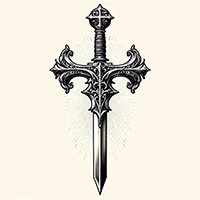
WE SHALL NEVER SURRENDER
What is PyErr_NoMemory? đPyErr_NoMemory is a function in Pythonâs C API thatâs used to indicate the program has run out of memory. Think of it as a red flag your program raises to signal, âHey, I canât go any further; I need more memoryâor better memory management!â
In Python, this corresponds to raising a MemoryError exception in your Python code. This is Pythonâs mechanism to let you know that it has run out of the required memory to complete an operation.
What is PyErr_NormalizeException? đPyErr_NormalizeException is a function from Pythonâs C APIâ the underlying machinery that powers Python. Think of it as the backstage crew ensuring the show runs smoothly, transforming the raw materials of an exception into a structured format that Python can handle and present to you, the developer, in a comprehensible way.
In simple terms, PyErr_NormalizeException takes the confusion out of exceptions by refining the error type, value, and traceback before passing these components back to the Python runtime for your handling.
What is PyErr_Print? đIn Python, PyErr_Print is your go-to function for dealing with exceptions when youâre extending Python with C. Think of it as your friendly translator between the error-heavy language of the Python interpreter and your human-understandable error messages.
Imagine youâre the director of a stage play. Unexpectedly, one of the actors (your Python code) forgets their lines (throws an exception). PyErr_Print acts like the prompter, amplifying the actorâs error loud and clear, so not only you but also the audience (other developers or users) can understand what went wrong.
What is PyErr_ResourceWarning? đIn simple terms, PyErr_ResourceWarning is like a vigilant watchman. Its job is to alert you when resources, such as files or network connections, are not being properly managed in your Python code. When these resources are not closed correctly, it can lead to potential memory leaks or other unintended behavior.
Why is it Important? đLetâs imagine for a moment that youâre a librarian. You have a finite number of books (resources).
What is PyErr_Restore? đIn Python, when an error occurs, an exception is raised. This is Pythonâs way of signaling that something went wrong. PyErr_Restore is a lower-level function in Pythonâs C API that helps manage these exceptions programmatically from C code. Essentially, it sets the information about the last error that occurred.
If youâve ever worked with Python exceptions using the try, except blocks, youâre familiar with catching errors when they happen.
What is PyErr_SetExcFromWindowsErr? đImagine youâre steering a ship, and you hit an unexpected icebergâthe Titanic moment. Youâd want to alert everyone immediately, right? In programming, particularly when interfacing with Windows-specific APIs, youâll occasionally hit an âicebergââerrors and exceptionsâthat require attention.
PyErr_SetExcFromWindowsErr is like raising the alarm bell in Python code that wraps Windows API calls. It sets a specific Python exception based on the last Windows error code encountered.
Breaking Down the Function đThe prototype for PyErr_SetExcFromWindowsErr looks like this:
What It Does đImagine youâre working on a Python project where you need to interface with Windows-specific features, like filesystem operations. You might encounter Windows-specific errors. The PyErr_SetExcFromWindowsErrWithFilename function comes to your rescue by creating a Python exception that corresponds to a Windows error code, and optionally, relates this error to a specific file.
Think of it as a translator that converts a Windows errorâletâs say a âFile Not Foundâ errorâinto a Python exception that your Python code can understand and handle properly.
What Does PyErr_SetExcFromWindowsErrWithFilenameObject Do? đSimply put, PyErr_SetExcFromWindowsErrWithFilenameObject is a function used within Python C extensions to raise a Python exception based on a Windows error code, while also optionally associating it with a filename.
Letâs break that down step by step.
Raise a Python Exception: In C extensions, you sometimes need to raise a Python exception to signal that something went wrong. This function does exactly that. Based on a Windows Error Code: The source of the error is a Windows-specific error code (also known as a DWORD).
What Does PyErr_SetExcFromWindowsErrWithFilenameObjects Do? đIn essence, PyErr_SetExcFromWindowsErrWithFilenameObjects sets a Python exception based on a Windows error code. If youâve ever encountered a âFile not foundâ error or the infamous âAccess is deniedâ message on Windows, this function allows Python to capture those errors and present them in a way that Python developers are familiar with.
Function Signature and Parameters đBefore diving into the nitty-gritty, letâs look at the functionâs signature:
What Is PyErr_SetExcInfo? đIn simple terms, PyErr_SetExcInfo is like the traffic controller for exceptions in Python. When an exception occurs, Python needs to store information about it somewhereâ a place it can look up later when itâs time to handle or display the error. This function does just that; it updates or sets the current exception info.
