PyConfig.optimization_level is a setting within Pythonâs configuration that controls how optimized the bytecode should be. Bytecode, if you will, is the native language Python speaks to execute your Python scripts effectively.
What Exactly is PyConfig.pythonpath_env? đThink of Pythonâs PyConfig.pythonpath_env as a postal service for Python modules. In more technical terms, itâs a configuration option that defines where Python looks for modules to import. Itâs a part of the PyConfig structure, which is employed to configure Python in an embedded contextâessentially when Python is run as part of another application.
How is PyConfig.pythonpath_env Used? đBefore diving into the gritty details, letâs consider this metaphor: Imagine youâve just moved to a new city, and youâd like to know where all the useful stores and services are located.
What is PyConfig.tracemalloc? đImagine you are trying to keep track of all the snacks youâve hidden around your house. Over time, you might forget what you stashed where, and your supply of goodies could start to dwindle without a clear understanding of where the snacks went. Similarly, Python programs can sometimes be forgetful about where they allocated memory, leading to memory leaks.
PyConfig.tracemalloc is like a meticulous notetaker who keeps a log of all memory allocations.
What is PyConfig.warnoptions? đThink of PyConfig.warnoptions as the control panel for Pythonâs warning system. Just as you use the control panel in your car to manage the air conditioning or radio settings, PyConfig.warnoptions allows you to customize how warnings are handled in your Python program. Itâs a part of the broader PyConfig structure introduced in Python 3.8 to configure the runtime environment before the Python interpreter starts executing your code.
What is PyContext_New()? đThe PyContext_New() function is a lesser-known but crucial part of Pythonâs underlying machinery. Essentially, it is a C API function responsible for creating a new context in Pythonâs implementation. If youâve never encountered it, thatâs because it operates deep within the bowels of Pythonâs execution environment, particularly in dealing with asynchronous programming.
How is PyContext_New() Used? đIn more practical terms, you wonât typically invoke PyContext_New() directly in your everyday Python scripting.
What is PyDateTime_DeltaType? đThe PyDateTime_DeltaType is an integral part of Pythonâs datetime module. It represents the difference (or âdeltaâ) between two dates, times, or datetime objects. This delta can be used to perform arithmetic operations on dates and times, allowing you to effectively add or subtract intervals, manipulate time data, and much more.
In plainer terms, imagine you have two dolls, one representing today and the other representing a future date.
What is PyDict_GetItemWithError? đImagine you have a magical box (a dictionary in our world). You want to find out if a particular item is inside without causing a commotion (or an error). This is where PyDict_GetItemWithError steps in. Itâs like a polite treasure map for our magical box, guiding us to the exact spot where our item might be, and if it isnât there, it gently lets us know there was an issue looking for it.
What is PyDict_SetDefault? đImagine youâve got a magical box (we call it a dictionary) that stores pairs of items. Each item in the box has a unique identifier, much like having unique names on luggage tags at an airport. Now, you often need to check whether a particular piece of luggage (key) is already in your magical box. If itâs not, youâd like to add a new piece of luggage with some default content (value).
What is PyErr_SetFromErrno? đIn Python, handling errors gracefully is crucial. PyErr_SetFromErrno is a part of the Python C API, and itâs used to set a Python exception based on the last system error. Imagine youâve just tried accessing a locked door, and the handle shakes but doesnât budge. PyErr_SetFromErrno is like the sign that pops up saying, âHey, you canât get in because this door is locked, and hereâs why.â
What Does PyErr_SetObject Do? đIn simple terms, PyErr_SetObject is a function in Pythonâs C-API that sets the error indicator to a specific exception. Think of it as a way for Python to say, âHey, something went wrong, and hereâs the reason why.â When you encounter a try...except statement in Python, the magic under the hood often involves PyErr_SetObject.
Hereâs a real-world analogy: imagine youâre a librarian and a visitor is looking for a book you donât have.
What is PyFunction_NewWithQualName? đAt its core, PyFunction_NewWithQualName is a function from Pythonâs C-API, which is used to create a new function object in Python. Think of it as the factory where Python functions are born. However, its specialty lies in giving the function a qualified name (hence the âQualNameâ in the name), which helps in identifying functions within the broader scope of classes and modules.
How is it Used? đFirstly, if youâre a beginner, you primarily interact with Python through its high-level syntax and libraries.
