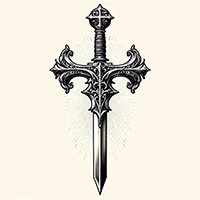
WE SHALL NEVER SURRENDER
What is PyLong_FromVoidPtr? đIn Python, integers are a piece of cake. You declare them, manipulate them, and Python handles all the dirty work behind the scenes. But when you step into the realm of C and Python interaction, things get a bit more⌠complicated.
PyLong_FromVoidPtr is a function from Pythonâs C API that converts a pointer (specifically, a void* pointer) into a Python integer (PyLong). Think of it like translating one language to another: it takes something from Câs world (a pointer) and makes it comprehensible in Pythonâs world (an integer).
What is PyMapping_DelItemString? đPyMapping_DelItemString is a function provided by Pythonâs C API, allowing C extensions to manipulate Python objects with mapping interfaces (like dictionaries) more efficiently. Think of it as a specialized tool in a carpenterâs kit, designed specifically for removing items from a dictionary.
In simple terms, PyMapping_DelItemString deletes a key-value pair from a dictionary when you are working within the C layer of Python.
How is PyMapping_DelItemString Used? đBefore we go further, letâs set the stage with a little context.
What is PyMapping_GetItemString? đPyMapping_GetItemString is a C function in the Python/C API that helps you retrieve an item from a mapping object, like a dictionary, using a key in the form of a C string. Think of it as a translator in a diplomatic mission: it helps the C language communicate effectively with Python objects.
Why Use PyMapping_GetItemString? đWhen writing C extensions for Python, you might need to manipulate Python objects directly from C code.
What is PyMapping_HasKeyString? đAt its core, PyMapping_HasKeyString is a function from the C-API of Python. This function is designed to check whether a specific key exists within a mapping object, such as a dictionary. The âstringâ in PyMapping_HasKeyString tells us that this function specifically looks for keys that are strings.
Imagine you have a treasure map (your dictionary) filled with clues (keys). PyMapping_HasKeyString is like a quick check to see if a particular clue (string key) is on the map without actually unrolling the entire thing.
What is PyMapping_Keys? đThink of PyMapping_Keys as a magical tool that allows you to open up a treasure chest â in this case, a dictionary â and see all the keys inside. In technical terms, PyMapping_Keys is a function provided by Pythonâs C-API that returns a list of all keys from a given Python mapping object, such as dictionaries.
How to Use PyMapping_Keys đThough itâs part of the C-API (typically used in C extensions rather than everyday Python coding), understanding it can give you more insight into how Python works under the hood.
What is PyMapping_SetItemString? đImagine you have a magical box (letâs call it a âmapping objectâ) that can store countless magical items (letâs call these âkey-value pairsâ). Now, PyMapping_SetItemString is like a friendly wizardâs spell that helps you place a new item or update an existing item in this magical box.
In technical terms, PyMapping_SetItemString is a function in the Python C API that allows you to set a value in a mapping object (like a dictionary) using a string as the key.
What is PyMapping_Size? đImagine you have a giant toolbox filled with different tools. Each tool is different but useful in its own way. In the world of Python, a âmappingâ is like that toolbox, where each tool is a key-value pair. The PyMapping_Size function helps you figure out how many tools (key-value pairs) are inside your toolbox (mapping).
How to Use PyMapping_Size đThink of PyMapping_Size as your assistant who, with a quick glance, tells you the exact number of tools you have.
What Is PyMappingMethods.mp_ass_subscript? đPyMappingMethods.mp_ass_subscript is part of a structure in Pythonâs C API that specifically manages the item assignment, deletion, and subscripting of your mappings (like dictionaries). In more human terms, itâs like the librarian (you) assigning books (values) to specific shelves (keys), replacing old books, or even removing books out of the shelf.
How Is It Used? đSince PyMappingMethods.mp_ass_subscript is part of the Python C extension, the everyday Python programmer wonât use it directly in their code.
What is PyMappingMethods.mp_subscript? đIn Python, objects like lists, dictionaries, and tuples can be interacted with using the subscript notation. Essentially, when you use brackets ([]) to access or assign values, youâre using subscript notation. For instance, when you do something like my_list[1] to get the second element in a list, youâre utilizing subscripting.
PyMappingMethods is a structure in Pythonâs C API that provides a set of function pointers for operations that can be performed on mapping objects (like dictionaries).