Why Use PyErr_SetExcInfo? đImagine you are at a busy airport, and thereâs an air traffic controller who takes note of where each plane is headed.
What Is PyErr_SetFromErrnoWithFilenameObject? đAt its core, PyErr_SetFromErrnoWithFilenameObject is a function in the Python/C API. This function helps you raise an OSError (or one of its subclasses) based on the current value of the C standard libraryâs errno variable. Think of it as a tailor-made error-raising mechanism specifically for system-level errors involving file operations.
How Is It Used? đImagine youâre writing some Python code thatâs supposed to manipulate a fileâsay, open or delete it.
What is PyErr_SetFromErrnoWithFilenameObjects? đImagine working at a bakery. You have a master list of baking errors (letâs call it the âbaking error dictionaryâ) that helps you identify and respond to issues like âOvenTooHotErrorâ or âDoughNotRisingError.â PyErr_SetFromErrnoWithFilenameObjects is akin to adding a note to this baking error dictionary, specifying not just the type of error, but also providing extra context like which oven (or file, in our case) caused the error.
What is PyErr_SetHandledException? đThink of PyErr_SetHandledException as a signal flare for errors in C extensions for Python. When you write Python extensions in C, handling exceptions requires a good understanding of how Python manages errors. PyErr_SetHandledException provides a way to notify Python that an error has occurred and that youâve decided to handle it yourself, often to convert it into another form or perform some cleanup before passing it on.
What is PyErr_SetImportError? đPyErr_SetImportError is a function in Pythonâs C API. Itâs specifically designed to set an ImportError, which signals that an error occurred while attempting to import a module. This function is used internally, but understanding it can give you a clearer picture of how errors are managed in Python.
How is PyErr_SetImportError Used? đTypically, you wonât call PyErr_SetImportError directly in your Python scripts, but itâs helpful to understand how itâs used under the hood.
What is PyErr_SetInterrupt? đImagine youâre at a concert. The main act is performing, and suddenly, an emergency announcement interrupts the show â this is similar to what PyErr_SetInterrupt does to a running Python program. To put it simply, PyErr_SetInterrupt is a function used to simulate a keyboard interrupt (Ctrl+C) in Pythonâs core. This is usually a signal to a running program to stop what itâs doing immediately.
How is PyErr_SetInterrupt Used?
What is PyErr_SetNone? đImagine youâre a librarian (bear with me here). Everythingâs running smoothly until someone asks for a book that you donât have. Instead of staring at them blankly, you need a way to indicate that thereâs an issue. PyErr_SetNone is like putting up a sign that says, âWe donât have that book.â Essentially, it sets a specific error indicator without the need for additional error messages.
How is PyErr_SetNone Used?
What is PyErr_SetString? đLetâs start with the basics. In the simplest terms, PyErr_SetString is a function used within the Python C API to raise exceptions. Think of it as a way for C-based extensions to communicate errors back to the Python interpreter, much like a lighthouse guiding ships safely to shore.
Hereâs the function signature:
void PyErr_SetString(PyObject *type, const char *message); type: This is the type of the exception you wish to raise.
What is PyErr_SyntaxLocation? đImagine youâre at a theater watching a play, and suddenly, a spotlight flashes on a particular actor who has forgotten their lines. That spotlight helps the audience focus on the mistake so that it can be fixed. In the Python world, PyErr_SyntaxLocation functions similarly by pinpointing the exact location in your code where a syntax error has occurred.
Hereâs a formal definition: PyErr_SyntaxLocation is a function in Pythonâs C APIâprimarily used in writing Python interpreters or extensions.
What is PyErr_WarnEx? đThink of PyErr_WarnEx as Pythonâs way of raising a warning flag. Imagine youâre sailing a ship, and you spot something unusual on the horizon. You raise a yellow flag to alert everyone on board: âHey, somethingâs off, but itâs not catastrophicâjust be aware!â This is essentially what PyErr_WarnEx does in your Python code.
In slightly more technical terms, PyErr_WarnEx is a C API function in Pythonâs C interface that allows you to issue warnings directly from C extensions.
What is PyErr_WarnExplicit? đThink of PyErr_WarnExplicit as the backstage manager in a theaterâyou see the actors (warnings) on stage, but thereâs someone behind the scenes making sure everything runs smoothly. In the realm of Python, PyErr_WarnExplicit is a C API function that explicitly raises a warning, giving you fine-tuned control over how and where the warning is issued.
How is it Used? đAlright, letâs get into the nuts and bolts. If youâre knee-deep in a C extension module and need to toss a warning, PyErr_WarnExplicit is your go-to.