What is PyIndex_Check? đImagine youâre throwing a dinner party and you have a list of invited guests. You want to make sure that anyone who shows up at your door is indeed on the guest list before letting them in. In the Python world, PyIndex_Check is like the doorman of your party, ensuring that the types entering an indexing operation are valid.
In simpler terms, PyIndex_Check is a C API function that checks whether a given object can be used as an index in sequences like lists, tuples, or strings.
What is the GIL? đImagine youâre crafting a batch of cookiesâoodles of them. You have several workers (threads) ready to help. However, you only have one rolling pin (the GIL). Even though you have a bunch of eager bakers (your computerâs CPU cores), only one can use the rolling pin at any given moment. Consequently, the rest are idling or doing other prep work while waiting for their turn. This rolling pin is a metaphor for the Global Interpreter Lock (GIL) in Python.
What is PyLong_AsUnsignedLong? đSimply put, PyLong_AsUnsignedLong is a function provided by Pythonâs C API that allows you to convert a Python integer (which is an instance of int or long in Python 2, and just int in Python 3) to an unsigned long integer (a C data type). Think of it as a translator that bridges Pythonâs way of handling numbers with Câs way.
In Python, integers are handled with great flexibility, supporting arbitrarily large values.
What is PyMem_Calloc? đPyMem_Calloc is a function provided by the Python C API that specifically handles memory allocation with a twist. Unlike its sibling PyMem_Malloc, which just allocates memory, PyMem_Calloc goes the extra mile to zero-initialize the memory it allocates. Think of it as not just providing a new notebook but also erasing any previous scribbles, leaving you with crisp, blank pages.
How is It Used? đUsing PyMem_Calloc is fairly straightforward, but it involves a few steps.
What is PyMem_GetAllocator? đPyMem_GetAllocator is a function in Pythonâs C API (Application Programming Interface). Its primary role is to fetch the current memory allocator for the âRawâ domain. This function is essential for understanding and customizing the way Python manages memory at a low level.
Think of PyMem_GetAllocator as a concierge in a high-end hotel. It knows exactly who is handling what part of the memory, and you can ask it anytime to know how the allocation work is being managed.
What is PyModule_ExecDef? đImagine that Python and C are best friends working on a project. PyModule_ExecDef is like the translator facilitating their conversation. Specifically, itâs a C API function used to initialize and execute the definitions (defs) of a Python module from the C side.
How is PyModule_ExecDef Used? đWhen youâre writing a module in C to expose its functionalities to Python, you need to define your moduleâs methods and its specifications in terms of C structures.
What is PyNumberMethods.nb_float? đPyNumberMethods.nb_float is part of Pythonâs C-API, specifically designed for handling numeric operations in Pythonâs built-in and user-defined types. It plays a crucial role in converting your custom objects to floating-point numbers (or floats).
Imagine PyNumberMethods.nb_float as an interpreter who speaks the language of integers, strings, and other data types, and converts them to a universally understood âfloating-pointâ language when needed.
How is it Used? đWhen you attempt to convert an object to a float in your Python code using the built-in float() function, Python needs to know how to perform this conversion.
What is PyNumberMethods.nb_inplace_lshift? đIn simple terms, PyNumberMethods.nb_inplace_lshift is part of Pythonâs C-API, and it corresponds to the in-place left shift operation (<<=) for objects that support the number protocol. This might sound like a mouthful, so letâs break it down.
Imagine you have a number, say x, and you want to perform a left shift operation on it, like this:
x <<= 3 This operation shifts the binary representation of x to the left by 3 places.
What Exactly Is PyNumberMethods.nb_inplace_power? đImagine youâre at a construction site. You have a powerful jackhammer, but you also need a handheld drill for more delicate tasks. PyNumberMethods.nb_inplace_power is like that drillâsmall but mighty in its handling of in-place power operations.
In Python, this method pertains to the in-place exponentiation operation (**=), allowing objects to efficiently raise a number to a given power and directly store the result back in the original variable.
What is PyNumberMethods.nb_invert? đPyNumberMethods.nb_invert is a slot in the PyNumberMethods structure in Pythonâs C API. This slot is designed to handle the unary bitwise invert operation, which is represented by the ~ operator in Python.
Like Magic, But With Numbers đImagine you have a magical wand that can instantly transform positive numbers to their negative counterparts with a twist of a wrist and vice versa. Thatâs somewhat akin to what the ~ operator does at a binary level, flipping every bit in the number: zeros become ones and ones become zeros.