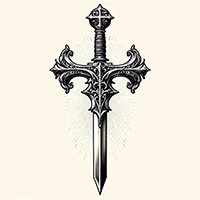
WE SHALL NEVER SURRENDER
Understanding PyDateTime_TIME_GET_HOUR đAt its core, PyDateTime_TIME_GET_HOUR is a macro that extracts the hour component from a time object in Python. This might sound a bit technical, but letâs paint a clearer picture.
Imagine your time object as a delicious sandwich. Within this sandwich, you have different componentsâminutes, seconds, and, most importantly, hours. PyDateTime_TIME_GET_HOUR is like a hungry squirrel that specifically nibbles away at the hour part of your sandwich, ignoring the rest.
What is PyDateTime_TimeZone_UTC? đImagine coordinating a global online meeting; you need everyone to be on the same page about the timing, regardless of their geographical location. This need for a universal standard is where UTC (Coordinated Universal Time) comes into play, functioning somewhat like the Earthâs default clock. PyDateTime_TimeZone_UTC is Pythonâs way of providing programmers with the ability to work with this universal time standard seamlessly.
In essence, PyDateTime_TimeZone_UTC is a built-in object in the Python datetime module that represents the UTC time zone.
What is PyDescr_NewClassMethod? đPicture Python as a bustling kitchen. Each object, class, and method are like chefs, utensils, and ingredients required to whip up a dish. Now, thereâs a special kind of âchefâ in this kitchen known as a class method. This chef (class method) is different because it works directly with the recipe book (class) rather than specific dishes (instances). Enter PyDescr_NewClassMethod: itâs a function that helps create these special class methods.
What is PyDescr_NewGetSet? đIn laymanâs terms, PyDescr_NewGetSet is like the invisible butler of Pythonâs descriptor protocol. Its primary role is to create a new descriptor object that manages the attributes of your class through getter and setter methods. It helps define how attributes of your objects are accessed and modified, ensuring everything stays neatly wrapped up with a little bow on top.
How is it Used? đImagine you have a class with private attributes, but you still want controlled access to these attributes.
What is PyDict_Check? đImagine youâre at a party, and your job is to check if the people coming in are on the guest list. In the world of Pythonâs C API, PyDict_Check is that bouncer â it checks if the object youâre passing through the door is a dictionary.
More formally, PyDict_Check is a macro provided by the Python C API that determines whether a given Python object is a dictionary (dict).
What is PyDict_CheckExact? đSimply put, PyDict_CheckExact is a function in Pythonâs C API that checks whether a given object is an exact dictionary type (dict). Itâs part of the CPython implementation, which is the most widely used implementation of the Python language.
How is PyDict_CheckExact Used? đImagine youâre the gatekeeper at a fancy club. Your job is not just to check if peopleâs IDs say theyâre over 21 but specifically if theyâre carrying the highest level of membership card.
What is PyDict_Clear? đPyDict_Clear is a powerful function in Pythonâs C-API, designed to empty a dictionary, removing all key-value pairs. Think of it as a reset button. Imagine you have a whiteboard covered with scribbles and charts. Using PyDict_Clear is like erasing everything at once, getting it ready for fresh ideas.
Syntax đvoid PyDict_Clear(PyObject *p) In splendid Pythonic glory, this function takes a single argument: a pointer to a dictionary object (PyObject *p).
What is PyDict_Contains? đLetâs start with the basics. PyDict_Contains is a function available in Pythonâs C API. In simple terms, it checks whether a specific key exists in a given dictionary. Think of a Python dictionary as a specialized container, like a filing cabinet, where each file (key) has its own specific piece of information (value). If you want to know whether a particular file exists in your cabinet, PyDict_Contains is the function that does the job.
What is PyDict_Copy? đPyDict_Copy is a function from Pythonâs C API that creates a shallow copy of a dictionary. This might sound a bit like technical jargon, so letâs break it down. Imagine you have a recipe book (your dictionary) full of delicious recipes (your key-value pairs). If you wanted a duplicate of this book so you could experiment with new recipes without altering the original, PyDict_Copy is the copying machine youâd use.
What is PyDict_GetItem? đImagine your Python dictionary as a sophisticated warehouse where each item is labeled with a unique barcode (the keys). When you need to fetch an item, you hand over the barcode to the warehouseâs robotic system, which then zooms through the aisles and brings the item back to you. PyDict_GetItem is basically that robotic system.
In technical terms, PyDict_GetItem is a C function within the Python C API that retrieves an item from a dictionary given a specific key.