What is PyMarshal_ReadShortFromFile? đThink of PyMarshal_ReadShortFromFile as a specialized tool in the large toolbox that is Pythonâs C-APIâa set of interfaces that allow Python code to interact with C (the programming language, not the average grade). PyMarshal_ReadShortFromFile is specifically used to read a short integerâmeaning a small, whole numberâfrom a file.
How Does it Work? đAlright, hereâs where we roll up our sleeves. When we talk about âreading a short integer from a file,â what weâre really talking about is accessing data thatâs been serialized (converted into a format that can be stored).
What is PyMem_RawRealloc? đIn simple terms, PyMem_RawRealloc is a function used to resize a block of memory. Imagine youâre throwing a party and you initially set out a small table for snacks. As more guests arrive, you might find that the table is too smallâso, you bring in a bigger table to hold more snacks. In much the same way, PyMem_RawRealloc takes an existing block of memory and resizes it, either expanding or shrinking it based on your needs.
What is PyMem_Realloc? đImagine youâve hosted a party. Guests (data) keep arriving and, occasionally, you need more chairs (memory). Youâd rather not kick anyone out, but instead, add more chairs to accommodate everyone comfortably. PyMem_Realloc is your party planner that efficiently arranges additional chairs without causing chaos.
Simply put, PyMem_Realloc is a C API function used in Python to resize an already allocated memory block. Itâs particularly useful when the size of data you need to store changes dynamically.
What is PyMem_SetAllocator? đPython, like many other programming languages, uses a memory allocator to handle memory allocation and deallocation. Simply put, memory allocation is akin to organizing books on a shelf; you need to assign a particular space to each book (block of data). PyMem_SetAllocator allows advanced users to customize this process by setting their own memory allocator.
Imagine you have a librarian whoâs quite good at organizing. However, you believe you could do an even better job.
What is PyMember_GetOne? đIn essence, PyMember_GetOne is a function used internally within the Python/C API to retrieve the value of a member variable (attribute) from a Python object. This function is not something youâd use directly in everyday Python programming, but itâs vital when youâre working at the intersection of C and Python, particularly in extending Python with C or embedding Python in C applications.
How is PyMember_GetOne Used? đSince PyMember_GetOne is part of the lower-level Python/C API, you wonât typically see it in standard Python scripts.
What is PyMember_SetOne? đIn the land of Python, there are many tools Python professionals use for creating and managing objects. One such tool is the PyMember_SetOne function, which is part of the Python C-API. Think of PyMember_SetOne like the backstage crew in a magic show, ready to set the stage for the main illusions (your Python objects) to perform seamlessly.
Simply put, PyMember_SetOne is used to set a specific value to a specific member (attribute) of an object within a C extension module.
What is PyMemberDef? đBefore we jump straight into PyMemberDef.doc, letâs introduce the broader concept of PyMemberDef in Python. Think of PyMemberDef as a blueprint for defining members (attributes) of Python classes in C-extension modules. If Python were a house, the PyMemberDef struct would be like a detailed architectural plan for each room (class attribute).
Hereâs what a PyMemberDef struct looks like under the hood:
typedef struct PyMemberDef { const char *name; // The name of the member int type; // The type of the member: T_INT, T_OBJECT, etc.
Understanding PyMemberDef.name: The Unsung Hero of Pythonâs C API đImagine youâre at a party, and everyone is mingling aroundâthe members of our Python class are like the guests at this shindig. Some guests are a bit quiet and private, while others are more outgoing and ready to chat. Now, think about the PyMemberDef.name as the nametags each guest wearsâessential for identifying whoâs who at a glance.
Breaking Down PyMemberDef and Its Role đBefore we get to the nametag part, letâs talk about PyMemberDef.
What is PyMemberDef? đBefore we dissect PyMemberDef.type, letâs get acquainted with PyMemberDef. This structure is used when defining members of new types in Python extensions written in C. Essentially, itâs akin to declaring variables and their properties in a class.
Hereâs a simple analogy: Imagine PyMemberDef as a blueprint for a custom toy robot. You detail each part â its type, position, and properties â before manufacturing it. When you create a Python extension, PyMemberDef acts as this blueprint.
What Is PyMemoryView_FromBuffer? đThink of PyMemoryView as Pythonâs way of glancing at a window rather than copying the entire landscape painting. When dealing with large data buffers, creating a memory view allows you to interact directly with the buffer without the overhead of creating a new object. Itâs a lean, mean, memory-efficient machine.