What is PyErr_WarnExplicitObject? đImagine youâre giving a presentation, and instead of rudely interrupting you, someone discreetly hands you a note warning you about an upcoming mistake. PyErr_WarnExplicitObject is kind of like that polite warning system for Python code. It allows you to generate warnings that can warn developers about certain conditions without stopping the entire program.
Why Use It? đWarnings are particularly useful in scenarios where you need to alert the developer about potential issues that arenât necessarily critical.
What is PyErr_WarnFormat? đIn simple terms, PyErr_WarnFormat is like a polite nudge you might give someone when theyâre about to make a small mistake. Itâs Pythonâs way of politely flagging a warning without crashing your program. Unlike exceptions, which are like red lights forcing you to stop, warnings are cautionary yellow lights suggesting you proceed carefully.
How Does PyErr_WarnFormat Work? đHereâs the deal: Python has a robust mechanism for signaling errors and exceptions, but sometimes you donât want to halt everything.
Whatâs PyEval_AcquireLock? đPyEval_AcquireLock is essentially a function that plays a crucial role in managing the GIL. Think of it as the âhandshake dealâ between the bouncer and a threadâa way to say, âHey, I need some exclusive party time for a while.â When a thread calls PyEval_AcquireLock, itâs requesting the GIL, effectively stopping other threads from moving forward until itâs done with its business.
How Is It Used? đPyEval_AcquireLock isnât something youâll typically use in everyday Python scripting or even basic multi-threading.
What is PyEval_EvalFrameEx? đThink of Python code as a script waiting for an actor to bring it to life. That actor is PyEval_EvalFrameEx. In technical terms, PyEval_EvalFrameEx is the C function responsible for executing Python bytecode. Itâs the beating heart of the CPython interpreter, managing the flow of execution for every function and operation.
How is it Used? đThe average Python developer may never directly interact with PyEval_EvalFrameEx, but itâs indispensable for those diving into Python internals or working on extending the interpreter.
What is PyEval_GetFrame? đThink of PyEval_GetFrame as a backstage pass to Pythonâs internal mechanisms. In straightforward terms, PyEval_GetFrame is a function that returns the current thread stateâs frame object. The frame object represents the execution context of the currently running code.
To illustrate, imagine youâre watching a play. The frame object is like peeking behind the curtain to see the actorsâ scripts, their current lines, and their positions on stage. Itâs the behind-the-scenes look at what part of the code is running and all the variables interacting at that moment.
What is PyEval_MergeCompilerFlags? đImagine youâre driving a car. The car itself is Python, the roads are the code, and the signals and signs are compiler flags that direct how the journey (or code execution) should unfold. PyEval_MergeCompilerFlags is akin to a function that merges these signals to ensure youâre heading in the right direction smoothly.
To put it technically, PyEval_MergeCompilerFlags merges the compiler flags in effect for a given code block with those provided by the user.
What is PyEval_ReleaseLock? đImagine youâve got a big, bustling bakery. Thereâs only one oven, so while multiple chefs can be mixing dough and baking cookies, only one chef can use the oven at any one time. In the world of Python, this oven is known as the Global Interpreter Lock (GIL). The GIL is a mutex (sort of like a padlock) that protects access to Python objects, preventing multiple native threads from executing Python bytecodes at once.
What is PyEval_SetTrace? đPyEval_SetTrace is a function in Pythonâs C API (Application Programming Interface) that lets you set a âtrace function.â A trace function is a special kind of function that gets called at various points during the execution of Python codeâlike when a function is called, when a function returns, or when a line of code is executed. Think of it as a security camera thatâs recording every step and move inside your Python script.
What is PyEval_ThreadsInitialized? đTo put it simply, PyEval_ThreadsInitialized is a function in Pythonâs C API that checks whether the Python interpreterâs thread support is initialized. Threads allow you to run multiple chunks of code in seeming parallel, which can boost performance and responsiveness in your programs. However, managing threads can be as tricky as juggling flaming torches.
Think of PyEval_ThreadsInitialized as a stage manager who ensures everything is set before allowing the actors (your threads) to perform on stage.
What is PyException_GetArgs? đThink of PyException_GetArgs as a detective that helps you extract clues from exceptions in Python. When an error occurs, Python creates an âexception objectâ that contains valuable information about what went wrong. PyException_GetArgs retrieves the arguments that were passed to this exception object, enabling you to dissect and handle errors more effectively.
How Itâs Used: A Practical Example đImagine youâve got a shiny new piece of code, but somethingâs off.