What is PyDict_GetItemString? đPyDict_GetItemString is part of the Python/C API, a set of functions that allows C code to interact with Python objects. Specifically, PyDict_GetItemString retrieves a value from a Python dictionary (dict) using a C string as the key.
How to Use PyDict_GetItemString đHereâs a concise guide:
Include the Header:
To use PyDict_GetItemString, ensure you include Pythonâs main header file:
#include <Python.h> Retrieve an Item:
Use the function as follows:
What is PyDict_Items? đAt its core, PyDict_Items is a C-API function used within the Python interpreter to access all key-value pairs in a dictionary. Think of it as a backstage pass to every detail in your Python dictionary.
How Does It Work? đDictionaries in Python are like real-life dictionaries. Instead of words and their definitions, they contain key-value pairs. If your Python dictionary was a magical chest, PyDict_Items would be the spell that reveals all the treasures inside.
What is PyDict_Merge? đImagine youâve got two workshops filled with tools (dictionaries). Wouldnât it be convenient if there was a magical assistant that could seamlessly combine all the tools into one super workshop without losing anything important? Thatâs precisely what PyDict_Merge does, but with Python dictionaries.
In essence, PyDict_Merge is a C function in Pythonâs C API that efficiently merges two Python dictionaries. Itâs the behind-the-scenes magician that handles the merging process without you breaking a sweat.
What is PyDict_MergeFromSeq2? đPyDict_MergeFromSeq2 is a function used within the C-API of Python, primarily involved with dictionary operations. Imagine you have two pieces of a jigsaw puzzle, each comprising multiple smaller pieces. Youâd need to combine these two chunks to form a single coherent picture. PyDict_MergeFromSeq2 is the function performing this merging task, but instead of jigsaw pieces, itâs working with dictionaries and sequences.
What Does It Do? đIn essence, PyDict_MergeFromSeq2 takes a sequence of key-value pairs and merges them into an existing dictionary.
What Does PyDict_SetItemString Do? đAt its core, PyDict_SetItemString inserts an item into a Python dictionary. Think of it like slipping a new sheet of paper into an old, leather-bound dictionary; this paper has one word written on it (our key) and a definition explaining that word (our value). The PyDict_SetItemString function updates or adds this key-value pair to the dictionary.
Prototype:
int PyDict_SetItemString(PyObject *p, const char *key, PyObject *val); The parameters here are pretty straightforward:
What is PyDict_Size? đIn the Python C API, PyDict_Size is a function that returns the number of items in a dictionary. Think of it as the dictionaryâs personal trainer giving you an exact count of all the elements it holds.
The Python Dictionary đBefore we cast the PyDict_Size spell, letâs get a good grasp of what a Python dictionary is. A dictionary in Python is essentially a collection of key-value pairs.
What is PyDict_Type? đThink of PyDict_Type as the DNA of Python dictionaries. In Python, everything is an object, and every object is an instance of a type. For dictionaries, that type is PyDict_Type. Essentially, Python dictionaries rely on this type definition to structure their behavior and properties.
How is PyDict_Type Used? đTo put it simply, PyDict_Type is used internally by Python to manage dictionary objects. When you create a dictionary in Python (using {} or dict()), Python uses PyDict_Type to handle the object.
What is PyDict_Unwatch? đSimply put, PyDict_Unwatch is a function in Pythonâs C API that stops the âwatchingâ mechanism on a Python dictionary. If we imagine Python dictionaries as living in a bustling metropolis, PyDict_Unwatch would be akin to taking down the surveillance cameras that keep an eye on the dictionaryâs activities.
How is it Used? đFirst things first: PyDict_Unwatch is not something youâll typically use in your average Python script. Itâs a part of the CPython internals, primarily utilized by developers diving into Pythonâs core or extending Python with C/C++ code.
What is PyDict_Update? đLetâs start with the basics. PyDict_Update is a function in Pythonâs C API, which allows you to update a dictionary (yes, the dict objects we know and love) with elements from another dict or an iterable of key-value pairs. Think of it as transferring the contents from one shopping cart to anotherâyouâre consolidating everything you need in one place.
Usage: When and Why? đImagine you have two dictionaries:
What is PyErr_BadArgument? đIn simple terms, PyErr_BadArgument is a function used in Pythonâs C API to signal that a C function was called with an incorrect argument. Think of it as waving a red flag when something goes wrong with the arguments passed to a function.
Imagine youâre a gatekeeper, and your job is to check tickets at an event. If someone hands you a ticket to the wrong event, youâd raise your hand to alert everyone that this person doesnât belong here.