Specifically, PyMemoryView_FromBuffer constructs a memory view object from a given buffer. This is essentially Pythonâs way of giving you a direct peek into an array, a bit like getting VIP backstage access to a concert instead of listening to it from the parking lot.
What is PyMemoryView_FromMemory? đImagine memory in a computer as a big library filled with books (data). If you want to read or modify some information, you need a way to find the right book and open it to the correct page. PyMemoryView_FromMemory is like a map that helps you locate these books and pages in the library, giving you direct access to the specific areas of memory you need to work with.
What is PyMemoryView_GET_BASE? đIn more technical terms, PyMemoryView_GET_BASE is a function provided by the Python C/API that returns the base object underlying a memoryview object. This base object is the original data from which the memoryview was created.
How to Use PyMemoryView_GET_BASE đBefore we run, letâs walk through a simple use-case. Say youâre dealing with a large dataset and you want to work with chunks of this data for efficiency. Memoryviews let you do this without copying the data.
What is PyMemoryView_GET_BUFFER? đLetâs start with the basics. PyMemoryView_GET_BUFFER is a function in Pythonâs C API. This might sound scary if youâre new to Python, mostly because Python is known for its simplicity. However, under the hood, a lot of C operations make the magic happen.
So, what does this mysterious function do? In simple terms, PyMemoryView_GET_BUFFER gives you direct access to the buffer interface of a Python object that supports it.
What is PyMethod_Check? đImagine you are organizing a library, and you have books and magazines that need to be sorted into the right sections. In Python, objects can be a bit like those books and magazinesâthey belong to different types and classes. The PyMethod_Check function is like a librarian who quickly checks if an item is specifically a bound method. Bound methods are instances of the method type that bind together a function and its associated class instance.
What Is PyMethod_Function? đLetâs start with the basics. The PyMethod_Function is a function in Pythonâs C API, which involves delving into Pythonâs core. Think of the C API as the workshop where Python constructs its features using robust, low-level tools.
So, whatâs the role of PyMethod_Function in this workshop? Simply put, it allows you to extract the underlying function object from a method object. Imagine you have a beautifully wrapped gift (the method), and PyMethod_Function is the super-sleuth who lets you peek inside and see the actual present (the function).
What is PyMethod_New? đPyMethod_New is a function in Pythonâs C API that creates a bound or unbound method object. This function essentially bridges the gap between a normal function and a method that is associated with a particular object or class. Think of it as a special glue that binds functions to their objects or classes.
When Do You Use PyMethod_New? đNow, you might be asking yourself, âWhen would I ever need to use PyMethod_New?
What is PyMethod_Self? đImagine youâve been handed the keys to a sleek new car, but instead of driving it, you want to understand what goes on under the hood when you press the accelerator. When weâre dealing with Python, PyMethod_Self is one of those internal gears that make object-oriented programming tick.
In essence, PyMethod_Self is a mechanism that allows you to access the object instance on which a method operates. When you call obj.
What is PyMethodDef.ml_doc? đPyMethodDef.ml_doc is a crucial component in Pythonâs C API (Application Programming Interface). In simple terms, itâs a way to attach documentation strings (docstrings) to methods in extension modules written in C. When you define a function in C to be used in Python, you can provide a human-readable description of what the function does, how it should be used, and any other pertinent details.
How Does It Work?
What is PyMethodDef? đBefore we delve into ml_flags, itâs essential to understand what PyMethodDef is. Think of PyMethodDef as a blueprint that describes a Python method when youâre working at the C level of Pythonâthis is where the magic of extending Python with C happens. It tells Python about your methodâs name, the function that implements it, the type of arguments it expects, and a docstring that describes what it does.
What is PyModule_AddObject? đImagine youâre curating an art gallery, and each module is a separate room filled with artworks (objects). PyModule_AddObject is like the curator who places a new piece of art into the correct gallery room. In technical terms, PyModule_AddObject is a C API function used to insert a new object into a module.
How to Use PyModule_AddObject đAlright, youâre the curator. How do you put that fabulous artwork (your object) into the right room (your module)?
What is PyModule_AddStringConstant? đThink of PyModule_AddStringConstant as a special helper that allows you to essentially âteachâ a Python module a new string constant. To be more precise, this function is used to add a new constant string to a moduleâs namespace. It serves a key role in extending Python by allowing C developers to embed new constants into their Python modules, helping to bridge the gap between the C language and Python.