What is PyErr_CheckSignals? đImagine youâre at a concert. The band is playing, and the crowd is enthusiastic. Among the noise, you get a phone call. You want to listen to the music but also check if the call is important. PyErr_CheckSignals is Pythonâs way of pausing the concert for a split second to see if there are any important calls (signals) that Python needs to handle right away.
How is PyErr_CheckSignals Used?
What is PyErr_Clear? đThe PyErr_Clear function is part of the Python C API, a set of functions that allow for interaction between Python and C/C++ code. Specifically, PyErr_Clear() is used to clear the current error indicator in the Python interpreter.
Consider the Python interpreter as a well-managed office building. When an error occurs, itâs like a warning light flashing in the buildingâs control room. PyErr_Clear is the action of turning off that warning light, signifying that the error has been acknowledged and dismissed.
What is PyErr_DisplayException? đImagine Python running your code as a determined messenger delivering letters (each line of code) through a windy countryside. Occasionally, this messenger (your code) stumbles upon a particularly nasty potholeâa bug or an error. Think of PyErr_DisplayException as the town crier who loudly announces any significant mishaps encountered by our trusty messenger, so everyone (developers and debugging tools) is aware of what went wrong.
In technical terms, PyErr_DisplayException is a function in Pythonâs C-API that is responsible for displaying information about an exception that has occurred.
What is PyErr_Fetch? đIn the simplest terms, PyErr_Fetch is a function in Pythonâs C API that retrieves the current active exception. Think about it as a detective fetching clues about what went wrong. It captures the type, value, and traceback of the exception.
Hereâs the prototype:
void PyErr_Fetch(PyObject **ptype, PyObject **pvalue, PyObject **ptraceback); ptype: Pointer to the exception type. pvalue: Pointer to the exception instance (the âvalueâ of the exception). ptraceback: Pointer to the traceback object (provides stack information).
What is PyErr_Format? đAt its core, PyErr_Format is a function in Pythonâs C API that allows you to set a Python exception with a formatted error message. Think of it as Pythonâs way of saying, âIâm encountering a problem here, and let me tell you exactly why in a human-readable way.â
How to Use PyErr_Format? đThe syntax for PyErr_Format might look intimidating if youâre new, but itâs quite straightforward. Hereâs the prototype:
What is PyErr_GetExcInfo? đPyErr_GetExcInfo is a function in the Python C API, part of the underlying machinery that powers Pythonâs exception handling. Think of it as the behind-the-scenes director in a theater production, coordinating the actors (your code) and managing unexpected mishaps (errors).
In simpler terms, PyErr_GetExcInfo retrieves detailed information about the current active exception. This includes the exception type, value (or message), and tracebackâall essential elements for diagnosing and debugging errors.
What is PyErr_GetHandledException? đPyErr_GetHandledException is a C-level API method provided by Pythonâs C API. This function is typically not encountered by everyday Python programmers who stick to high-level code. Instead, itâs a tool for those digging deeper into Pythonâs internals, possibly writing extension modules or embedding Python in larger C applications.
In simple terms, PyErr_GetHandledException helps you retrieve the exception that has been caught by the most recent PyErr_Check* or PyErr_Occurred function calls.
What is PyErr_GetRaisedException? đIn Python, the C API provides several functions for managing errors and exceptions. PyErr_GetRaisedException is one such function designed to retrieve the current active exception. Think of it like a fish net you cast into the turbulent waters of your code to catch the elusive error fish swimming by.
How to Use PyErr_GetRaisedException đUsing PyErr_GetRaisedException is quite straightforward, but it does require some understanding of Pythonâs C API, which can be a bit more technical than your average Python script.
What is PyErr_GivenExceptionMatches? đImagine youâre baking cookies, and youâve got a specific recipe in mind for chocolate chip cookies. You go through your pantry, identifying ingredients for this particular cookie recipe. Similarly, PyErr_GivenExceptionMatches is a function in Pythonâs C API that sifts through raised exceptions to check if they match a particular type.
The Basics đAt its core, PyErr_GivenExceptionMatches checks if a given exception is a specific class or a subclass.
What Does PyErr_NewExceptionWithDoc Do? đImagine youâre a director of a play, and you need a specific type of character - letâs call them the protagonist - who has unique traits and a detailed backstory. PyErr_NewExceptionWithDoc is akin to casting this protagonist with all their quirks. In technical terms, it allows you to create a new custom exception in Python and document it at the same time.
How Is It Used? đCreating a custom exception in Python can be critical when you need to signal an error specific to your application or library.